bert bilstm crf模型代码
时间: 2024-03-11 07:41:50 浏览: 146
BERT-BiLSTM-CRF模型是一种用于命名实体识别(NER)任务的深度学习模型,它结合了BERT预训练模型、双向LSTM和条件随机场(CRF)层。下面是一个简单的BERT-BiLSTM-CRF模型的代码示例:
```python
import torch
import torch.nn as nn
from transformers import BertModel
class BERTBiLSTMCRF(nn.Module):
def __init__(self, num_labels, hidden_size, lstm_hidden_size, dropout_rate):
super(BERTBiLSTMCRF, self).__init__()
self.bert = BertModel.from_pretrained('bert-base-uncased')
self.dropout = nn.Dropout(dropout_rate)
self.lstm = nn.LSTM(hidden_size, lstm_hidden_size, bidirectional=True, batch_first=True)
self.hidden2tag = nn.Linear(lstm_hidden_size * 2, num_labels)
self.crf = CRF(num_labels)
def forward(self, input_ids, attention_mask):
outputs = self.bert(input_ids=input_ids, attention_mask=attention_mask)
sequence_output = outputs[0]
sequence_output = self.dropout(sequence_output)
lstm_output, _ = self.lstm(sequence_output)
logits = self.hidden2tag(lstm_output)
return logits
def loss(self, input_ids, attention_mask, tags):
logits = self.forward(input_ids, attention_mask)
loss = -self.crf(logits, tags)
return loss
def decode(self, input_ids, attention_mask):
logits = self.forward(input_ids, attention_mask)
tags = self.crf.decode(logits)
return tags
```
这段代码使用了PyTorch和Hugging Face的transformers库。模型的构建包括以下几个步骤:
1. 导入所需的库和模块。
2. 定义BERTBiLSTMCRF类,继承自nn.Module。
3. 在类的构造函数中,初始化BERT模型、dropout层、双向LSTM层、线性层和CRF层。
4. 实现forward方法,用于前向传播计算模型输出。
5. 实现loss方法,用于计算模型的损失函数。
6. 实现decode方法,用于解码模型的输出结果。
这只是一个简单的示例代码,实际使用时可能需要根据具体任务进行修改和调整。
阅读全文
相关推荐
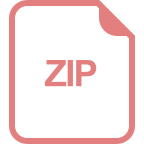
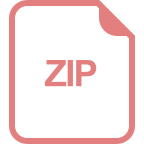
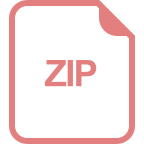




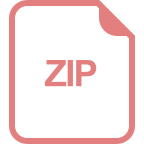







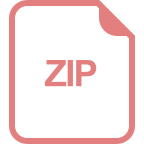
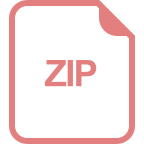
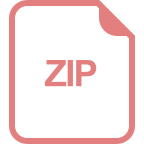
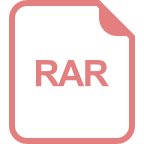