编写一个能创建单链表并实现其基本操作的程序
时间: 2024-10-12 16:08:44 浏览: 22
创建一个单链表并实现基础操作,通常涉及以下几个步骤:
首先,我们需要定义一个节点类`ListNode`,它包含一个数据元素和指向下一个节点的引用。
```python
class ListNode:
def __init__(self, data=0):
self.data = data
self.next = None
```
然后,我们可以定义链表类`LinkedList`,它包含创建链表、添加节点、删除节点以及打印链表等方法。
```python
class LinkedList:
def __init__(self):
self.head = None
# 添加节点到链表尾部
def append(self, data):
if not self.head:
self.head = ListNode(data)
else:
current = self.head
while current.next:
current = current.next
current.next = ListNode(data)
# 删除指定位置的节点
def delete_node(self, position):
if not self.head or position <= 0:
return
current = self.head
for _ in range(position - 1):
if not current.next:
break
current = current.next
if current and current.next:
current.next = current.next.next
# 打印链表
def print_list(self):
elements = []
current = self.head
while current:
elements.append(current.data)
current = current.next
print("->".join(map(str, elements)))
# 示例
linked_list = LinkedList()
linked_list.append(1)
linked_list.append(2)
linked_list.append(3)
linked_list.print_list() # 输出: 1 -> 2 -> 3
linked_list.delete_node(1) # 删除第二个节点
linked_list.print_list()
阅读全文
相关推荐
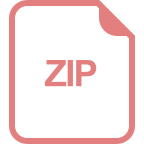
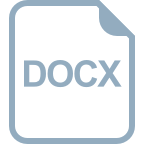
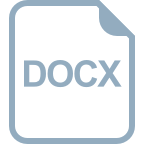















