如何利用泰勒公式精确计算正弦函数 sin(x) 的近似值?C语言
时间: 2024-11-25 11:14:42 浏览: 65
泰勒公式是一种数学工具,用于近似复杂的函数,如正弦函数sin(x),通过将其表示为无穷级数。对于正弦函数,我们可以使用其泰勒级数展开,该级数在x=0处展开:
sin(x) = x - (x^3 / 3!) + (x^5 / 5!) - ... + (-1)^n * (x^(2n+1) / (2n+1)!)
其中n是一个非负整数,3!, 5! 等表示阶乘。
在C语言中,你可以编写一个函数来实现这个过程,例如,你可以设定一个精度阈值ε,然后循环计算并累加级数项,直到满足精度要求。下面是一个简单的示例:
```c
#include <math.h> // 包含fabs函数,用于计算绝对值
double taylor_sin(double x, double epsilon) {
double term = x;
int n = 0;
while (fabs(term) > epsilon) { // 当当前项小于精度阈值时停止迭代
if (term * term < 0) term *= -1; // 如果奇数项,改变符号
term *= x * x / ((2*n + 3) * (2*n + 1)); // 计算下一项
n++;
}
return term;
}
```
使用此函数时,传入需要计算的x值和所需的精度阈值ε。请注意,实际应用中可能需要处理特殊情况,比如x过大导致指数增长的情况。
相关问题
写一个C语言代码,用泰勒公式计算正弦函数在x=0.1时的近似值,展开40项
泰勒公式是一种数学工具,用于通过无穷级数逼近函数在某一点的值。对于正弦函数 \(\sin(x)\),其泰勒级数展开式可以表示为:
\[
\sin(x) = x - \frac{x^3}{3!} + \frac{x^5}{5!} - \frac{x^7}{7!} + \cdots
\]
其中 \(n!\) 表示阶乘,例如 \(3! = 3 \times 2 \times 1\)。
如果你想计算 \(x = 0.1\) 的近似值并取前40项,你可以编写一个C语言程序来实现这个任务。以下是简单的C代码示例:
```c
#include <stdio.h>
double factorial(int n) {
if (n == 0 || n == 1)
return 1;
else
return n * factorial(n - 1);
}
double sin_xaylor(double x, int terms) {
double sum = 0, term;
for (int i = 1; i <= terms; ++i) {
term = pow(-1, (i+1)) * pow(x, 2*i+1) / factorial(2*i+1);
sum += term;
}
return sum;
}
int main() {
double x = 0.1;
int terms = 40;
printf("Using Taylor series approximation with %d terms, \nsin(0.1) ≈ %.8lf\n", terms, sin_xaylor(x, terms));
return 0;
}
```
在这个代码里,我们首先定义了一个递归函数`factorial()`来计算阶乘,然后在`sine_xaylor()`函数中计算正弦函数的泰勒级数,并在主函数中设置\(x = 0.1\)和\(terms = 40\),然后输出结果。
用C语言利用泰勒公式计算sinx近似值
在C语言中,我们可以利用泰勒公式(Taylor Series)来计算正弦函数sin(x)的一个近似值。泰勒公式允许我们将复杂的函数表示为无穷级数的形式,对于像sin(x)这样的周期函数,它特别有用。最简单的一阶近似就是用切线法,但对于更精确的结果,可以采用二阶、三阶等高阶项。
以下是一个简单的例子,展示如何用二阶泰勒公式计算sin(x):
```c
#include <stdio.h>
#include <math.h>
double taylor_sin(double x, int order) {
double sin_x = x;
for (int i = 1; i <= order; i++) {
sin_x += pow(-1, i + 1) * pow(x, 2 * i + 1) / fact(2 * i + 1);
}
return sin_x;
}
// 计算阶乘
unsigned long long fact(int n) {
unsigned long long res = 1;
for (int i = 2; i <= n; ++i)
res *= i;
return res;
}
int main() {
double x_value;
printf("请输入角度(弧度制): ");
scanf("%lf", &x_value);
int order = 2; // 使用二阶泰勒公式
double approx = taylor_sin(x_value, order);
printf("sin(%lf)的二阶泰勒公式近似值为: %lf\n", x_value, approx);
return 0;
}
```
在这个程序中,`taylor_sin` 函数接收输入的角度 `x` 和泰勒展开的阶数 `order`,然后计算并返回sin(x)的近似值。`fact` 函数用于计算阶乘。
请注意,随着阶数 `order` 的增加,泰勒公式将提供更准确的结果,但也需要更多的计算步骤。
阅读全文
相关推荐
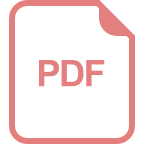
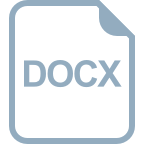
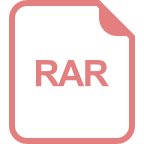













