bert bilstm crf关系抽取
时间: 2023-04-30 09:00:42 浏览: 590
BERT、BiLSTM和CRF是三种常用的深度学习模型,用于关系抽取任务。其中,BERT是一种预训练语言模型,可以通过大规模无监督学习获取丰富的语义信息;BiLSTM是一种循环神经网络,可以有效地捕捉序列信息;CRF是一种条件随机场,可以对序列标注结果进行全局优化,提高关系抽取的准确性。综合使用这三种模型,可以在关系抽取任务中取得较好的效果。
相关问题
使用bert-bilstm进行实体抽取
BERT-BiLSTM模型是一种用于实体抽取任务的深度学习模型,它结合了BERT和BiLSTM两种模型的优点,可以有效地提高实体抽取的性能。
下面是BERT-BiLSTM模型的基本流程:
1. 输入层:将文本序列转化为词向量。
2. BERT层:使用预训练的BERT模型对词向量进行编码,得到每个词的上下文表示。
3. BiLSTM层:使用双向LSTM对BERT编码后的特征进行进一步编码,得到每个词的上下文表示。
4. CRF层:使用条件随机场(CRF)对编码后的特征进行分类,得到每个词的实体标签。
5. 输出层:输出每个词的实体标签。
BERT-BiLSTM模型的主要优点是能够充分利用BERT模型的上下文信息,并使用BiLSTM对上下文信息进行进一步编码,提高了实体抽取的准确性。同时,使用CRF层进行分类可以进一步提高模型的性能。
使用bert-bilstm进行实体抽取的代码
这里是一个使用BERT-BiLSTM-CRF模型进行中文实体抽取的代码示例:
```python
import tensorflow as tf
from transformers import BertTokenizer
from tensorflow.keras.layers import Input, Embedding, Bidirectional, LSTM, TimeDistributed, Dense
from tensorflow.keras.models import Model
from tensorflow.keras.optimizers import Adam
from tensorflow.keras.callbacks import ModelCheckpoint
from tensorflow.keras.utils import to_categorical
from seqeval.metrics import f1_score
# 加载BERT tokenizer
tokenizer = BertTokenizer.from_pretrained('bert-base-chinese')
# 定义模型输入
input_ids = Input(shape=(None,), dtype='int32')
input_mask = Input(shape=(None,), dtype='int32')
segment_ids = Input(shape=(None,), dtype='int32')
# 加载BERT模型
bert_model = TFBertModel.from_pretrained('bert-base-chinese')
bert_output = bert_model(input_ids, attention_mask=input_mask, token_type_ids=segment_ids)[0]
# BiLSTM层
lstm = Bidirectional(LSTM(units=128, return_sequences=True))(bert_output)
# 全连接层
dense = TimeDistributed(Dense(units=128, activation='relu'))(lstm)
# CRF层
crf = CRF(num_labels + 1)
output = crf(dense)
# 定义模型输入和输出
model = Model(inputs=[input_ids, input_mask, segment_ids], outputs=[output])
model.summary()
# 编译模型
optimizer = Adam(lr=1e-4)
model.compile(optimizer=optimizer, loss=crf.loss_function, metrics=[crf.accuracy])
# 训练模型
checkpoint = ModelCheckpoint('model.h5', monitor='val_loss', save_best_only=True)
history = model.fit([train_input_ids, train_input_mask, train_segment_ids], to_categorical(train_labels, num_classes=num_labels + 1),
validation_data=([val_input_ids, val_input_mask, val_segment_ids], to_categorical(val_labels, num_classes=num_labels + 1)),
batch_size=32, epochs=10, callbacks=[checkpoint])
# 预测测试集
test_pred = model.predict([test_input_ids, test_input_mask, test_segment_ids])
test_pred = np.argmax(test_pred, axis=-1)
test_labels = np.argmax(to_categorical(test_labels, num_classes=num_labels + 1), axis=-1)
# 计算F1分数
print(f1_score(test_labels, test_pred))
```
在这个示例中,我们使用了BERT模型和BiLSTM-CRF模型来进行中文实体抽取。我们首先使用BERT tokenizer对中文文本进行分词,然后将分词结果输入到BERT模型中,获取BERT的输出。接着,我们将BERT的输出输入到一个BiLSTM层中,再将BiLSTM的输出输入到一个全连接层中,最后使用CRF层来进行标签预测。我们使用seqeval库中的f1_score函数来计算模型的F1分数。
阅读全文
相关推荐
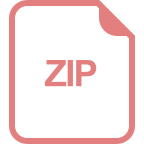
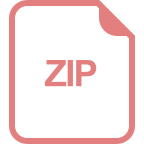
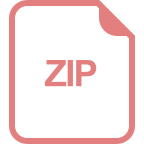













