python查询数据库number类型的字段,返回值中带有decimal,怎么去除
时间: 2024-12-18 11:28:49 浏览: 6
在Python中,如果你使用像SQLAlchemy、pymysql、sqlite3等库操作数据库,并从`number`类型的字段获取到包含小数的Decimal对象,通常直接打印或处理会显示为字符串形式。如果你想将Decimal转换回浮点数(float)以便后续处理,可以使用Decimal对象的`quantize`或`normalize`方法,或者通过除以10的幂来去除小数部分。
例如:
```python
from decimal import Decimal
# 假设你已经有了一个Decimal对象
decimal_value = Decimal('3.14159')
# 使用quantize去掉指定精度的小数部分
float_value = decimal_value.quantize(Decimal('0'))
# 或者直接转换成float(默认四舍五入)
float_value = float(decimal_value)
# 如果需要保留固定位数的小数,可以先转为字符串,然后切片
fixed_point_value = str(decimal_value).split('.')[1][:2] + '.0'
print(float_value) # 输出:3.14
print(fixed_point_value) # 输出:0.14
```
相关问题
python中怎么查询数据库某一字段的数字类型
在Python中,查询数据库某一字段是否为数字类型通常涉及到数据库操作和SQL查询,这取决于你使用的数据库驱动程序,如SQLite、MySQL、PostgreSQL等。以下是使用几种常见库的方法:
**1. SQLAlchemy(适用于多种数据库)**:
```python
from sqlalchemy import create_engine, MetaData
# 创建引擎
engine = create_engine('your_database_connection_string')
# 创建Metadata对象
metadata = MetaData()
# 获取表格信息并检查特定字段的数据类型
table_name = 'your_table_name'
column_name = 'your_column_name'
metadata.reflect(bind=engine)
for table in metadata.tables.values():
column = table.c[column_name]
if isinstance(column.type, Integer) or isinstance(column.type, Numeric):
print(f'{column_name} is of numeric type.')
```
**2. psycopg2(PostgreSQL)**:
```python
import psycopg2
with psycopg2.connect(database="your_database", user="your_user", password="your_password", host="localhost") as conn:
cursor = conn.cursor()
cursor.execute("SELECT column_name, data_type FROM information_schema.columns WHERE table_name='your_table'")
rows = cursor.fetchall()
for row in rows:
if 'numeric' in row[1]:
print(f'{row[0]} is a numeric type')
```
**3. pymysql(MySQL)**:
```python
import pymysql
with pymysql.connect(host='localhost', user='your_user', password='your_password', db='your_database') as connection:
with connection.cursor() as cursor:
sql = f"DESCRIBE your_table_name"
cursor.execute(sql)
columns = cursor.fetchall()
for col in columns:
if 'INT' in str(col[1]) or 'DECIMAL' in str(col[1]):
print(f'{col[0]} is of numeric type')
```
**4. SQLite(sqlite3库)**:
```python
import sqlite3
conn = sqlite3.connect('your_database.db')
cursor = conn.cursor()
cursor.execute("PRAGMA table_info(your_table_name);")
rows = cursor.fetchall()
for row in rows:
if row[2] == 'INTEGER' or row[2].startswith('REAL'):
print(f'{row[1]} is a numeric type')
conn.close()
```
请注意,上述代码示例假设你的数据库支持相应的信息获取函数。实际应用中,你需要根据所使用的数据库和连接方式调整代码。
python中怎么查询pymssql数据库某一字段的数字类型
在Python中,如果你使用`pymssql`库查询Pymssql数据库的某个字段的数字类型(如整数或浮点数),你需要执行SQL查询并解析结果。以下是一个基本示例:
```python
import pymssql
# 连接到数据库
server = 'your_server'
database = 'your_database'
username = 'your_username'
password = 'your_password'
conn = pymssql.connect(server, username, password, database)
cursor = conn.cursor()
# 假设你想查询名为'your_table'表里的'decimal_column'字段(假设它是数值类型)
sql_query = "SELECT decimal_column FROM your_table WHERE some_condition"
cursor.execute(sql_query) # 执行查询
# 获取所有行的结果
rows = cursor.fetchall()
# 遍历结果,检查每行的'decimal_column'是否为数字类型
for row in rows:
value = row[0] # 假设索引0对应'decimal_column'
if isinstance(value, (int, float)): # 使用isinstance检查数值类型
print(f"{value} is a numeric value")
# 关闭连接
cursor.close()
conn.close()
```
在这个例子中,我们首先执行SQL查询获取数据,然后遍历结果,检查每个值是否为整数(`int`)或浮点数(`float`)类型。如果需要更精确的类型判断,比如区分整数和浮点数,你可能需要使用`numbers`模块的`Number`或`Integral`等。
阅读全文
相关推荐
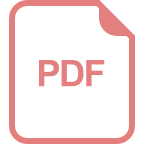
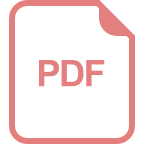
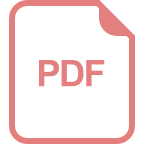













