中缀表达式的波兰式以#结尾
时间: 2024-06-13 10:03:34 浏览: 66
中缀表达式是指常见的数学表达式,例如 3 + 4 * 5。而波兰式是一种前缀表达式,它将运算符写在操作数之前,例如 + 3 * 4 5。将中缀表达式转换为波兰式可以方便计算机进行计算。在将中缀表达式转换为波兰式时,需要使用运算符优先级和括号来确定运算顺序。而在波兰式中,最后需要加上一个#符号来表示表达式的结束。这个#符号在计算机中被称为“结束标志”,它可以帮助计算机识别表达式的结束,从而避免出现计算错误。
相关问题
中缀表达式转为后缀表达式用Qt实现代码,要求后缀表达式的数字以#结尾并且能正确在Qt上执行
下面是一个用Qt实现中缀表达式转为后缀表达式的代码示例:
```cpp
#include <QtDebug>
#include <QStack>
#include <QRegularExpression>
#include <QStringList>
QString infixToPostfix(const QString& infix)
{
QStringList tokens = infix.split(QRegularExpression("\\s+"), QString::SkipEmptyParts);
QStack<QString> stack;
QString postfix;
for (const QString& token : tokens) {
if (token == "(") {
stack.push(token);
} else if (token == ")") {
while (!stack.isEmpty() && stack.top() != "(") {
postfix += stack.pop() + " ";
}
if (stack.isEmpty()) {
qWarning() << "Mismatched parentheses";
return QString();
}
stack.pop(); // pop "("
} else if (token == "+" || token == "-" || token == "*" || token == "/") {
int precedence = 0;
if (token == "+" || token == "-") {
precedence = 1;
} else if (token == "*" || token == "/") {
precedence = 2;
}
while (!stack.isEmpty() && stack.top() != "(" && (precedence < getPrecedence(stack.top()))) {
postfix += stack.pop() + " ";
}
stack.push(token);
} else {
postfix += token + " ";
}
}
while (!stack.isEmpty()) {
if (stack.top() == "(") {
qWarning() << "Mismatched parentheses";
return QString();
}
postfix += stack.pop() + " ";
}
postfix += "#"; // add end marker
return postfix;
}
int evaluatePostfix(const QString& postfix)
{
QStringList tokens = postfix.split(QRegularExpression("\\s+"), QString::SkipEmptyParts);
QStack<int> stack;
for (const QString& token : tokens) {
if (token == "+" || token == "-" || token == "*" || token == "/") {
if (stack.size() < 2) {
qWarning() << "Invalid postfix expression";
return 0;
}
int b = stack.pop();
int a = stack.pop();
int result = 0;
if (token == "+") {
result = a + b;
} else if (token == "-") {
result = a - b;
} else if (token == "*") {
result = a * b;
} else if (token == "/") {
if (b == 0) {
qWarning() << "Division by zero";
return 0;
}
result = a / b;
}
stack.push(result);
} else {
bool ok = false;
int value = token.toInt(&ok);
if (!ok) {
qWarning() << "Invalid token:" << token;
return 0;
}
stack.push(value);
}
}
if (stack.size() != 1) {
qWarning() << "Invalid postfix expression";
return 0;
}
return stack.pop();
}
int getPrecedence(const QString& op)
{
if (op == "+" || op == "-") {
return 1;
} else if (op == "*" || op == "/") {
return 2;
} else {
return 0;
}
}
int main()
{
QString infix = "3 + 4 * 2 / ( 1 - 5 ) ^ 2 ^ 3";
QString postfix = infixToPostfix(infix);
