如何用C语言实现正弦函数(sin)的计算?
时间: 2024-12-02 08:21:16 浏览: 60
在C语言中,你可以通过数学库函数`sin()`来直接计算正弦值。这个函数通常位于`math.h`头文件中。下面是一个简单的示例,展示了如何使用它:
```c
#include <stdio.h>
#include <math.h> // 引入 math.h 头文件以使用 sin 函数
int main() {
double angle_in_degrees; // 输入角度 (单位:度)
double result;
printf("请输入角度:");
scanf("%lf", &angle_in_degrees); // 读取用户输入的角度
// 将角度转换为弧度,因为 `sin` 函数期望弧度作为参数
result = sin(dblDegreesToRadians(angle_in_degrees));
printf("正弦值 of %.2f 度 is: %.6f\n", angle_in_degrees, result);
return 0;
}
// 辅助函数将角度从度转换为弧度
double dblDegreesToRadians(double degrees) {
const double PI = M_PI; // 使用 π 的常数
return degrees * (PI / 180.0);
}
```
在这个程序中,我们首先获取用户的输入角度,然后将其转换为弧度,这是因为`sin()`函数需要弧度作为输入。然后我们调用`sin()`函数并打印结果。
相关问题
如何使用C语言实现泰勒级数法来计算正弦函数sin(x)?
使用C语言实现泰勒级数法来计算正弦函数sin(x),通常涉及到编写一个递归或循环结构来逐步近似正弦值。泰勒级数展开式是:
sin(x) = x - (x^3 / 3!) + (x^5 / 5!) - ... + (-1)^(n+1) * (x^(2n+1) / (2n+1)!)
以下是一个简单的C程序示例,展示了如何使用前几项进行计算:
```c
#include <stdio.h>
#include <math.h>
double factorial(int n) {
if (n == 0 || n == 1) return 1;
else return n * factorial(n - 1);
}
double sin_taylor(double x, int terms) {
double sum = x, fact = factorial(3), term = 1.0;
for (int i = 2; i <= terms && abs(term) > 1e-8; ++i) {
term *= -1 * pow(x, 2 * i + 1);
fact /= (2 * i + 1);
sum += term / fact;
}
return sum;
}
int main() {
double x, result;
printf("Enter the value of x: ");
scanf("%lf", &x);
int terms = 10; // 可以调整需要的精度,更多的项会得到更准确的结果
result = sin_taylor(x, terms);
printf("The approximation of sin(%lf) using Taylor series with %d terms is: %lf\n", x, terms, result);
return 0;
}
```
这个程序首先定义了一个计算阶乘的辅助函数,然后在`sin_taylor`函数中迭代地添加泰勒级数的每一项。用户可以输入他们想要计算的`x`值以及希望取多少项来进行近似。
如何用c语言计算正弦函数面积,C语言使用函数调用实现三角形面积的计算
要计算正弦函数面积,需要用到积分的概念。在C语言中,可以通过函数来实现三角形面积的计算,具体如下:
```c
#include <stdio.h>
#include <math.h>
double calc_triangle_area(double a, double b, double c) {
double s = (a + b + c) / 2;
double area = sqrt(s * (s - a) * (s - b) * (s - c));
return area;
}
int main() {
double a, b, c;
printf("请输入三角形三边长: ");
scanf("%lf%lf%lf", &a, &b, &c);
double area = calc_triangle_area(a, b, c);
printf("三角形的面积为: %.2lf\n", area);
return 0;
}
```
要计算正弦函数面积,可以使用数值积分法,例如梯形法。具体实现如下:
```c
#include <stdio.h>
#include <math.h>
double calc_sin_area(double a, double b, int n) {
double h = (b - a) / n;
double sum = (sin(a) + sin(b)) / 2;
for (int i = 1; i < n; i++) {
double x = a + i * h;
sum += sin(x);
}
double area = h * sum;
return area;
}
int main() {
double a, b;
int n;
printf("请输入正弦函数积分区间和梯形数: ");
scanf("%lf%lf%d", &a, &b, &n);
double area = calc_sin_area(a, b, n);
printf("正弦函数在[%.2lf, %.2lf]上的面积为: %.2lf\n", a, b, area);
return 0;
}
```
在上面的代码中,`calc_sin_area`函数用于计算正弦函数在指定区间上的面积,其中`a`和`b`是积分区间,`n`是梯形数,`h`是梯形的宽度,`sum`是梯形面积之和。
阅读全文
相关推荐
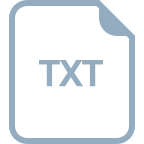
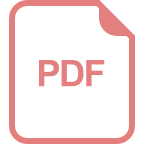
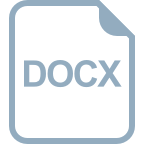
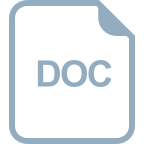






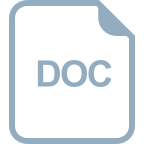
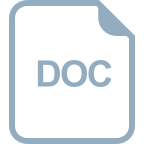




