迷宫求解。运用do语句C语言栈。求路径最小值
时间: 2024-10-21 12:04:22 浏览: 12
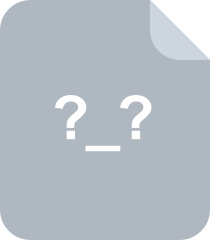
迷宫问题的最短路径C语言实现(栈实现)
迷宫求解是一个常见的计算机科学问题,常常涉及到图论和搜索算法。在C语言中,可以使用递归和栈来解决这个问题。特别是当你提到do-while循环和栈,通常会采用深度优先搜索(Depth First Search, DFS)的方式,因为DFS可以用栈来模拟搜索过程。
下面是一个简化的示例,展示了如何使用do-while循环和栈来找到迷宫中的最短路径,并假设路径长度由步数表示:
```c
#include <stdio.h>
#include <stdlib.h>
#define ROWS 5 // 迷宫的行数
#define COLS 5 // 迷宫的列数
typedef enum { WALL, PATH } CellType;
// 模拟迷宫的二维数组
CellType maze[ROWS][COLS];
// 栈结构体
struct Stack {
int row, col;
};
void dfsExplore(int row, int col, struct Stack* stack, int pathLength) {
if (maze[row][col] == PATH && (row == ROWS - 1 && col == COLS - 1)) { // 到达终点
printf("Path found with length %d: (%d,%d)\n", pathLength, row, col);
}
stack->row = row; stack->col = col; // 入栈
pathLength++; // 更新路径长度
do {
// 检查相邻单元格
int newCol = col + 1, newRow = row;
if (newRow < ROWS && maze[newRow][newCol] != WALL) dfsExplore(newRow, newCol, stack, pathLength); // 右移
newCol--;
if (newCol >= 0 && maze[row][newCol] != WALL) dfsExplore(row, newCol, stack, pathLength); // 左移
newRow = row - 1;
if (newRow >= 0 && maze[newRow][col] != WALL) dfsExplore(newRow, col, stack, pathLength); // 上移
newCol = col - 1;
if (newCol >= 0 && maze[row][newCol] != WALL) dfsExplore(row, newCol, stack, pathLength); // 下移
// 出栈并继续下一个方向
if (!stackEmpty(stack)) {
stack.pop();
}
} while (!stackEmpty(stack)); // 当栈非空时,不断出栈并尝试其他方向
}
int main() {
// 初始化迷宫...
// ...
struct Stack startStack = {0, 0}; // 设定起点
dfsExplore(0, 0, &startStack, 0); // 开始搜索
return 0;
}
```
这个程序首先初始化迷宫,然后从起点开始,通过栈来探索所有可能的路径,直到找到终点。注意这只是一个基本的演示,实际应用中可能需要处理边界条件、回溯等细节。
阅读全文
相关推荐
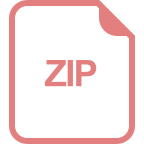
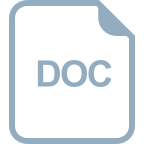
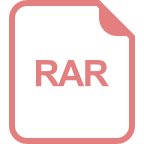
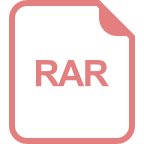
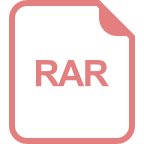
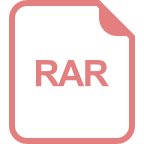
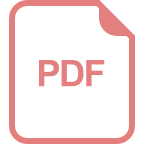
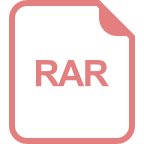
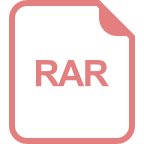
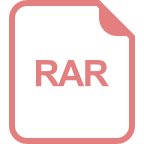
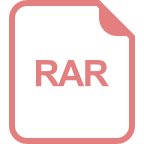
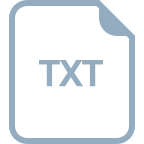
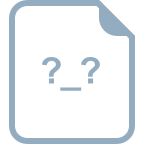
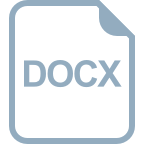