学生成绩管理系统devc++
时间: 2023-11-26 21:47:24 浏览: 318
学生成绩管理系统是一个常见的应用程序,可以帮助学校或老师管理学生的成绩信息。在Dev-C++中,可以使用C++语言编写学生成绩管理系统。
以下是一个简单的学生成绩管理系统的示例代码:
```cpp
#include <iostream>
#include <fstream>
#include <string>
using namespace std;
const int MAX_STUDENT_NUM = 100;
struct Student {
string class_0;
int num;
string name;
float elec;
float c_program;
float media;
float english;
float math;
float physics;
float sport;
float polity;
float average;
};
int main() {
int student_num;
Student stu[MAX_STUDENT_NUM];
// 从文件中读取学生信息
ifstream infile("students.txt");
infile >> student_num;
for (int i = 0; i < student_num; i++) {
infile >> stu[i].class_0 >> stu[i].num >> stu[i].name >> stu[i].elec >> stu[i].c_program >> stu[i].media >> stu[i].english >> stu[i].math >> stu[i].physics >> stu[i].sport >> stu[i].polity >> stu[i].average;
}
infile.close();
// 计算每个学生的平均分
for (int i = 0; i < student_num; i++) {
stu[i].average = (stu[i].elec + stu[i].c_program + stu[i].media + stu[i].english + stu[i].math + stu[i].physics + stu[i].sport + stu[i].polity) / 8.0;
}
// 输出每个学生的信息
for (int i = 0; i < student_num; i++) {
cout << stu[i].class_0 << " " << stu[i].num << " " << stu[i].name << " " << stu[i].elec << " " << stu[i].c_program << " " << stu[i].media << " " << stu[i].english << " " << stu[i].math << " " << stu[i].physics << " " << stu[i].sport << " " << stu[i].polity << " " << stu[i].average << endl;
}
// 将学生信息写入文件
ofstream outfile("students.txt");
outfile << student_num << endl;
for (int i = 0; i < student_num; i++) {
outfile << stu[i].class_0 << " " << stu[i].num << " " << stu[i].name << " " << stu[i].elec << " " << stu[i].c_program << " " << stu[i].media << " " << stu[i].english << " " << stu[i].math << " " << stu[i].physics << " " << stu[i].sport << " " << stu[i].polity << " " << stu[i].average << endl;
}
outfile.close();
return 0;
}
```
该程序使用结构体Student来存储每个学生的信息,包括班级、学号、姓名、各科成绩和平均分。程序从文件中读取学生信息,计算每个学生的平均分,并将学生信息输出到控制台和文件中。
阅读全文
相关推荐







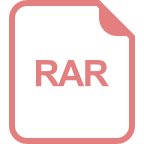






