python使用gui做幸运转盘
时间: 2024-11-21 07:14:54 浏览: 8
在Python中使用GUI库来做幸运转盘游戏,最常见的是结合Tkinter库(它是Python的标准GUI工具包)。以下是一个简单的步骤:
1. 导入必要的库:首先导入`tkinter`库和随机数生成模块`random`。
```python
import tkinter as tk
import random
```
2. 设计界面:创建一个窗口,并添加一个表示转盘的部分,通常可以使用`Canvas`绘制一个圆形区域,划分成几个扇区代表奖品。
```python
# 创建主窗口
root = tk.Tk()
# 创建canvas画布
canvas = tk.Canvas(root, width=400, height=400)
circle = canvas.create_oval(0, 0, 400, 400) # 圆形轮廓
```
3. 定义扇区:给每个扇区设置标签和对应的奖励,并在canvas上绘制分割线。
```python
sectors = [{'angle_start': 0, 'angle_end': 360 / n, 'text': prize, 'color': 'blue'} for n, prize in enumerate(prizes)] # prizes列表包含所有奖品
for sector in sectors:
start_angle = sector['angle_start']
end_angle = sector['angle_end']
x1, y1 = canvas.winfo_rootx(), canvas.winfo_rooty() + 200 # 中心点坐标
x2, y2 = x1 + 400, y1
canvas.create_arc(x1, y1, x2, y2, start=start_angle, extent=end_angle, fill=sector['color'])
text_pos = (x1 + (x2 - x1) * sector['angle_start'] // 360, y1 + (y2 - y1) * (90 - sector['angle_start']) // 360)
canvas.create_text(text_pos[0], text_pos[1], anchor='nw', text=sector['text'])
```
4. 用户交互:添加一个按钮触发转轮旋转,选择随机扇区并显示结果。
```python
def spin_wheel():
selected_sector = random.randint(0, len(sectors) - 1)
canvas.itemconfig(circle, fill=sectors[selected_sector]['color'])
label.config(text=sectors[selected_sector]['text'])
button = tk.Button(root, text="Spin", command=spin_wheel)
label = tk.Label(root)
...
```
5. 运行主循环:最后启动主循环,保持窗口打开直到关闭。
```python
root.mainloop()
```
阅读全文
相关推荐
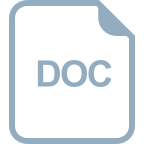
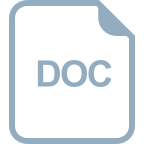
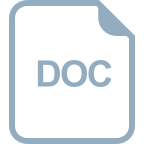
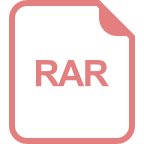
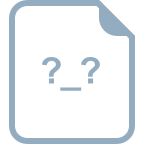
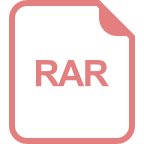
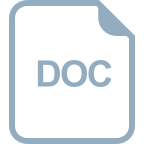
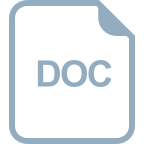
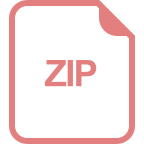
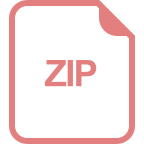
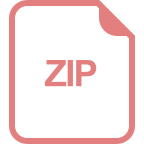
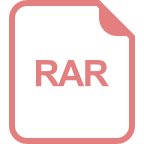
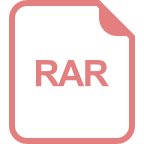
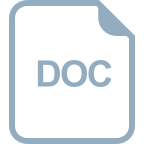
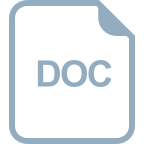
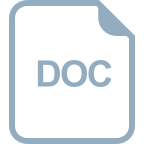
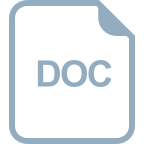
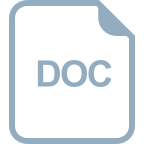
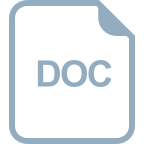
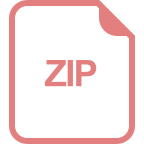