C++如何删除文件内容(指定的字符开始,直到指定的字符)
时间: 2024-10-07 12:02:52 浏览: 9
在C++中,要删除文件内容(从某个字符开始到另一个字符结束),通常需要读取整个文件,然后基于指定的起始和终止字符创建一个新的内容,最后再将这个新内容写回原文件。这涉及到文件I/O操作以及字符串处理。这里有一个简单的示例,假设你想从每个行的开始位置删除至特定的终止字符:
```cpp
#include <fstream>
#include <iostream>
#include <string>
void delete_content_between_chars(const std::string& filename, char start_char, char end_char) {
std::ifstream inputFile(filename);
if (!inputFile) {
std::cerr << "Error opening file: " << filename << std::endl;
return;
}
std::ofstream outputFile(filename, std::ios::out | std::ios::trunc); // truncate the file before writing new content
if (!outputFile) {
std::cerr << "Error creating output file: " << filename << std::endl;
return;
}
std::string line;
while (std::getline(inputFile, line)) {
size_t startIndex = line.find(start_char);
if (startIndex != std::string::npos) {
size_t endIndex = line.find(end_char, startIndex + 1);
if (endIndex == std::string::npos) { // 如果找不到终止字符,则保留到文件末尾
outputFile << line.substr(startIndex + 1);
} else {
outputFile << line.substr(startIndex + 1, endIndex - startIndex - 1); // 删除指定范围的内容并写入
}
} else {
outputFile << line; // 如果没找到起始字符,直接写入原行
}
}
inputFile.close();
outputFile.close();
}
// 使用示例
int main() {
delete_content_between_chars("example.txt", '(', ')');
return 0;
}
```
注意,这个例子假设文件是可以追加的文本文件,并且输入文件存在且可以被读取。对于二进制文件或其他特殊情况,可能会有所不同。
相关推荐
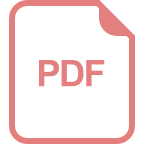
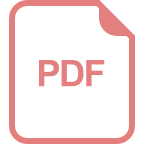
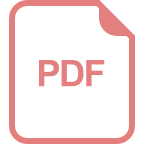














