java中知道学生出生日期怎么求年龄
时间: 2024-11-28 10:27:14 浏览: 12
在Java中,如果你有一个表示学生的类,比如`Student`,并且这个类有一个`birthDate`属性,代表学生的出生日期,你可以通过以下步骤计算学生的年龄:
1. 首先,你需要导入java.time包中的`LocalDate`和`YearMonth`类,这两个类可以方便地处理日期相关的操作。
```java
import java.time.LocalDate;
import java.time.Period;
```
2. 然后,在`Student`类或者某个方法里,假设当前日期是`LocalDate.now()`,你可以创建一个新的`LocalDate`对象,代表学生的生日,然后使用`Period.between`方法计算两个日期之间的年份差。
```java
LocalDate currentDate = LocalDate.now();
LocalDate birthDate = getBirthDateFromStudent(); // 获取学生出生日期的方法
Period age = Period.between(birthDate, currentDate);
int studentAge = age.getYears(); // 年龄就是年数
```
注意,这里假设`getBirthDateFromStudent()`是一个获取学生出生日期的方法,实际应用中需要你提供具体的实现。
相关问题
用java设计一个学生student类,包含学号、姓名、出生日期、性别等字段 从input.txt(从附件下载)中读取其中的学生信息,并存入学生集合list中 对学生集合list进行排序,按照出生日期从早到晚排序(即年龄由大到小) 将排序后的学生集合list输出到output.txt文件中,输出格式同input.txt:一行一个学生,依次输出学号、姓名、出生日期、性别并用空格隔开
好的,我可以帮你设计一个Java版的学生类并实现排序和输出。首先,让我们来看看学生类的实现。
```java
public class Student {
private String studentId;
private String name;
private LocalDate birthDate;
private String gender;
public Student(String studentId, String name, LocalDate birthDate, String gender) {
this.studentId = studentId;
this.name = name;
this.birthDate = birthDate;
this.gender = gender;
}
// getters and setters
}
```
以上是一个简单的Java版学生类实现,包含了学号、姓名、出生日期、性别等字段。注意出生日期使用了Java 8中的`LocalDate`类。
接下来,让我们来读取input.txt文件中的学生信息,并存入学生集合list中。
```java
List<Student> students = new ArrayList<>();
try (Scanner scanner = new Scanner(new File("input.txt"))) {
while (scanner.hasNextLine()) {
String[] data = scanner.nextLine().split(" ");
LocalDate birthDate = LocalDate.parse(data[2], DateTimeFormatter.ofPattern("yyyy-MM-dd"));
Student student = new Student(data[0], data[1], birthDate, data[3]);
students.add(student);
}
} catch (FileNotFoundException e) {
e.printStackTrace();
}
```
以上代码将会从input.txt文件中读取每一行学生信息,并将其存入一个学生对象中,最后添加到学生集合list中。注意出生日期的处理。
接下来,我们需要对学生集合list进行排序,按照出生日期从早到晚排序(即年龄由大到小)。
```java
students.sort(Comparator.comparing(Student::getBirthDate));
```
以上代码将会对学生集合list进行排序,按照出生日期从早到晚排序(即年龄由大到小)。
最后,我们需要将排序后的学生集合list输出到output.txt文件中,输出格式同input.txt:一行一个学生,依次输出学号、姓名、出生日期、性别并用空格隔开。
```java
try (PrintWriter writer = new PrintWriter(new File("output.txt"))) {
for (Student student : students) {
writer.printf("%s %s %s %s%n", student.getStudentId(), student.getName(),
student.getBirthDate().format(DateTimeFormatter.ofPattern("yyyy-MM-dd")), student.getGender());
}
} catch (FileNotFoundException e) {
e.printStackTrace();
}
```
以上代码将会将排序后的学生集合list输出到output.txt文件中,输出格式同input.txt:一行一个学生,依次输出学号、姓名、出生日期、性别并用空格隔开。
完整代码如下:
```java
import java.io.File;
import java.io.FileNotFoundException;
import java.io.PrintWriter;
import java.time.LocalDate;
import java.time.format.DateTimeFormatter;
import java.util.ArrayList;
import java.util.Comparator;
import java.util.List;
import java.util.Scanner;
public class Main {
public static void main(String[] args) {
List<Student> students = new ArrayList<>();
try (Scanner scanner = new Scanner(new File("input.txt"))) {
while (scanner.hasNextLine()) {
String[] data = scanner.nextLine().split(" ");
LocalDate birthDate = LocalDate.parse(data[2], DateTimeFormatter.ofPattern("yyyy-MM-dd"));
Student student = new Student(data[0], data[1], birthDate, data[3]);
students.add(student);
}
} catch (FileNotFoundException e) {
e.printStackTrace();
}
students.sort(Comparator.comparing(Student::getBirthDate));
try (PrintWriter writer = new PrintWriter(new File("output.txt"))) {
for (Student student : students) {
writer.printf("%s %s %s %s%n", student.getStudentId(), student.getName(),
student.getBirthDate().format(DateTimeFormatter.ofPattern("yyyy-MM-dd")), student.getGender());
}
} catch (FileNotFoundException e) {
e.printStackTrace();
}
}
}
```
希望能够帮助到你!
