如何在Python中创建一个加权随机选择函数?
时间: 2024-06-15 14:02:31 浏览: 13
在Python中,你可以使用`random.choices()`函数结合一个字典来实现加权随机选择。这个字典的键是你想要选择的选项,值是对应选项的权重。这里是一个简单的例子:
```python
import random
def weighted_random_choice(weights):
total = sum(weights.values())
r = random.uniform(0, total)
cum_weights = {k: v + r for k, v in weights.items()}
return next(k for k, v in cum_weights.items() if r < v)
# 创建一个字典,其中选项是键,权重是值
options = {
'option1': 0.3,
'option2': 0.5,
'option3': 0.2
}
# 调用函数进行加权随机选择
selected_option = weighted_random_choice(options)
print(selected_option)
```
在这个例子中,`weighted_random_choice`函数首先计算所有权重的总和,然后生成一个0到总和之间的随机数。然后,它遍历字典,累加每个选项的权重,直到达到或超过随机数。最后返回的是第一个满足条件的选项。
相关问题
简单随机加权采样python
以下是一个简单的随机加权采样的Python代码示例:
```python
import random
def weighted_random_sampling(weights, k):
"""
:param weights: A list of non-negative weights.
:param k: The number of samples to draw.
:return: A list of indices indicating the sampled elements.
"""
if k > len(weights):
raise ValueError("k should be no greater than the length of weights.")
cum_weights = [0] + list(accumulate(weights))
total_weight = cum_weights[-1]
indices = []
for _ in range(k):
rand = random.uniform(0, total_weight)
for i, cum_weight in enumerate(cum_weights):
if rand < cum_weight:
indices.append(i - 1)
total_weight -= weights[i - 1]
cum_weights = [0] + list(accumulate(weights[:i - 1])) + [total_weight]
weights = weights[:i - 1] + weights[i:]
break
return indices
```
该函数的输入参数为权重列表和需要采样的数量。输出为一个采样结果的索引列表。
该函数的实现基于累计权重的概念。首先,通过累加所有权重,创建一个新的列表cum_weights。然后,将列表中的每个权重除以总权重,从而获得一个累计权重列表。这个累计权重列表中的每个条目表示前面所有权重的总和。
对于每个采样,生成一个随机数rand,介于0和总权重之间。然后,遍历累计权重列表,找到第一个大于rand的条目(即对应的权重),并将其对应的索引添加到结果列表中。接下来,将该索引对应的权重从权重列表中删除,并更新cum_weights和total_weight。在下一次迭代中,只考虑剩余的权重。
关于加权随机采样,还需要注意以下几点:
- 如果权重中有负数,会引发ValueError异常。
- 如果需要采样的数量k大于权重列表的长度,会引发ValueError异常。
- 由于使用了随机数,因此每次运行该函数都可能得到不同的结果。
用python写一个最简单的单神经网络模型
以下是一个使用Python编写的最简单的单神经网络模型:
```python
import numpy as np
# 定义 sigmoid 函数
def sigmoid(x):
return 1 / (1 + np.exp(-x))
# 定义单神经元
class Neuron:
def __init__(self, input_size):
# 初始化权重和偏置
self.weights = np.random.rand(input_size)
self.bias = np.random.rand(1)
def forward(self, inputs):
# 计算加权和
z = np.dot(inputs, self.weights) + self.bias
# 计算输出
output = sigmoid(z)
return output
# 测试单神经元
if __name__ == '__main__':
# 创建一个单神经元
neuron = Neuron(2)
# 输入数据
inputs = np.array([0.5, 0.7])
# 计算输出
output = neuron.forward(inputs)
# 打印输出
print(output)
```
该模型只有一个神经元,并使用 sigmoid 函数作为激活函数。神经元的输入是一个大小为 2 的向量,其中的权重和偏置是随机初始化的。可以通过调用 `forward` 方法输入数据并计算输出。在本例中,输入数据是 `[0.5, 0.7]`,输出应该是一个介于 0 和 1 之间的数值。
相关推荐
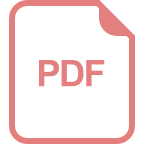
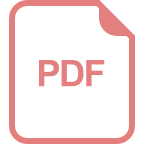












