用 java 语言编写一个计算器类,求实现加、减、乘、除、求平方根、求绝对 值、求倒数 1/x, 方法,并用 JUnit 进行对象类的单元测试。参阅帮助文档。 (说明,设计求除法、求倒数的方法,可在方法中不检测 x 是否为 0
时间: 2024-10-21 15:14:27 浏览: 19
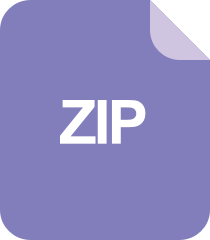
Java计算器 实现最简单的加减乘除、开方、求倒数等

在Java中,我们可以创建一个名为Calculator的类,包含上述功能的方法,并使用JUnit进行单元测试。首先,我们定义Calculator类:
```java
public class Calculator {
// 加法
public double add(double a, double b) {
return a + b;
}
// 减法
public double subtract(double a, double b) {
return a - b;
}
// 乘法
public double multiply(double a, double b) {
return a * b;
}
// 除法,忽略除数为0的情况
@Override
public double divide(double a, double b) {
if (b != 0) {
return a / b;
} else {
throw new IllegalArgumentException("Divisor cannot be zero");
}
}
// 求平方根
public double squareRoot(double num) {
return Math.sqrt(num);
}
// 求绝对值
public double absoluteValue(double num) {
return Math.abs(num);
}
// 求倒数
public double reciprocal(double num) {
if (num != 0) {
return 1.0 / num;
} else {
throw new ArithmeticException("Cannot calculate the reciprocal of zero");
}
}
}
```
接下来,我们编写JUnit测试类来验证Calculator类的功能:
```java
import org.junit.Test;
import static org.junit.Assert.*;
public class CalculatorTest {
private Calculator calculator = new Calculator();
@Test
public void testAdd() {
assertEquals(5, calculator.add(2, 3), 0.0);
}
@Test
public void testSubtract() {
assertEquals(-3, calculator.subtract(5, 8), 0.0);
}
@Test
public void testMultiply() {
assertEquals(10, calculator.multiply(2, 5), 0.0);
}
@Test
public void testDivide() {
assertEquals(2.0, calculator.divide(6, 3), 0.0);
try {
calculator.divide(2, 0);
fail("Expected exception for division by zero");
} catch (IllegalArgumentException e) {
// Expected
}
}
@Test
public void testSquareRoot() {
assertEquals(4.0, calculator.squareRoot(16), 0.0);
}
@Test
public void testAbsoluteValue() {
assertEquals(5, calculator.absoluteValue(-5), 0.0);
}
@Test
public void testReciprocal() {
assertEquals(0.5, calculator.reciprocal(2), 0.0);
try {
calculator.reciprocal(0);
fail("Expected exception for zero denominator");
} catch (ArithmeticException e) {
// Expected
}
}
}
```
阅读全文
相关推荐
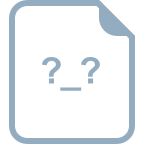
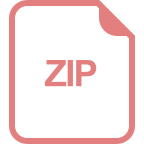


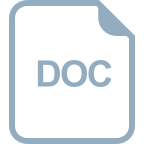
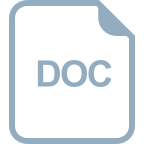
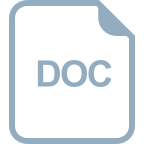
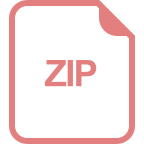
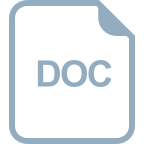
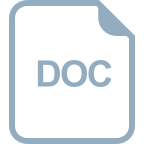
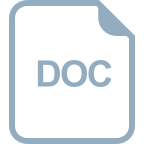
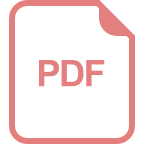
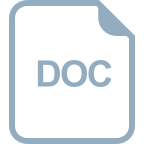
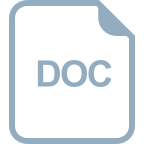
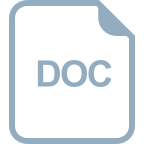
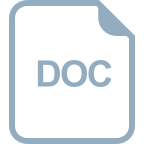
