这不是递归了吗我需要非递归
时间: 2024-09-27 21:08:32 浏览: 22
抱歉,我理解了您的需求。如果需要非递归的方式实现深度优先搜索(DFS),那么我们可以使用栈(Stack)数据结构来模拟递归的过程。以下是一个C语言的非递归DFS核心代码示例:
```c
#include <stdio.h>
#include <stdlib.h>
#include <stdbool.h> // 引入布尔类型
typedef struct Node {
int value;
struct Node* next; // 邻居节点指针
} Node;
typedef struct Graph {
int num_nodes;
Node** adj_list; // 邻接表数组
} Graph;
bool visit(int node, Graph* graph, Stack* stack, bool visited[]) {
visited[node] = true;
printf("%d ", node); // 输出节点值
Node* current = graph->adj_list[node];
while (current != NULL) {
if (!visited[current->value]) {
stack_push(stack, current->value); // 将未访问的邻接节点压入栈
}
current = current->next;
}
return !stack_is_empty(stack); // 如果栈不空,说明还有未访问的节点
}
void non_recursive_dfs(Graph* graph, int start) {
int num_nodes = graph->num_nodes;
bool* visited = (bool*)malloc(num_nodes * sizeof(bool)); // 初始化访问标记
Stack* stack = create_stack(); // 初始化堆栈
visited[start] = true;
stack_push(stack, start);
while (!stack_is_empty(stack)) {
int node = stack_pop(stack);
if (!visit(node, graph, stack, visited)) break; // 当栈空或者所有可达节点都访问过时跳出循环
}
free(visited);
destroy_stack(stack);
}
int main() {
Graph g;
... // 初始化邻接表...
non_recursive_dfs(&g, 0); // 从节点0开始
return 0;
}
```
在这个代码里,我们首先初始化一个栈和访问标记数组,然后将起始节点放入栈中。在while循环中,我们不断地弹出栈顶节点并访问它的邻居,直到栈为空或所有可达节点都被访问。这样就实现了非递归的DFS。
阅读全文
相关推荐
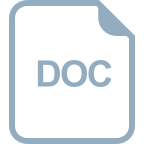
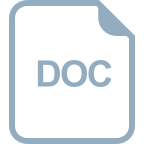
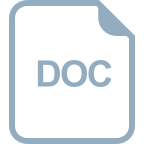
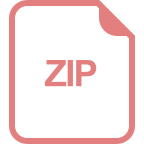
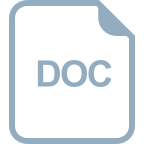
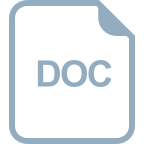
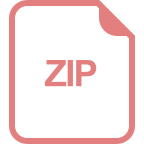
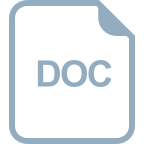
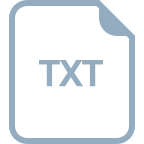
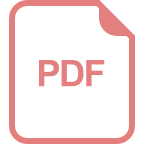
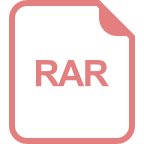
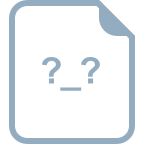
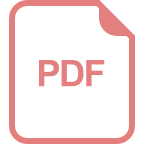
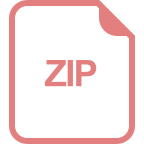