用c++写一个括号匹配函数
时间: 2024-10-19 18:17:40 浏览: 30
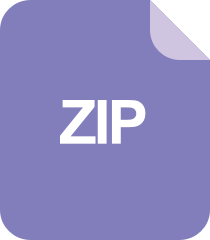
c++括号匹配算法.zip
在C++中,你可以创建一个名为`isParenthesesMatched`的函数,它接受一个字符串参数,代表输入的括号序列。我们可以使用栈来实现括号匹配的逻辑。下面是一个简单的示例代码:
```cpp
#include <stack>
#include <string>
bool isParenthesesMatched(const std::string& expression) {
// 创建一个栈,用于存放左括号
std::stack<char> parenthesesStack;
// 定义一组合法的括号对
const char* validPairs = "()[]{}";
for (char c : expression) {
if (c == '(' || c == '[' || c == '{') { // 打开一个新的括号
parenthesesStack.push(c);
} else if (c == ')' && !parenthesesStack.empty() && parenthesesStack.top() == '(') {
parenthesesStack.pop(); // 匹配成功,移除栈顶的左括号
} else if (c == ']' && !parenthesesStack.empty() && parenthesesStack.top() == '[') {
parenthesesStack.pop();
} else if (c == '}' && !parenthesesStack.empty() && parenthesesStack.top() == '{') {
parenthesesStack.pop();
} else { // 不匹配的括号
return false;
}
}
// 检查栈是否为空,如果为空表示所有的括号都已匹配
return parenthesesStack.empty();
}
// 示例
int main() {
std::string testCases[] = {"()", "({})", "[{()}]", "({[()]})", "({))"};
for (const auto& expr : testCases) {
bool result = isParenthesesMatched(expr);
std::cout << expr << " -> " << (result ? "matched" : "not matched") << '\n';
}
return 0;
}
```
阅读全文
相关推荐
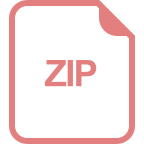
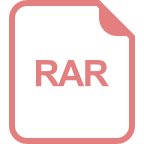

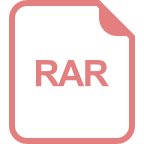
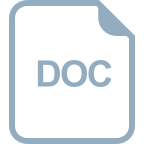
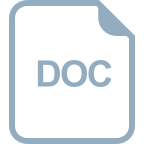
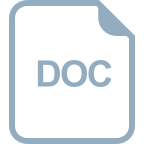








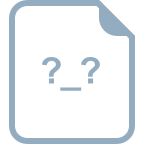
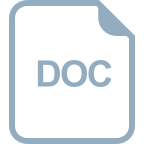
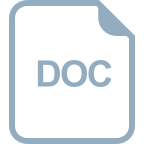