用数据结构知识写出栈的初始化入栈出栈取栈顶元素并可实现的代码
时间: 2024-09-30 15:16:32 浏览: 19
栈是一种特殊的数据结构,它遵循"后进先出"(LIFO, Last In First Out)的原则。在Python中,我们可以使用列表(List)来实现一个简单的栈。下面是基本操作的代码示例:
```python
class Stack:
def __init__(self):
self.stack = []
# 初始化(构造函数)
def init_stack(self):
print("Stack initialized.")
# 入栈(push)
def push(self, item):
self.stack.append(item)
print(f"{item} pushed to the stack.")
# 出栈(pop)
def pop(self):
if not self.is_empty():
return self.stack.pop()
else:
return "Stack is empty."
# 取栈顶元素(peek)
def peek(self):
if not self.is_empty():
return self.stack[-1]
else:
return "Stack is empty."
# 判断栈是否为空
def is_empty(self):
return len(self.stack) == 0
# 打印当前栈顶元素
def show_top(self):
if not self.is_empty():
print(f"The top element in the stack is {self.peek()}.")
else:
print("Stack is empty.")
# 使用示例
stack = Stack()
stack.init_stack()
stack.push(1)
stack.push("two")
stack.show_top() # 输出 "The top element in the stack is two."
print(stack.pop()) # 输出 "two"
stack.show_top()
相关推荐
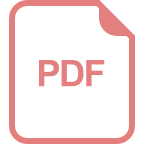
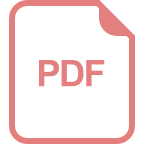
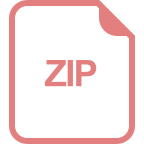














