c++ 字符串查找子串
时间: 2023-11-24 13:51:48 浏览: 170
以下是C++中字符串查找子串的例子:
```cpp
#include <iostream>
#include <string>
using namespace std;
int main() {
string a = "abcdefghigklmn";
string b = "def";
string c = "123";
string::size_type idx;
idx = a.find(b); // 在a中查找b
if (idx == string::npos) // 不存在
cout << "not found\n";
else // 存在
cout << "found\n";
return 0;
}
```
该程序中,使用了string类的find()函数来查找子串。如果子串存在,则返回子串在原字符串中的起始位置;如果不存在,则返回string::npos。在该程序中,我们定义了三个字符串a、b、c,其中a为原字符串,b为要查找的子串,c为一个不存在于a中的字符串。程序首先在a中查找b,如果存在,则输出“found”;如果不存在,则输出“not found”。
相关问题
c++字符串查找子串
在C++中,你可以使用`find`函数来查找子串。以下是一个简单的示例代码:
```cpp
#include <iostream>
#include <string>
int main() {
std::string str = "Hello, World!";
std::string subStr = "World";
size_t found = str.find(subStr);
if (found != std::string::npos
c++字符串查找子串函数
在C++中,你可以使用`find()`函数来进行字符串的子串查找操作。`find()`函数是`std::string`类的成员函数,用于在一个字符串中查找另一个子串,并返回第一次出现的位置。
以下是使用`find()`函数进行子串查找的示例代码:
```cpp
#include <iostream>
#include <string>
int main() {
std::string str = "Hello, World!";
std::string target = "World";
size_t found = str.find(target);
if (found != std::string::npos) {
std::cout << "Substring found at index " << found << std::endl;
} else {
std::cout << "Substring not found" << std::endl;
}
return 0;
}
```
输出结果为:
```
Substring found at index 7
```
这表明目标子串"World"在原字符串"Hello, World!"中的索引位置为7。
你还可以使用`rfind()`函数来从字符串的末尾开始查找子串。此外,还有其他一些成员函数,如`find_first_of()`、`find_last_of()`、`find_first_not_of()`等,可以根据特定需求进行子串查找操作。具体使用哪个函数取决于你的需求。
阅读全文
相关推荐
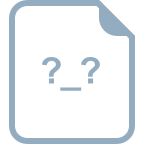

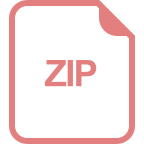
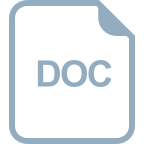








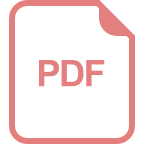
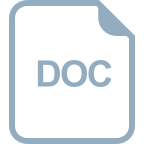
