bilstm-crf实体关系抽取模型输出预测结果的代码
时间: 2024-01-25 09:04:05 浏览: 169
以下是一个简单的 BiLSTM-CRF 实体关系抽取模型的输出预测结果的 Python 代码示例:
```python
import numpy as np
import tensorflow as tf
# 定义标签列表
label_list = ['O', 'B-PER', 'I-PER', 'B-LOC', 'I-LOC', 'B-ORG', 'I-ORG']
# 定义模型参数
hidden_size = 128
num_layers = 2
dropout_rate = 0.5
learning_rate = 0.001
# 定义输入数据占位符
input_ids = tf.placeholder(dtype=tf.int32, shape=[None, None], name="input_ids")
input_mask = tf.placeholder(dtype=tf.int32, shape=[None, None], name="input_mask")
segment_ids = tf.placeholder(dtype=tf.int32, shape=[None, None], name="segment_ids")
# 定义模型
bert_config = modeling.BertConfig.from_json_file("bert_config.json")
model = modeling.BertModel(
config=bert_config,
is_training=False,
input_ids=input_ids,
input_mask=input_mask,
token_type_ids=segment_ids,
use_one_hot_embeddings=False)
output_layer = model.get_sequence_output()
logits = tf.layers.dense(output_layer, len(label_list), name="logits")
crf_params = tf.get_variable("crf_params", shape=[len(label_list), len(label_list)], dtype=tf.float32)
pred_ids, _ = tf.contrib.crf.crf_decode(logits, crf_params, tf.reduce_sum(input_mask, axis=1))
# 加载模型参数
saver = tf.train.Saver()
with tf.Session() as sess:
saver.restore(sess, "model.ckpt")
# 加载测试数据
test_input_ids = [...] # 测试数据的 input_ids
test_input_mask = [...] # 测试数据的 input_mask
test_segment_ids = [...] # 测试数据的 segment_ids
# 预测结果
feed_dict = {
input_ids: np.array([test_input_ids]),
input_mask: np.array([test_input_mask]),
segment_ids: np.array([test_segment_ids])
}
pred_ids_result = sess.run(pred_ids, feed_dict=feed_dict)[0]
# 将预测结果转换为标签序列
pred_label_list = [label_list[id] for id in pred_ids_result]
print(pred_label_list)
```
这段代码使用了 TensorFlow 实现 BiLSTM-CRF 模型,并使用预训练的 BERT 模型作为输入。在使用时,需要将测试数据的 `input_ids`、`input_mask`、`segment_ids` 输入到模型中,并使用 `tf.contrib.crf.crf_decode()` 函数计算预测结果。最后将预测结果转换为标签序列即可。
阅读全文
相关推荐
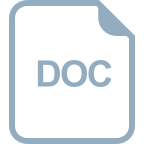
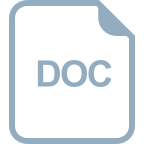
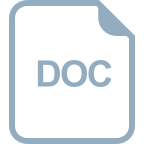
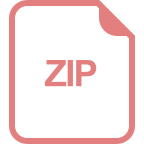




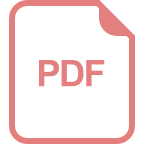
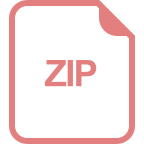
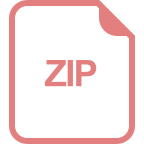
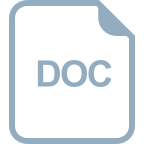
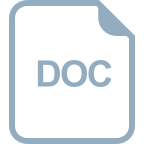
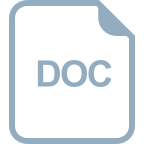
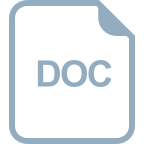



