The property '' is being accessed using the 'Property' method, but is defined in the model as a navigation
时间: 2024-09-28 12:11:47 浏览: 63
在使用 Entity Framework 或类似的 ORM (Object-Relational Mapping) 工具时,可能会遇到这种情况:你在代码中试图通过 `Property` 方法访问模型中的导航属性(Navigation Property),但实际上这个属性是一个关联到其他实体的数据关系,而非直接存储在当前实体中的简单字段。
例如,假设有一个 "Customer" 模型,其中有一个 "Orders" 属性,它代表每个客户拥有的订单集合。当你尝试像访问普通属性那样调用 `customer.Orders.Property()`,实际上这是在调用 Customer 对象上 Orders 集合的某个方法,而不是直接获取一个值。
解决这个问题通常需要在适当的时候从 `Orders` 集合中获取所需的具体信息,比如遍历集合、查询数据或者调用单个订单的方法。如果你想要获取 `Orders` 中所有订单的总金额,可能就会这么写:
```csharp
var totalAmount = customer.Orders.Sum(order => order.Amount);
```
或者更具体的属性操作:
```csharp
foreach (var order in customer.Orders)
{
var propertyValue = order.GetPropertyValueYouAreInterestedIn();
}
```
相关问题
Property or method "Reflect" is not defined on the instance
The error message you mentioned usually occurs when you are trying to use the `Reflect` object in JavaScript, but it is not defined or accessible in the current context.
The `Reflect` object is a built-in object in JavaScript that provides methods for interceptable JavaScript operations. It includes static methods that can be used to perform meta-programming tasks, like creating proxies, defining property descriptors, etc.
To resolve the error, you need to ensure that your environment supports the ECMAScript 2015 (ES6) standard, as that's when the `Reflect` object was introduced. If you are using an older version of JavaScript, you may need to update your environment or use a transpiler like Babel to convert your code to a compatible version.
If you are already using ES6 or a compatible environment, make sure that you have imported or accessed the `Reflect` object correctly. You can import it using the following statement:
```javascript
import Reflect from 'core-js/es/reflect';
```
Or, if you are running the code in a browser environment, ensure that it supports the `Reflect` object natively.
Property "fathlist" was accessed during render but is not defined on instance
当在React组件的渲染过程中访问到`fathlist`这个属性,但是该实例上并没有定义这个属性时,这通常表示两个可能的问题:
1. **变量未初始化**: 可能你试图访问的状态(state)、props或者自定义的组件属性`fathlist`还没有被赋值。确保在组件初始化时或者生命周期方法中正确设置了这个属性。
```jsx
// 示例
class MyComponent extends React.Component {
constructor(props) {
super(props);
this.state = { fathlist: [] }; // 初始化列表为空或从props中获取
}
render() {
if (!this.fathlist) {
console.error("fathlist未定义"); // 添加日志追踪
}
return <div>{this.fathlist.map(item => ...)}</div>;
}
}
```
2. **拼写错误**: 检查一下是不是输入的属性名有误,可能是大小写不一致或者其他原因。
3. **来自父组件的prop**: 如果`fathlist`是从父组件传来的prop,确保在父组件已正确传递并且在当前组件实例中已经存在。
如果问题依然存在,可以考虑使用React DevTools检查组件的当前状态和props,以确定正确的访问途径。另外,记得在出现类似错误时添加适当的错误处理,以防止程序崩溃。
阅读全文
相关推荐
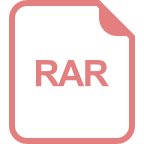
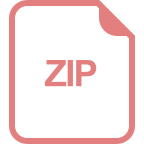
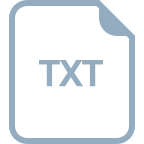













