如何判断一个数组下标在不在vector表内
时间: 2024-09-09 10:16:55 浏览: 40
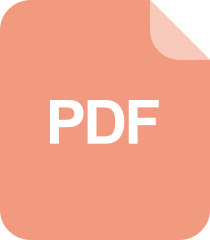
下标操作符重载模拟多维数组详解
在C++中,可以使用`std::vector`来存储一系列元素,并且可以通过下标来访问这些元素。若要判断一个特定的数组下标是否存在于`std::vector`表内,我们可以采用以下方法:
1. 直接访问判断:尝试通过下标访问`std::vector`中的元素,然后捕获可能发生的异常。如果访问成功,则表示该下标存在;如果抛出`std::out_of_range`异常,则表示该下标不存在。
```cpp
#include <iostream>
#include <vector>
#include <stdexcept>
int main() {
std::vector<int> vec = {1, 2, 3, 4, 5};
int index = 3; // 假设我们要检查这个下标
try {
// 尝试访问下标为index的元素
int element = vec[index];
std::cout << "下标 " << index << " 存在,元素值为 " << element << std::endl;
} catch (const std::out_of_range& e) {
std::cout << "下标 " << index << " 不存在" << std::endl;
}
return 0;
}
```
2. 使用`std::find`:如果`std::vector`中的元素是可比较的,可以使用`std::find`来查找特定值是否存在于`std::vector`中,然后根据返回的迭代器判断元素是否被找到。
```cpp
#include <iostream>
#include <vector>
#include <algorithm> // 包含std::find
int main() {
std::vector<int> vec = {1, 2, 3, 4, 5};
int valueToFind = 3; // 假设我们要检查这个值
if (std::find(vec.begin(), vec.end(), valueToFind) != vec.end()) {
std::cout << "值 " << valueToFind << " 存在于vector中" << std::endl;
} else {
std::cout << "值 " << valueToFind << " 不存在于vector中" << std::endl;
}
return 0;
}
```
在实际使用中,第一种方法使用下标访问是一种更为直接的方式,尤其适用于下标值需要被频繁使用时。第二种方法则适用于需要查找特定值而不在乎其位置的情况。
阅读全文
相关推荐
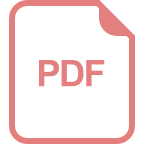
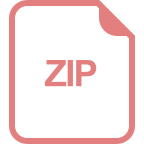














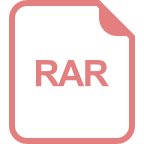
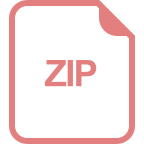
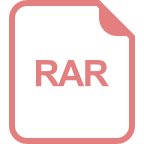