void MainWindow::initDbcTab() { treeModel = new QTableWidget(this); // 设置表格列数和列标题 treeModel->setColumnCount(18); treeModel->setHorizontalHeaderLabels(QStringList()<<"Name"<<"Message"<<"Multiplexing/Group"<<"Startbit"<<"Length[Bit]"<<"Byte Order"<<"Value Type"<<"Initial Value"<<"Factor"<<"Offset"<<"Minimum"<<"Maximum"<<"Unit"<<"Value Table"<<"comment"<<"GensigInactiveValue"<<"GenStartValue"<<"GenSendValue"); // 设置水平表头为可伸缩 treeModel->horizontalHeader()->setSectionResizeMode(QHeaderView::Interactive); // 添加表格到主界面 QVBoxLayout *tableLayout = new QVBoxLayout(this); tableLayout->addWidget(treeModel); }解析
时间: 2023-08-06 18:06:11 浏览: 59
这段代码的目的是在主窗口中初始化一个 QTableWidget,并设置其列数、列标题等属性,并将该表格添加到主界面。
具体解析如下:
1. 首先通过 `treeModel = new QTableWidget(this);` 创建了一个 QTableWidget 对象,并指定了它的父对象为当前的主窗口。这样可以确保在主窗口关闭时,释放相关资源。
2. 通过 `treeModel->setColumnCount(18);` 设置表格的列数为 18。这样表格将拥有 18 列。
3. 使用 `setHorizontalHeaderLabels()` 方法设置表格的水平表头,通过 QStringList 将列标题以列表的形式传入,每个字符串表示一个列的标题。
4. 通过 `treeModel->horizontalHeader()->setSectionResizeMode(QHeaderView::Interactive);` 将水平表头设置为可伸缩,这样用户可以手动调整列宽。
5. 创建一个 QVBoxLayout 对象,并将表格添加到布局中,最后通过 `tableLayout->addWidget(treeModel);` 将布局添加到主界面。
这样,你就完成了在主界面初始化一个包含指定列数和列标题的 QTableWidget 的操作。请确保在主窗口的构造函数或其他适当的地方调用 `initDbcTab()` 方法来执行初始化。
相关问题
if(menunum==1)//微分绘线 { line=new ScanLine(); line->DDALine(&painter, 30, 10, 10, 30); update(); } else if(menunum==2)//中点绘线 { line=new ScanLine(); line->MidLine(&painter ,30, 10, 10, 30); update(); } else if(menunum==3)//brenham { line=new ScanLine(); line->Bresenham(&painter ,30, 10, 10, 30); update(); } } void MainWindow::pop1() { } void MainWindow::pop2() { } void MainWindow::pop3() { }完善槽函数
可以尝试按照以下方式来完善槽函数:
```c++
void MainWindow::pop1() {
menunum = 1;
// 可以在这里添加对应的代码,比如弹出一个窗口让用户输入绘制直线所需的参数等等
}
void MainWindow::pop2() {
menunum = 2;
// 可以在这里添加对应的代码,比如弹出一个窗口让用户输入绘制直线所需的参数等等
}
void MainWindow::pop3() {
menunum = 3;
// 可以在这里添加对应的代码,比如弹出一个窗口让用户输入绘制直线所需的参数等等
}
void MainWindow::paintEvent(QPaintEvent *event) {
if(menunum==1) { // 微分绘线
line = new ScanLine();
line->DDALine(&painter, 30, 10, 10, 30);
update();
} else if(menunum==2) { // 中点绘线
line = new ScanLine();
line->MidLine(&painter, 30, 10, 10, 30);
update();
} else if(menunum==3) { // brenham
line = new ScanLine();
line->Bresenham(&painter, 30, 10, 10, 30);
update();
}
}
```
在 `pop1()`、`pop2()`、`pop3()` 中,我们给 `menunum` 赋上对应的值,这个值将会在 `paintEvent()` 中被使用来判断绘制哪一种直线。
你可以根据需要,添加弹窗等交互功能,让用户输入所需的参数,然后再根据这些参数来调用 `DDALine()`、`MidLine()`、`Bresenham()` 等函数进行绘制。
void MainWindow::paintEvent(QPaintEvent *event) { QPainter painter(this); if(menunum==1)//微分绘线 { line=new ScanLine(); // line->DDALine(&painter); update(); } else if(menunum==2)//中点绘线 { line=new ScanLine(); // line->MidLine(&painter); update(); } else if(menunum==3)//brenham { line=new ScanLine(); // line->Bresenham(&painter); update(); } }新创建一个ScanLine类并在其中构建三种方法绘出直线
根据代码,您可以在ScanLine类中添加三种绘制直线的方法:DDALine、MidLine和Bresenham。下面是一个示例:
```c++
class ScanLine
{
public:
// DDA直线绘制算法
void DDALine(QPainter* painter)
{
// 绘制直线的代码
painter->drawLine(QPointF(0, 0), QPointF(100, 100));
}
// 中点直线绘制算法
void MidLine(QPainter* painter)
{
// 绘制直线的代码
painter->drawLine(QPointF(0, 0), QPointF(100, 100));
}
// Bresenham直线绘制算法
void Bresenham(QPainter* painter)
{
// 绘制直线的代码
painter->drawLine(QPointF(0, 0), QPointF(100, 100));
}
};
```
然后,在主窗口的paintEvent事件中,根据所选的菜单项调用ScanLine类的相应方法即可:
```c++
void MainWindow::paintEvent(QPaintEvent *event)
{
QPainter painter(this);
if (menunum == 1)
{
ScanLine line;
line.DDALine(&painter);
}
else if (menunum == 2)
{
ScanLine line;
line.MidLine(&painter);
}
else if (menunum == 3)
{
ScanLine line;
line.Bresenham(&painter);
}
}
```
当然,您需要根据实际需求修改ScanLine类中的绘制直线的代码。
相关推荐
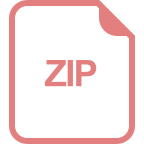
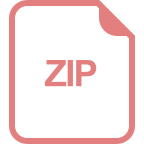
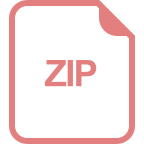












