将这段代码翻译成python语言 private StringBuilder removeSpace(String s) { // System.out.println("ReverseWords.removeSpace() called with: s = [" + s + "]"); int start = 0; int end = s.length() - 1; while (s.charAt(start) == ' ') start++; while (s.charAt(end) == ' ') end--; StringBuilder sb = new StringBuilder(); while (start <= end) { char c = s.charAt(start); if (c != ' ' || sb.charAt(sb.length() - 1) != ' ') { sb.append(c); } start++; } // System.out.println("ReverseWords.removeSpace returned: sb = [" + sb + "]"); return sb; }
时间: 2024-02-14 14:29:10 浏览: 30
Sure! Here's the equivalent Python code for the given Java code:
```python
def removeSpace(s):
start = 0
end = len(s) - 1
while s[start] == ' ':
start += 1
while s[end] == ' ':
end -= 1
sb = []
while start <= end:
c = s[start]
if c != ' ' or sb[-1] != ' ':
sb.append(c)
start += 1
return ''.join(sb)
```
Note that in Python, we use a list (`sb`) instead of a `StringBuilder` to concatenate characters since strings are immutable. Finally, we use the `join` method to convert the list back into a string.
相关问题
Runtime.getRuntime().exec()调用python 并获取python 执行的结果,封装成bean
可以通过Java的ProcessBuilder类来执行Python脚本,并将结果封装成Bean对象。具体步骤如下:
1. 创建一个ProcessBuilder对象,设置要执行的Python命令和参数:
```java
ProcessBuilder pb = new ProcessBuilder("python", "your_python_script.py", "arg1", "arg2");
```
2. 启动进程并等待其完成:
```java
Process process = pb.start();
int exitCode = process.waitFor();
```
3. 读取Python脚本的输出:
```java
BufferedReader reader = new BufferedReader(new InputStreamReader(process.getInputStream()));
String line;
StringBuilder result = new StringBuilder();
while ((line = reader.readLine()) != null) {
result.append(line).append("\n");
}
```
4. 将输出转换为Bean对象:
假设Python脚本的输出为如下JSON格式:
```json
{
"name": "John",
"age": 30
}
```
则可以定义一个对应的Java Bean类:
```java
public class Person {
private String name;
private int age;
// getters and setters
}
```
然后使用Jackson库将JSON字符串转换为Java对象:
```java
ObjectMapper objectMapper = new ObjectMapper();
Person person = objectMapper.readValue(result.toString(), Person.class);
```
完整代码如下:
```java
import com.fasterxml.jackson.databind.ObjectMapper;
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStreamReader;
public class PythonExecutor {
public static void main(String[] args) throws IOException, InterruptedException {
ProcessBuilder pb = new ProcessBuilder("python", "your_python_script.py", "arg1", "arg2");
Process process = pb.start();
int exitCode = process.waitFor();
BufferedReader reader = new BufferedReader(new InputStreamReader(process.getInputStream()));
String line;
StringBuilder result = new StringBuilder();
while ((line = reader.readLine()) != null) {
result.append(line).append("\n");
}
ObjectMapper objectMapper = new ObjectMapper();
Person person = objectMapper.readValue(result.toString(), Person.class);
System.out.println(person.getName());
System.out.println(person.getAge());
}
}
```
AVA实现Python中struct.pack()和struct.unpack()方法
在 Java 中,你可以使用 ByteBuffer 类来实现类似于 Python 中 struct 模块中的 pack 和 unpack 方法的功能。以下是一个示例代码:
```javaimport java.nio.ByteBuffer;
import java.nio.ByteOrder;
public class StructExample {
public static void main(String[] args) {
// 将 int 值打包为字节序列 int intValue =12345;
byte[] packedBytes = packInt(intValue);
System.out.println("Packed bytes: " + bytesToHex(packedBytes));
// 将字节序列解包为 int 值 int unpackedInt = unpackInt(packedBytes);
System.out.println("Unpacked int: " + unpackedInt);
}
private static byte[] packInt(int value) {
ByteBuffer buffer = ByteBuffer.allocate(4);
buffer.order(ByteOrder.LITTLE_ENDIAN); // 设置字节序为小端序列(与Python的struct默认相同)
buffer.putInt(value);
return buffer.array();
}
private static int unpackInt(byte[] bytes) {
ByteBuffer buffer = ByteBuffer.wrap(bytes);
buffer.order(ByteOrder.LITTLE_ENDIAN); // 设置字节序为小端序列 return buffer.getInt();
}
private static String bytesToHex(byte[] bytes) {
StringBuilder sb = new StringBuilder();
for (byte b : bytes) {
sb.append(String.format("%02X", b));
}
return sb.toString();
}
}
```
上述代码演示了 Java 中如何实现类似于 Python 的 struct 模块中的 pack 和 unpack 方法。`packInt` 方法将一个 int 值打包为字节序列,`unpackInt` 方法将字节序列解包为一个 int 值。在这个示例中,我们使用了 ByteBuffer 类来处理字节操作,并通过设置字节序为小端序列来与 Python 的 struct 默认行为保持一致。
相关推荐
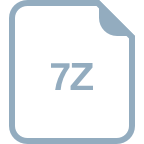
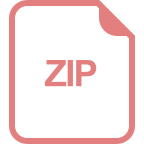
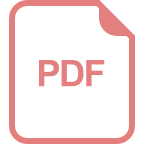