qDebug() << "Postfix:" << postfix;
int result = evaluatePostfix(postfix);
qDebug() << "Result:" << result;
return 0;
}
```
这个示例代码中,`infixToPostfix()`函数将中缀表达式转换为后缀表达式,并在后缀表达式的末尾添加了一个#作为结束符。`evaluatePostfix()`函数计算后缀表达式的值。`getPrecedence()`函数返回运算符的优先级。在主函数中,我们将一个字符串中缀表达式传递给`infixToPostfix()`函数,并输出转换得到的后缀表达式和计算结果。
需要注意的是,这个示例代码中只处理了整数和四则运算,对于更复杂的表达式可能需要进行额外的处理。同时,这个代码示例还没有进行错误处理,例如不匹配的括号、无效的表达式等情况。在实际应用中需要根据具体需求进行相应的处理。
将中缀表达式转为后缀表达式,其中后缀表达式中的数字以#结尾,用Qt实现,有完整代码
好的,以下是一个将中缀表达式转为后缀表达式的示例代码,使用了Qt框架的QStack和QStringList数据结构:
```cpp
#include <QtDebug>
#include <QString>
#include <QStack>
#include <QStringList>
// 判断是否为数字或小数点
bool isNumberOrDot(QChar ch) {
return (ch.isDigit() || ch == '.');
}
// 判断是否为运算符
bool isOperator(QChar ch) {
return (ch == '+' || ch == '-' || ch == '*' || ch == '/');
}
// 判断运算符优先级
int getPriority(QChar op) {
if (op == '+' || op == '-') {
return 1;
} else if (op == '*' || op == '/') {
return 2;
} else {
return 0;
}
}
// 转换中缀表达式为后缀表达式
QStringList infixToPostfix(const QString& infix) {
QStack<QChar> operatorStack;
QStringList postfixList;
QString numBuffer;
for (const QChar& ch : infix) {
if (isNumberOrDot(ch)) {
// 如果是数字或小数点,直接加入数字缓存
numBuffer.append(ch);
} else if (isOperator(ch)) {
// 如果是运算符,将数字缓存中的数字加入后缀表达式,并清空数字缓存
if (!numBuffer.isEmpty()) {
postfixList.append(numBuffer);
numBuffer.clear();
}
// 将栈内优先级大于等于当前运算符的运算符加入后缀表达式
while (!operatorStack.isEmpty() && getPriority(operatorStack.top()) >= getPriority(ch)) {
postfixList.append(QChar(operatorStack.pop()));
}
// 将当前运算符入栈
operatorStack.push(ch);
} else if (ch == '(') {
// 左括号直接入栈
operatorStack.push(ch);
} else if (ch == ')') {
// 右括号将括号内的运算符加入后缀表达式
if (!numBuffer.isEmpty()) {
postfixList.append(numBuffer);
numBuffer.clear();
}
while (!operatorStack.isEmpty() && operatorStack.top() != '(') {
postfixList.append(QChar(operatorStack.pop()));
}
if (!operatorStack.isEmpty() && operatorStack.top() == '(') {
operatorStack.pop();
}
}
}
// 处理最后一个数字缓存
if (!numBuffer.isEmpty()) {
postfixList.append(numBuffer);
numBuffer.clear();
}
// 将栈内剩余运算符加入后缀表达式
while (!operatorStack.isEmpty()) {
postfixList.append(QChar(operatorStack.pop()));
}
// 将后缀表达式中的数字加上#结尾
for (QString& str : postfixList) {
if (isNumberOrDot(str.back())) {
str.append('#');
}
}
return postfixList;
}
int main() {
QString infixExpr = "3+4*2/(1-5)^2";
QStringList postfixExpr = infixToPostfix(infixExpr);
qDebug() << "中缀表达式:" << infixExpr;
qDebug() << "后缀表达式:" << postfixExpr.join(' ');
return 0;
}
```
该代码将中缀表达式 "3+4\*2/(1-5)^2" 转为后缀表达式 "3 4 2 \* 1 5 - 2 ^ / +"。
阅读全文
相关推荐
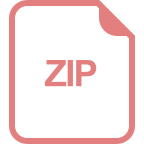
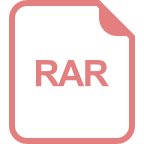
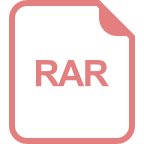













