用 C 语言解二元一次方程
时间: 2024-09-23 08:10:20 浏览: 90
在C语言中,解二元一次方程通常涉及到线性代数的基本知识,尤其是求解线性系统的操作。一个典型的二元一次方程组形式如下:
a * x + b * y = c
d * x + e * y = f
其中x和y是未知数,a、b、c、d、e和f是常数。
下面是一个简单的函数示例,用于解决此类方程组。我们将使用高斯消元法(Gaussian Elimination),这是最基础的线性方程组求解方法之一:
```c
#include <stdio.h>
void solveLinearEquation(double a, double b, double c, double d, double e, double f) {
// 检查行列式是否非零,表示方程有唯一解
if (a * e - b * d != 0) {
// 计算逆矩阵的元素
double det = a * e - b * d;
double invDet = 1 / det;
double x = (c * e - b * f) * invDet; // x的值
double y = (a * f - c * d) * invDet; // y的值
printf("The solution is: x = %.2lf, y = %.2lf\n", x, y);
} else if (a * e == b * d && a != 0) { // 方程无解
printf("No unique solution.\n");
} else { // 行列式为0,无穷多解
printf("Infinite solutions.\n");
}
}
int main() {
double a, b, c, d, e, f;
printf("Enter coefficients for the system of equations:\na*x + b*y = c\nd*x + e*y = f\n");
scanf("%lf %lf %lf %lf %lf %lf", &a, &b, &c, &d, &e, &f);
solveLinearEquation(a, b, c, d, e, f);
return 0;
}
```
阅读全文
相关推荐
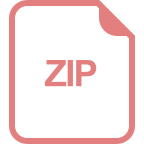
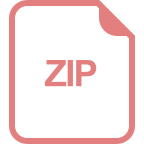
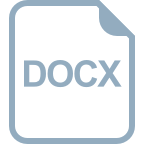
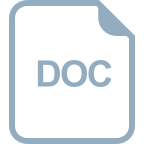



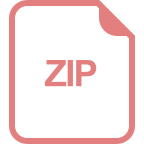
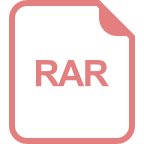
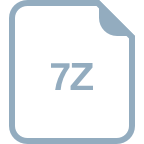
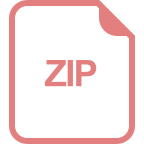
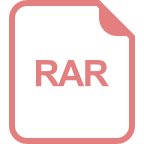



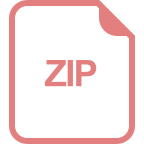
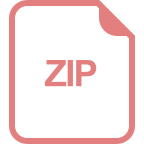
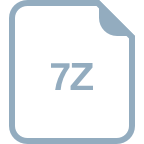
