使用指针设计一个fun函数 把主函数输入的三个数,最大的放在a
时间: 2024-09-10 07:29:07 浏览: 49
在C语言中,可以通过指针来设计一个函数`fun`,用于将主函数输入的三个数中最大的数放在变量`a`中。以下是一个简单的示例实现:
```c
#include <stdio.h>
void fun(int *a, int *b, int *c) {
int max = *a;
if (*b > max) {
max = *b;
}
if (*c > max) {
max = *c;
}
*a = max; // 假设我们要将最大的数放在a指向的位置
}
int main() {
int a, b, c, d;
printf("请输入三个整数:");
scanf("%d %d %d", &a, &b, &c);
fun(&a, &b, &c); // 传递变量的地址
printf("最大的数是:%d\n", a); // 假设a是我们想要存放最大数的变量
return 0;
}
```
在这个例子中,`fun`函数接收三个整数的指针作为参数,并在函数内部比较这三个数的大小,将最大值赋给`a`所指向的位置。
相关问题
使用指针设计一个函数fun,把主函数中输入的3个数最大的放a中,最小的放c
你可以使用C++语言中的指针来设计这样一个`fun`函数,这个函数接收三个整数引用作为参数,并通过比较找到最大值和最小值。这里是一个简单的实现:
```cpp
#include <iostream>
// 函数声明,接受指向int的指针作为参数
void findMaxAndMin(int* a, int* b, int* c) {
// 初始化假设a是最大值,c是最小值
int max = *a;
int min = *b;
if (*b > max) {
max = *b;
}
if (*c < min) {
min = *c;
}
// 如果b或c比当前的最大值或最小值还大或小,则更新相应的指针所指向的值
if (*b > max) {
*a = *b; // 更新最大值
} else if (*c < min) {
*a = *c; // 更新最大值,如果c更小
}
if (*c < min) {
*c = min; // 如果c比当前的最小值还小,则更新最小值
}
}
// 主函数
int main() {
int num1, num2, num3;
std::cout << "Enter three numbers: ";
std::cin >> num1 >> num2 >> num3;
int a, b, c;
a = num1;
b = num2;
c = num3;
findMaxAndMin(&a, &b, &c);
std::cout << "The maximum number is: " << a << "\n";
std::cout << "The minimum number is: " << c << "\n";
return 0;
}
```
在这个例子中,`findMaxAndMin`函数接受三个整数的地址,通过对它们的值进行比较,找到并更新`a`和`c`的实际数值。在主函数中,我们首先获取用户输入的三个数,然后传递给`fun`函数处理。
使用c语言 (2)使用指针设计一个函数fun的功能是:把主函数中输入的3个数,最大的放在a中,最小的放在。 中。例如,输入的数为:55 12 34,输出结果应当是:a=55.0,b=34.0,c=12.0
好的,这是一个关于使用C语言指针的问题。下面是一个可能的解答:
```c
#include <stdio.h>
void fun(float *a, float *b, float *c) {
float tmp;
if (*a < *b) {
tmp = *a;
*a = *b;
*b = tmp;
}
if (*a < *c) {
tmp = *a;
*a = *c;
*c = tmp;
}
if (*b < *c) {
tmp = *b;
*b = *c;
*c = tmp;
}
}
int main() {
float a, b, c;
printf("请输入三个数:");
scanf("%f%f%f", &a, &b, &c);
fun(&a, &b, &c);
printf("最大值:%f,中间值:%f,最小值:%f\n", a, b, c);
return 0;
}
```
上述代码定义了一个函数`fun`,该函数使用指针参数,可以将输入的三个数按照大小顺序存储到`a`、`b`、`c`三个变量中。在`main`函数中,首先输入三个数,然后调用`fun`函数,最后输出结果。
相关推荐
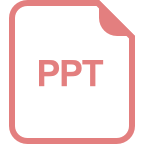
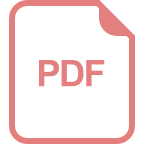
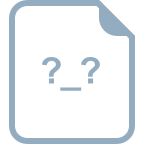

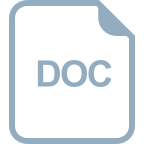












