matlab attention
时间: 2023-12-29 09:04:18 浏览: 137
在MATLAB中,注意力机制(Attention)是一种用于加强模型对输入数据中重要部分的关注的技术。它可以帮助模型更好地理解和利用输入数据的信息,提高模型的性能和准确性。
在引用和引用中提到了使用注意力机制的多变量时间序列预测模型和回归预测模型。这些模型结合了注意力机制和其他优化算法(如鲸鱼算法)来提高预测的准确性。
具体实现注意力机制的方法可能因模型而异,但一般步骤如下:
1. 准备数据集:将多个特征作为输入,单个变量作为输出的多变量时间序列数据集。
2. 构建模型:使用LSTM(长短期记忆)等神经网络模型作为基础模型,并在其上添加注意力机制。
3. 训练模型:使用数据集训练模型,优化模型参数,使其能够更好地预测输出变量。
4. 预测结果:使用训练好的模型对新的输入数据进行预测,并得到预测结果。
注意力机制可以帮助模型更好地关注输入数据中的重要特征,从而提高预测的准确性和稳定性。
相关问题
matlab self attention layer
在 MATLAB 中实现 Self-Attention Layer,可以按照以下步骤进行:
1. 定义一个自注意力层的类,该类应该继承自 matlab.mixin.CustomDisplay 类,以便在命令窗口中显示自定义信息。
2. 在类的构造函数中设置自注意力层的参数,包括输入向量的维度、自注意力头的数量、隐藏层的大小等。
3. 实现自注意力层的前向传播函数,该函数应该接受输入向量并返回自注意力层的输出。在前向传播函数中,需要实现自注意力机制,包括计算注意力权重、加权求和等操作。
4. 实现自注意力层的反向传播函数,该函数应该接收反向传播的梯度并返回输入向量的梯度。在反向传播函数中,需要计算注意力权重的导数、加权求和的导数等。
下面是一个简单的 MATLAB 自注意力层的示例代码:
```matlab
classdef SelfAttentionLayer < matlab.mixin.CustomDisplay
properties
input_dim
num_heads
hidden_dim
dropout_rate
query_weights
key_weights
value_weights
end
methods
function obj = SelfAttentionLayer(input_dim, num_heads, hidden_dim, dropout_rate)
obj.input_dim = input_dim;
obj.num_heads = num_heads;
obj.hidden_dim = hidden_dim;
obj.dropout_rate = dropout_rate;
obj.query_weights = randn(hidden_dim, input_dim);
obj.key_weights = randn(hidden_dim, input_dim);
obj.value_weights = randn(hidden_dim, input_dim);
end
function output = forward(obj, input)
batch_size = size(input, 1);
query = input * obj.query_weights';
key = input * obj.key_weights';
value = input * obj.value_weights';
query = reshape(query, [batch_size, obj.num_heads, obj.hidden_dim/obj.num_heads]);
key = reshape(key, [batch_size, obj.num_heads, obj.hidden_dim/obj.num_heads]);
value = reshape(value, [batch_size, obj.num_heads, obj.hidden_dim/obj.num_heads]);
attention_weights = softmax(query * permute(key, [1, 3, 2]) / sqrt(obj.hidden_dim/obj.num_heads), 3);
attention_weights = dropout(attention_weights, obj.dropout_rate);
output = reshape(attention_weights * value, [batch_size, obj.hidden_dim]);
end
function input_gradient = backward(obj, output_gradient, input)
batch_size = size(input, 1);
query = input * obj.query_weights';
key = input * obj.key_weights';
value = input * obj.value_weights';
query = reshape(query, [batch_size, obj.num_heads, obj.hidden_dim/obj.num_heads]);
key = reshape(key, [batch_size, obj.num_heads, obj.hidden_dim/obj.num_heads]);
value = reshape(value, [batch_size, obj.num_heads, obj.hidden_dim/obj.num_heads]);
attention_weights = softmax(query * permute(key, [1, 3, 2]) / sqrt(obj.hidden_dim/obj.num_heads), 3);
attention_weights = dropout(attention_weights, obj.dropout_rate);
output_gradient = reshape(output_gradient, [batch_size, obj.num_heads, obj.hidden_dim/obj.num_heads]);
value_gradient = attention_weights' * output_gradient;
attention_weights_gradient = output_gradient * permute(value, [1, 3, 2]);
attention_weights_gradient = attention_weights_gradient .* (attention_weights .* (1-attention_weights));
attention_weights_gradient = dropout(attention_weights_gradient, obj.dropout_rate);
query_gradient = attention_weights_gradient * key;
key_gradient = permute(query, [1, 3, 2]) * attention_weights_gradient;
input_gradient = (query_gradient + key_gradient + value_gradient) * obj.query_weights;
end
function displayScalarObject(obj)
disp(['SelfAttentionLayer with input_dim = ', num2str(obj.input_dim), ', num_heads = ', num2str(obj.num_heads), ', hidden_dim = ', num2str(obj.hidden_dim), ', dropout_rate = ', num2str(obj.dropout_rate)]);
end
end
end
```
该代码使用了随机初始化的权重矩阵,实现了自注意力层的前向传播和反向传播函数,并添加了自定义信息的显示功能。
添加attention matlab
在 MATLAB 中实现 Attention 通常需要使用神经网络工具箱(Neural Network Toolbox)中的函数,例如 `trainNetwork` 和 `sequenceInputLayer`。具体实现步骤如下:
1. 定义输入层和 LSTM 层
```matlab
inputSize = 100; % 输入向量的维度
numHiddenUnits = 200; % LSTM 层的隐藏单元数量
layers = [ ...
sequenceInputLayer(inputSize)
lstmLayer(numHiddenUnits)];
```
2. 定义 Attention 层
```matlab
attentionSize = 50; % Attention 层的大小
attentionLayer = attentionLayer(attentionSize);
layers = [layers
attentionLayer];
```
3. 定义输出层
```matlab
numClasses = 10; % 输出类别数量
outputLayer = fullyConnectedLayer(numClasses);
layers = [layers
outputLayer];
```
4. 定义训练选项
```matlab
options = trainingOptions('adam', ...
'MaxEpochs', 50, ...
'MiniBatchSize', 64, ...
'GradientThreshold', 1, ...
'Shuffle', 'every-epoch', ...
'Verbose', false);
```
5. 使用 `trainNetwork` 函数进行训练
```matlab
net = trainNetwork(XTrain, YTrain, layers, options);
```
其中,`XTrain` 和 `YTrain` 分别是训练数据和标签,`layers` 是定义好的网络层,`options` 是训练选项。
6. 使用训练好的模型进行预测
```matlab
YPred = classify(net, XTest);
```
其中,`XTest` 是测试数据,`YPred` 是预测结果。
以上是一个简单的实现过程,具体还需要根据具体情况进行调整。
阅读全文
相关推荐
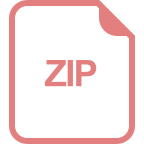
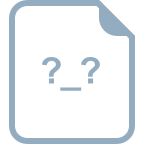
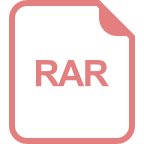
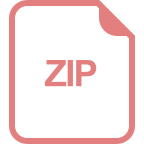












