c语言输入极径和极角,求俩个极坐标距离
时间: 2024-10-10 20:17:39 浏览: 52
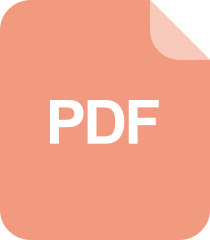
C语言实现直角坐标转换为极坐标的方法
在C语言中,计算两个极坐标的距离通常涉及以下步骤:
1. **输入极坐标**:
- 用户需要分别输入第一个点的极径(r1)和极角(θ1,以弧度表示),例如通过`scanf`函数读取。
- 同样,用户也需要输入第二个点的极径(r2)和极角(θ2)。
2. **转换到直角坐标**:
- 极坐标到直角坐标的转换公式是 \( x = r \cos(\theta) \) 和 \( y = r \sin(\theta) \),这里 \( r \) 是半径,\( θ \) 是角度。
- 对于每个点,先计算对应的直角坐标 (x1, y1) 和 (x2, y2)。
3. **计算两点间的欧几里得距离**:
- 欧氏距离公式是 \( d = \sqrt{(x2-x1)^2 + (y2-y1)^2} \)。
- 使用这个公式,代入直角坐标的差值来得到两点之间的距离。
4. **输出结果**:
- 最后,将计算出的距离输出给用户。
下面是一个简单的伪代码示例:
```c
#include <stdio.h>
#include <math.h>
double polar_to_cartesian(double r, double theta) {
return r * cos(theta);
}
double distance_in_cartesian(double x1, double y1, double x2, double y2) {
return sqrt((x2 - x1) * (x2 - x1) + (y2 - y1) * (y2 - y1));
}
int main() {
double r1, theta1, r2, theta2;
printf("请输入第一个点的极径和极角(单位:弧度): ");
scanf("%lf %lf", &r1, &theta1);
printf("请输入第二个点的极径和极角(单位:弧度): ");
scanf("%lf %lf", &r2, &theta2);
double x1 = polar_to_cartesian(r1, theta1);
double y1 = polar_to_cartesian(r1, theta1 + M_PI / 2); // 因为角度范围通常是0到π,所以加 π/2 转换到笛卡尔坐标系
double x2 = polar_to_cartesian(r2, theta2);
double y2 = polar_to_cartesian(r2, theta2 + M_PI / 2);
double distance = distance_in_cartesian(x1, y1, x2, y2);
printf("两点之间的距离是: %.2f\n", distance);
return 0;
}
```
阅读全文
相关推荐
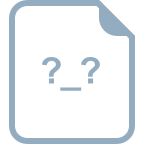
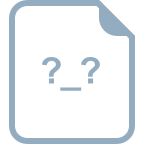
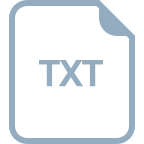
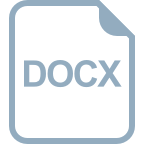
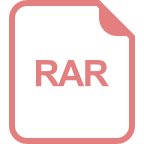
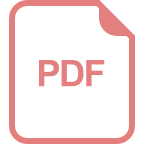
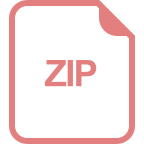
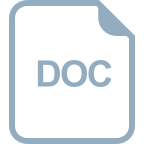
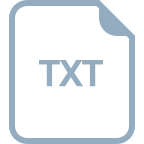
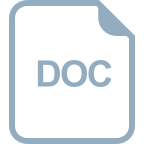
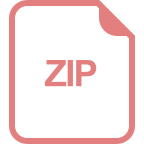