python 测距补偿算法
时间: 2023-11-07 18:49:24 浏览: 133
Python中的测距补偿算法可以通过多种方式实现,具体取决于你使用的传感器和测距技术。以下是一种常见的测距补偿算法示例,用于基于超声波传感器的测距:
```python
import math
def distance_compensation(raw_distance, temperature):
speed_of_sound = 331.4 + 0.6 * temperature # 根据温度计算声速,单位为 m/s
compensated_distance = raw_distance * speed_of_sound / 2 # 计算补偿后的距离
return compensated_distance
# 例如,假设原始距离为10 cm,温度为25摄氏度
raw_distance = 10
temperature = 25
compensated_distance = distance_compensation(raw_distance, temperature)
print("补偿后的距离:", compensated_distance, "cm")
```
在这个示例中,我们根据温度计算声速,并将其用于距离补偿计算。需要注意的是,这只是一个简单的示例,实际应用中可能需要更复杂的算法来考虑其他因素,例如空气湿度、传感器特性等。
如果你使用的是其他传感器或测距技术,请提供更具体的信息,以便我能够给出适合的补偿算法。
相关问题
车辆测距常见算法python
常见的车辆测距算法包括三角测距法和视差测距法。以下是使用Python实现这两种算法的示例代码:
1. 三角测距法:
```python
import math
def calculate_distance(focal_length, actual_width, perceived_width):
distance = (actual_width * focal_length) / perceived_width
return distance
focal_length = 1000 # 焦距(单位:像素)
actual_width = 2 # 实际宽度(单位:米)
perceived_width = 200 # 在图像中测得的宽度(单位:像素)
distance = calculate_distance(focal_length, actual_width, perceived_width)
print("车辆距离: ", distance, "米")
```
2. 视差测距法:
```python
def calculate_distance(baseline, focal_length, disparity):
distance = (baseline * focal_length) / disparity
return distance
baseline = 3 # 基线长度(单位:米)
focal_length = 1000 # 焦距(单位:像素)
disparity = 50 # 视差(单位:像素)
distance = calculate_distance(baseline, focal_length, disparity)
print("车辆距离: ", distance, "米")
```
这些代码只是简单的示例,实际使用时需要根据具体的相机参数和图像处理流程进行调整。
光线补偿算法 python
光线补偿是一种用于图像增强的技术,它可以根据图像的亮度和对比度,对图像进行自动调整以提高图像的可视性和质量。下面是一个基于Python的简单光线补偿算法:
```python
import cv2
def lighting_compensation(img, a=0.02, b=0.0):
# 对图像进行灰度化处理
gray = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
# 计算图像的平均亮度
mean = cv2.mean(gray)[0]
# 根据公式计算补偿系数
alpha = 1.0 / (1.0 - a * mean)
beta = b - alpha * mean
# 对图像进行补偿处理
img = cv2.convertScaleAbs(img, alpha=alpha, beta=beta)
return img
```
在上面的代码中,我们首先将输入图像转换为灰度图像。然后,我们计算灰度图像的平均亮度,并根据公式计算补偿系数alpha和beta。最后,我们使用cv2.convertScaleAbs函数对图像进行补偿处理。alpha和beta的值可以根据实际情况进行调整以达到最佳效果。
使用示例:
```python
import cv2
# 读取图像
img = cv2.imread('test.jpg')
# 进行光线补偿
img = lighting_compensation(img)
# 显示结果
cv2.imshow('Result', img)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
在上面的示例代码中,我们读取一张图片并使用光线补偿算法对其进行增强处理。最后,我们将处理结果显示出来。
阅读全文
相关推荐
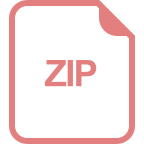
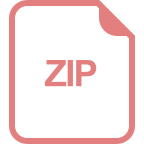
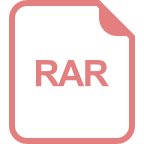













