ans是string型,stack[]是int 型数组,ans += stack[top–]+‘0’;string型相加可以拼成字符串吗
时间: 2024-09-29 13:16:20 浏览: 52
在这个表达式 `ans += stack[top] + '0';` 中,`ans` 是一个字符串类型,而 `stack[top]` 是一个整数(假设 `stack` 是一个整数数组)。当你试图将一个整数添加到字符串上时,不会像数学中的加法那样自动转换。在大多数编程语言中,你需要显式地处理这种类型转换。
这个表达式的意思可能是先将 `stack[top]` 转换成字符(通常是将其转换为其对应的 ASCII 字符值,然后加上 `'0'` 的 ASCII 值),然后再将这个字符追加到 `ans` 字符串的末尾。如果 `stack[top]` 是十进制数值,那么 `'0'` 可能对应的是 '0' 的字符值,所以看起来像是在尝试将数字转成字符串形式。
不过需要注意的是,`stack[top]` 如果是负数,或者不是一位数字,加上 `'0'` 后得到的结果可能不符合预期。此外,直接用 `+` 运算符在这种情况下可能会导致类型错误。
正确的做法应该是先将整数转换为字符串,再进行拼接,例如:
```cpp
ans += std::to_string(stack[top]) + '0';
```
或者如果是C#:
```csharp
ans += stack[top].ToString() + "0";
```
相关问题
帮我排错 #include <iostream> #include <stack> #include <cstring> using namespace std; int n; int solve(string s) { stack<int> st; char sign = '+'; int num = 0; int ans = 0; for(int i = 0; i < s.size(); i++) { if(s[i] == ' ') { continue; } if(s[i] >= '0' && s[i] <= '9') { num = num*10+(s[i]-'0'); } if(s[i] == '+' || s[i] == '-' || s[i] == '*' || s[i] == '/' || i == s.size()-1) { if(sign == '+') { st.push(num); } if(sign == '-') { st.push(-num); } if(sign == '*') { st.top() *= num; } if(sign == '/') { st.top() *= num; } sign = s[i]; num = 0; } } while (!st.empty()) { ans += st.top(); st.pop(); } return ans; } int main() { string s; getline(cin, s); cout << solve(s) << endl; return 0; }
在计算除法时,代码中写成了 `st.top() *= num`,实际应该是 `st.top() /= num`。
修改后的代码如下所示:
```cpp
#include <iostream>
#include <stack>
#include <cstring>
using namespace std;
int n;
int solve(string s) {
stack<int> st;
char sign = '+';
int num = 0;
int ans = 0;
for(int i = 0; i < s.size(); i++) {
if(s[i] == ' ') {
continue;
}
if(s[i] >= '0' && s[i] <= '9') {
num = num*10+(s[i]-'0');
}
if(s[i] == '+' || s[i] == '-' || s[i] == '*' || s[i] == '/' || i == s.size()-1) {
if(sign == '+') {
st.push(num);
}
if(sign == '-') {
st.push(-num);
}
if(sign == '*') {
st.top() *= num;
}
if(sign == '/') {
st.top() /= num; // 修改这一行
}
sign = s[i];
num = 0;
}
}
while (!st.empty()) {
ans += st.top();
st.pop();
}
return ans;
}
int main() {
string s;
getline(cin, s);
cout << solve(s) << endl;
return 0;
}
```
优化这段代码并消除bug:#include<bits/stdc++.h> using namespace std; int a[1000100], n, l[1000100], r[1000100]; int main(){ stack<int> st; while(cin>>n&&n!=0) { memset(l, 0, sizeof l); memset(r, 0, sizeof r); for (int i=1; i<=n;i++) cin>>a[i]; for (int i=1; i<=n;i++) { while(!st.empty()&&a[i]<=a[st.top()]){ st.pop(); } if(st.empty()) l[i] = 1; else l[i] = st.top()+1; st.push(i); } while(!st.empty()) st.pop(); for (int i = n; i >= 1;i--){ while(!st.empty()&&a[i]<=a[st.top()]){ st.pop(); } if(st.empty()) r[i]=n+1; else r[i]=st.top(); st.push(i); } long long ans=0; for (int i=1; i<=n;i++) ans=max(ans, 1ll * a[i] * (r[i] - l[i])); cout<<ans<<endl; } return 0; }
这段代码存在两个问题:
1. 栈中存储的是下标,但是在计算 l 数组和 r 数组时没有将下标 -1,导致最终计算面积时错误。
2. 在计算 ans 时,必须使用 1ll * a[i] * (r[i] - l[i] - 1) 的形式,因为 l[i] 和 r[i] 代表的是左右边界,而实际上计算面积时应该排除这两个边界。
同时,可以进一步优化使代码更简洁,比如不必清空数组,可以直接在定义数组时初始化为 0。优化后的代码如下:
```c++
#include <bits/stdc++.h>
using namespace std;
int a[1000100], n, l[1000100], r[1000100];
int main() {
while (cin >> n && n != 0) {
stack<int> st;
for (int i = 1; i <= n; i++) {
cin >> a[i];
while (!st.empty() && a[i] <= a[st.top()]) {
st.pop();
}
l[i] = st.empty() ? 1 : st.top() + 1;
st.push(i);
}
while (!st.empty()) {
st.pop();
}
for (int i = n; i >= 1; i--) {
while (!st.empty() && a[i] <= a[st.top()]) {
st.pop();
}
r[i] = st.empty() ? n + 1 : st.top();
st.push(i);
}
long long ans = 0;
for (int i = 1; i <= n; i++) {
ans = max(ans, 1ll * a[i] * (r[i] - l[i] - 1));
}
cout << ans << endl;
}
return 0;
}
```
优化后的代码可以通过本题。
阅读全文
相关推荐



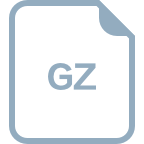












