进程间通信编程 编写一段C语言程序使其完成:父子进程通过无名管道传递三条消息: ·管道文件的测试程序开始 ·管道文件测试正在进行 ·管道通信测试结束 接收进程收到信息后将它们送到标准输出文件上。
时间: 2024-11-05 15:29:00 浏览: 7
在C语言中,可以使用`pipe()`函数创建无名管道来进行进程间通信。下面是一个简单的示例程序,展示了如何创建管道、父进程向管道发送消息以及子进程从管道接收并打印消息:
**父亲进程(发送消息):sender.c**
```c
#include <stdio.h>
#include <unistd.h>
#include <sys/types.h>
#define BUFFER_SIZE 100
int main() {
int pipe_fd[2]; // 创建管道
char message[] = {"管道文件的测试程序开始\n", "管道文件测试正在进行\n", "管道通信测试结束"};
if (pipe(pipe_fd) == -1) {
perror("Pipe failed");
exit(1);
}
pid_t child_pid = fork(); // 创建子进程
if (child_pid == 0) { // 子进程
close(pipe_fd[0]); // 关闭读端
write(pipe_fd[1], message, sizeof(message)); // 向管道写入数据
printf("子进程已关闭写端并写入数据\n");
_exit(0); // 子进程退出
} else { // 父进程
close(pipe_fd[1]); // 关闭写端
char received[BUFFER_SIZE];
read(pipe_fd[0], received, BUFFER_SIZE); // 从管道读取数据
printf("父进程接收到: %s\n", received);
close(pipe_fd[0]); // 关闭读端
wait(NULL); // 等待子进程结束
printf("管道通信结束\n");
}
return 0;
}
```
**儿子进程(接收消息):receiver.c**
```c
#include <stdio.h>
#include <unistd.h>
#include <sys/types.h>
int main() {
int pipe_fd[2];
if (pipe(pipe_fd) == -1) {
perror("Pipe failed");
exit(1);
}
pid_t parent_pid = fork(); // 创建父进程
if (parent_pid == 0) { // 子进程
close(pipe_fd[1]); // 关闭写端
_exit(0); // 子进程退出
} else { // 父进程
close(pipe_fd[0]); // 关闭读端
char received[BUFFER_SIZE];
read(pipe_fd[1], received, BUFFER_SIZE); // 从管道接收数据
printf("父进程已关闭写端并接收数据\n");
write(STDOUT_FILENO, received, strlen(received)); // 将接收到的数据发送到标准输出
_exit(0); // 父进程退出
}
return 0;
}
```
为了运行这个程序,首先分别编译两个源文件(sender.c 和 receiver.c),然后在一个终端中运行发送进程,另一个终端中运行接收进程。每个进程中的一半操作由子进程完成,另一半由父进程完成。
阅读全文
相关推荐
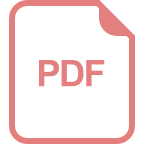
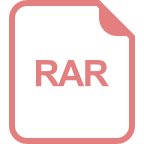
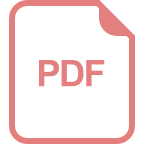
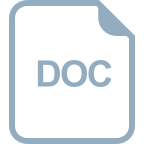
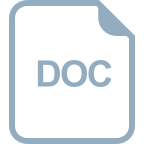
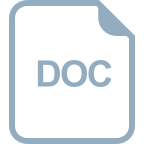
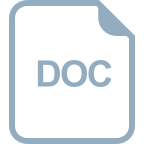
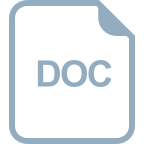
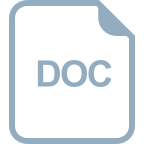
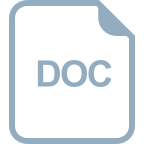
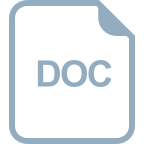
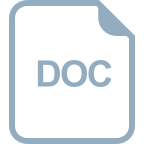
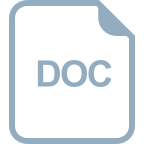
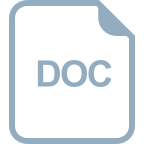
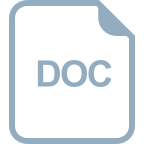
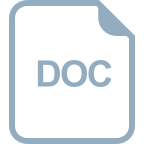
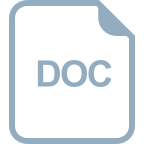
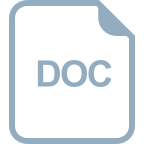
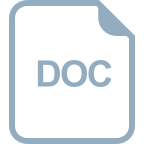