java中编写一个程序,实现一下功能,要求 设计一个学生student类,包含学号、姓名、出生日期、性别等字段 从input.txt(从附件下载)中读取其中的学生信息,并存入学生集合list中 对学生集合list进行排序,按照出生日期从早到晚排序(即年龄由大到小) 将排序后的学生集合list输出到output.txt文件中,输出格式同input.txt:一行一个学生,依次输出学号、姓名、出生日期、性别并用空格隔开
以下是实现该功能的Java程序:
```java
import java.io.File;
import java.io.FileWriter;
import java.io.IOException;
import java.time.LocalDate;
import java.time.format.DateTimeFormatter;
import java.util.ArrayList;
import java.util.Collections;
import java.util.Comparator;
import java.util.List;
import java.util.Scanner;
public class StudentSorter {
public static void main(String[] args) {
// 从 input.txt 中读取学生信息
List<Student> students = readStudentsFromFile("input.txt");
// 对学生集合进行排序
Collections.sort(students, new Comparator<Student>() {
public int compare(Student s1, Student s2) {
return s1.getBirthDate().compareTo(s2.getBirthDate());
}
});
// 将排序后的学生集合输出到 output.txt 文件中
writeStudentsToFile(students, "output.txt");
System.out.println("学生信息已排序并输出到 output.txt 文件中。");
}
private static List<Student> readStudentsFromFile(String filePath) {
List<Student> students = new ArrayList<>();
File file = new File(filePath);
try (Scanner scanner = new Scanner(file)) {
while (scanner.hasNextLine()) {
String line = scanner.nextLine();
String[] fields = line.split("\\s+");
String id = fields[0];
String name = fields[1];
LocalDate birthDate = LocalDate.parse(fields[2], DateTimeFormatter.ofPattern("yyyy-MM-dd"));
char gender = fields[3].charAt(0);
Student student = new Student(id, name, birthDate, gender);
students.add(student);
}
} catch (IOException e) {
System.out.println("读取文件出错:" + e.getMessage());
}
return students;
}
private static void writeStudentsToFile(List<Student> students, String filePath) {
File file = new File(filePath);
try (FileWriter writer = new FileWriter(file)) {
for (Student student : students) {
String line = student.getId() + " " + student.getName() + " "
+ student.getBirthDate().format(DateTimeFormatter.ofPattern("yyyy-MM-dd")) + " "
+ student.getGender();
writer.write(line + "\n");
}
} catch (IOException e) {
System.out.println("写入文件出错:" + e.getMessage());
}
}
}
class Student {
private String id;
private String name;
private LocalDate birthDate;
private char gender;
public Student(String id, String name, LocalDate birthDate, char gender) {
this.id = id;
this.name = name;
this.birthDate = birthDate;
this.gender = gender;
}
public String getId() {
return id;
}
public String getName() {
return name;
}
public LocalDate getBirthDate() {
return birthDate;
}
public char getGender() {
return gender;
}
}
```
程序的主要步骤如下:
1. 定义一个 `Student` 类表示学生,包含学号、姓名、出生日期、性别等字段。
2. 从 `input.txt` 文件中读取学生信息,并将每个学生信息封装成一个 `Student` 对象存入一个 `List<Student>` 集合中。
3. 使用 `Collections.sort()` 方法对学生集合进行排序,按照出生日期从早到晚排序。
4. 将排序后的学生集合输出到 `output.txt` 文件中,输出格式与 `input.txt` 文件相同。
5. 定义一个 `readStudentsFromFile()` 方法读取文件中的学生信息,返回一个 `List<Student>` 集合。
6. 定义一个 `writeStudentsToFile()` 方法将学生集合写入文件,写入格式与 `input.txt` 文件相同。
阅读全文
相关推荐
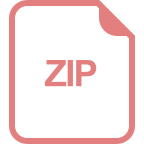
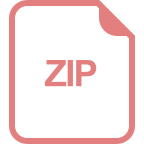
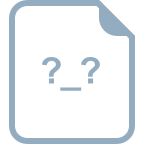










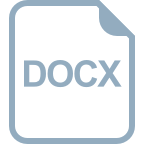