java中编写一个程序,实现一下功能,要求: 1.设计一个学生student类,包含学号、姓名、出生日期、性别等字段。 2.从input.txt(从附件下载)中读取其中的学生信息,并存入学生集合list中。 3.对学生集合list进行排序,按照出生日期从早到晚排序(即年龄由大到小)。 4.将排序后的学生集合list输出到output.txt文件中,输出格式同input.txt:一行一个学生,依次输出学号、姓名、出生日期、性别并用空格隔开。
时间: 2024-02-05 08:11:48 浏览: 160
下面是实现上述功能的Java程序:
```java
import java.io.File;
import java.io.FileWriter;
import java.io.IOException;
import java.text.ParseException;
import java.text.SimpleDateFormat;
import java.util.Collections;
import java.util.Comparator;
import java.util.Date;
import java.util.List;
import java.util.ArrayList;
import java.util.Scanner;
public class Main {
public static void main(String[] args) {
List<Student> students = readStudentsFromFile("input.txt");
Collections.sort(students, new StudentComparator());
writeStudentsToFile(students, "output.txt");
}
private static List<Student> readStudentsFromFile(String fileName) {
List<Student> students = new ArrayList<>();
try (Scanner scanner = new Scanner(new File(fileName))) {
while (scanner.hasNextLine()) {
String line = scanner.nextLine();
String[] fields = line.split("\\s+");
int id = Integer.parseInt(fields[0]);
String name = fields[1];
Date birthDate = new SimpleDateFormat("yyyy-MM-dd").parse(fields[2]);
char gender = fields[3].charAt(0);
students.add(new Student(id, name, birthDate, gender));
}
} catch (IOException | ParseException e) {
e.printStackTrace();
}
return students;
}
private static void writeStudentsToFile(List<Student> students, String fileName) {
try (FileWriter writer = new FileWriter(new File(fileName))) {
for (Student student : students) {
writer.write(student.toString() + "\n");
}
} catch (IOException e) {
e.printStackTrace();
}
}
static class Student {
private int id;
private String name;
private Date birthDate;
private char gender;
public Student(int id, String name, Date birthDate, char gender) {
this.id = id;
this.name = name;
this.birthDate = birthDate;
this.gender = gender;
}
public int getId() {
return id;
}
public String getName() {
return name;
}
public Date getBirthDate() {
return birthDate;
}
public char getGender() {
return gender;
}
@Override
public String toString() {
SimpleDateFormat format = new SimpleDateFormat("yyyy-MM-dd");
return String.format("%d %s %s %c", id, name, format.format(birthDate), gender);
}
}
static class StudentComparator implements Comparator<Student> {
@Override
public int compare(Student s1, Student s2) {
return s1.getBirthDate().compareTo(s2.getBirthDate());
}
}
}
```
该程序首先读取输入文件中的学生信息,并将其存入一个 `List<Student>` 集合中,然后使用 `Collections.sort` 函数对该集合进行排序,排序的依据是学生的出生日期。排序完成后,程序将排序后的学生集合写入输出文件。
该程序中使用了一个 `Student` 类来表示学生,包含学号、姓名、出生日期和性别等字段,同时还定义了一个 `StudentComparator` 类来实现学生按照出生日期排序的比较器。程序中还使用了 Java 的 IO 类库来读写文件。
注意,程序中使用了 try-with-resources 结构来确保文件资源在使用完毕后被正确关闭。此外,程序中还对可能出现的异常进行了处理,以避免程序崩溃。
阅读全文
相关推荐
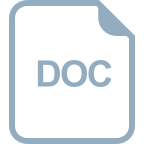
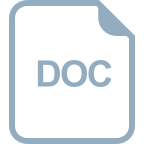
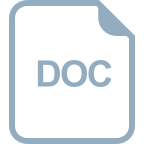













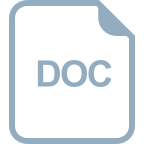
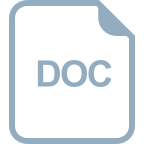