目前mcu每20ms发送一组数据,C语言开发一个demo从uart接口上获取MCU发送的数据,并打印出来
时间: 2024-12-24 13:36:16 浏览: 3
在C语言中,开发一个UART接口程序以接收MCU每20毫秒发送的一组数据并打印,首先需要准备好硬件连接、初始化UART通信以及设置定时器来处理间隔。以下是一个简化的示例代码:
```c
#include <stdio.h>
#include <stdint.h>
#include <fcntl.h> // 包含文件描述符操作
#include <termios.h> // 包含控制终端输入输出
// 宏定义UART设备路径和波特率
#define UART_DEV "/dev/ttyUSB0"
#define BAUD_RATE 9600
void setup_uart(int fd) {
struct termios options;
tcgetattr(fd, &options); // 获取当前配置
cfsetispeed(&options, BAUD_RATE); // 设置波特率
cfsetospeed(&options, BAUD_RATE);
cfmakeraw(&options); // 将模式变为无流控
options.c_cflag &= ~(CSIZE | PARENB); // 清除奇偶校验位
options.c_cflag |= CS8; // 设置8位数据
options.c_iflag &= ~(IXON | IXOFF | IGNPAR); // 关闭流量控制和忽略空闲字符
options.c_oflag &= ~OPOST; // 关闭输出过程中的特殊字符处理
options.c_lflag &= ~(ICANON | ECHO); // 关闭标准输入的缓冲区和回显
tcflush(fd, TCIOFLUSH); // 清理缓冲区
tcsetattr(fd, TCSANOW, &options); // 应用新设置
}
void read_data(int fd, uint8_t *data, size_t len) {
ssize_t bytes_received = read(fd, data, len);
if (bytes_received > 0) {
printf("Received %zd bytes: ", bytes_received);
for (size_t i = 0; i < bytes_received; i++) {
printf("%02x ", data[i]);
}
printf("\n");
} else {
perror("Error reading from UART");
}
}
int main() {
int uart_fd = open(UART_DEV, O_RDWR | O_NOCTTY | O_NDELAY);
if (uart_fd == -1) {
perror("Failed to open UART device");
return 1;
}
setup_uart(uart_fd);
while (true) {
uint8_t buffer[20];
read_data(uart_fd, buffer, sizeof(buffer));
// 模拟20ms延时
// 如果真实场景中有定时器,可以替换为如下的代码:
/* struct timespec delay;
* nanosleep(&delay, NULL);
* delay.tv_sec = 0;
* delay.tv_nsec = 20 * 1000000; // 20 ms in nanoseconds */
sleep(0.02); // 真实环境应使用RTOS的API来管理时间
}
close(uart_fd);
return 0;
}
```
阅读全文
相关推荐
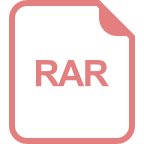
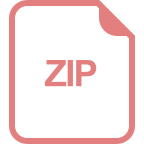
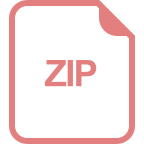
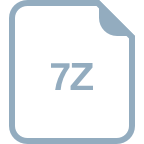
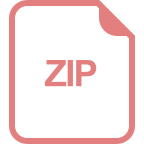
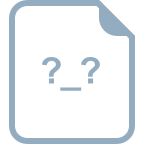
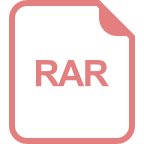
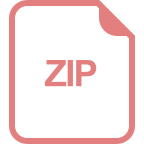
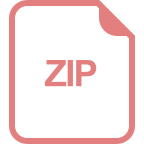
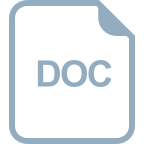
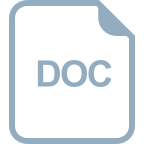
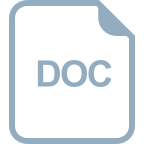
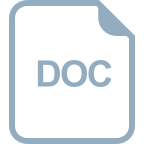
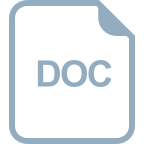
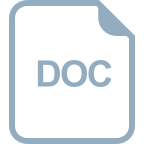
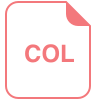
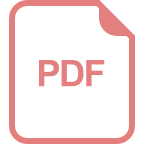