linux c++创建线程
时间: 2024-12-30 07:24:57 浏览: 8
### 创建线程的方法
#### 使用 `pthread` 库创建线程
在Linux环境中,可以利用POSIX线程库(`pthread`)来创建和管理线程。下面是一个简单的例子展示如何通过命令行编译并执行一个多线程的应用程序[^1]。
```cpp
#include <iostream>
#include <pthread.h>
void* thread_function(void*) {
std::cout << "Thread is running\n";
pthread_exit(0);
}
int main() {
pthread_t thread;
int ret = pthread_create(&thread, NULL, thread_function, NULL);
if (ret != 0) {
std::cerr << "Error creating thread\n";
return -1;
}
void *result;
pthread_join(thread, &result); // Wait for the thread to finish its execution.
std::cout << "Thread joined successfully.\n";
return 0;
}
```
为了编译上述代码,在终端输入如下指令:
```bash
g++ -lpthread pthread_example.cpp -o pthread_example
```
接着可以通过运行生成的可执行文件查看效果:
```bash
./pthread_example
```
#### 利用 C++11 标准库中的 `<thread>` 头文件
自C++11起引入了标准模板库(STL),其中包含了更现代化的方式来处理并发操作——即std::thread类。这种方式不需要额外链接任何第三方库,并且语法更加直观友好。这里提供了一个简单案例说明怎样启动一个新的工作线程去完成特定的任务[^2]。
```cpp
#include <iostream>
#include <thread>
void task(int id){
std::cout<<"Task "<<id<<": Running...\n";
}
int main(){
const unsigned num_threads=10;
std::thread threads[num_threads];
for(unsigned i=0;i<num_threads;++i){
threads[i]=std::thread(task,i+1);
}
for(auto& th : threads){
th.join(); // Ensure all threads complete before program ends.
}
return 0;
}
```
此段代码可以直接使用 g++ 编译器进行编译而无需指定 `-lpthread` 参数,因为从 C++11 开始,编译器会自动识别到需要链接 pthread 库。
阅读全文
相关推荐



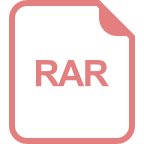

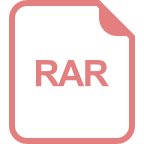

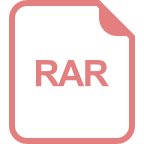
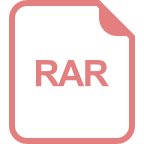


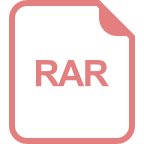





