Python求一个长方形的面积和周长,要提示输入半径
时间: 2024-09-23 12:07:44 浏览: 79
在Python中,计算长方形的面积和周长通常涉及两个变量,长度(length)和宽度(width)。如果你需要用户输入半径来计算长方形,首先我们需要将半径转换成边长,因为长方形是由两条相等的边组成的。以下是步骤:
1. 提示用户输入长方形的一条边的长度,而不是半径。
2. 如果用户提供的数据是圆形的半径,你需要先计算出边长,例如对于正方形,可以取圆的直径作为边长;对于一般矩形,可以假设半径对应的是一条对角线长度,然后除以√2得到一条边。
下面是一个简单的示例代码片段,用于获取用户输入并计算面积和周长:
```python
import math
# 提示用户输入长方形的边长或半径
side_length = float(input("请输入长方形的边长(如果是半径请输入圆的半径,系统会自动转换): "))
# 长方形的周长公式:2 * (长度 + 宽度)
perimeter = 2 * side_length
# 长方形的面积公式:长度 * 宽度
area = side_length ** 2
# 如果用户输入的是半径,则计算对应边长
if "半径" in input.lower(): # 检查用户是否提到“半径”
radius = side_length
side_length = 2 * radius # 对于正方形或矩形,边长是直径
print(f"长方形的周长是: {perimeter}")
print(f"长方形的面积是: {area}")
相关问题
定义一个类,里面存放一个公有方法求长方形面积和周长 。再定义一个私有方法求圆的面积和周长。长方形的长和宽、圆的半径从键盘输入
在面向对象编程中,你可以创建一个类来封装计算几何形状面积和周长的行为。下面是一个简单的Python类的例子,用于表示长方形和圆:
```python
class GeometryShape:
def __init__(self):
self.rectangle_length = float(input("请输入长方形的长度: "))
self.rectangle_width = float(input("请输入长方形的宽度: "))
def calculate_rectangle_area(self):
"""
公共方法计算长方形面积
"""
area = self.rectangle_length * self.rectangle_width
print(f"长方形面积: {area}")
def calculate_rectangle_perimeter(self):
"""
公共方法计算长方形周长
"""
perimeter = 2 * (self.rectangle_length + self.rectangle_width)
print(f"长方形周长: {perimeter}")
# 私有方法,不可直接通过实例调用
def _calculate_circle_area_and_perimeter(self, radius):
import math
"""
私有方法,用于计算圆的面积和周长
"""
circle_area = math.pi * (radius ** 2)
circle_circumference = 2 * math.pi * radius
return circle_area, circle_circumference
# 由于私有方法不能直接访问,需要通过公共方法间接操作
def calculate_circle_info(shape, radius):
circle_data = shape._calculate_circle_area_and_perimeter(radius)
print(f"圆的面积: {circle_data[0]}, 圆的周长: {circle_data[1]}")
# 示例中不直接调用私有方法
if __name__ == "__main__":
rectangle = GeometryShape()
rectangle.calculate_rectangle_area()
rectangle.calculate_rectangle_perimeter()
radius = float(input("请输入圆的半径: "))
calculate_circle_info(rectangle, radius)
```
在这个例子中,`GeometryShape`类包含了长方形的属性和公共方法。用户可以输入长方形的长和宽来获取面积和周长。而计算圆的面积和周长是一个内部细节,我们将其封装在一个私有方法 `_calculate_circle_area_and_perimeter` 中,外部用户无法直接调用它。如果你想让外部也能计算圆的面积和周长,可以通过辅助函数 `calculate_circle_info` 来间接实现。
定义成员方法求圆的面积、周长和长方形的面积
以下是一个示例的 Python 类,其中包含计算圆和长方形的面积以及圆的周长的成员方法:
```python
class Geometry:
def __init__(self, radius, length, width):
self.radius = radius
self.length = length
self.width = width
def circle_area(self):
return 3.14 * self.radius ** 2
def circle_circumference(self):
return 2 * 3.14 * self.radius
def rectangle_area(self):
return self.length * self.width
```
在这个类中,我们使用了构造函数 `__init__` 来初始化圆的半径和长方形的长度和宽度。然后,我们定义了三个成员方法:`circle_area` 计算圆的面积,`circle_circumference` 计算圆的周长,以及 `rectangle_area` 计算长方形的面积。
以下是使用该类的示例代码:
```python
g = Geometry(5, 10, 20)
print("Circle area:", g.circle_area())
print("Circle circumference:", g.circle_circumference())
print("Rectangle area:", g.rectangle_area())
```
输出:
```
Circle area: 78.5
Circle circumference: 31.400000000000002
Rectangle area: 200
```
在此示例中,我们创建了一个 `Geometry` 的对象 `g`,并将圆的半径设置为 5,长方形的长度设置为 10,宽度设置为 20。然后,我们调用了 `circle_area`, `circle_circumference`, 和 `rectangle_area` 方法来计算圆和长方形的面积以及圆的周长。
阅读全文
相关推荐
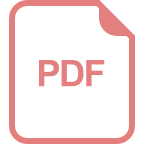














