C语言利用malloc函数开辟动态储存单元存放入三个学生信息然后输出这三个学生的信息
时间: 2024-09-29 15:08:18 浏览: 35
在C语言中,可以使用`malloc()`函数动态地分配内存用于存储结构体,比如学生信息。首先,我们需要定义一个包含学生信息的结构体,例如:
```c
struct Student {
char name[50];
int age;
float score;
};
```
接着,我们使用`malloc()`分配内存,并创建三个这样的结构体实例:
```c
struct Student* students = malloc(3 * sizeof(struct Student)); // 分配空间给三个学生
if (students == NULL) {
printf("Memory allocation failed.\n");
return; // 如果分配失败,处理错误
}
// 为每个学生填充数据
students[0].name = "张三";
students[0].age = 20;
students[0].score = 90.0;
students[1].name = "李四";
students[1].age = 22;
students[1].score = 85.0;
students[2].name = "王五";
students[2].age = 19;
students[2].score = 95.0;
```
最后,我们可以遍历这个结构数组,打印出每个学生的信息:
```c
for (int i = 0; i < 3; i++) {
printf("学生%d: 名字:%s, 年龄:%d, 成绩:%f\n", i + 1, students[i].name, students[i].age, students[i].score);
}
```
记得在程序结束前释放分配的内存,防止内存泄漏:
```c
free(students); // 释放内存
```
阅读全文
相关推荐
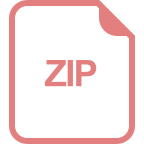
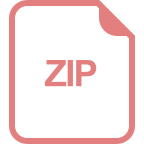
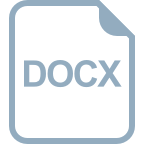
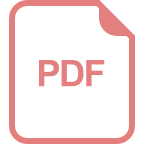
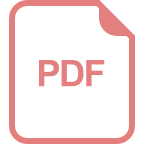
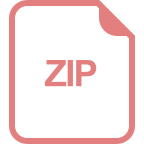
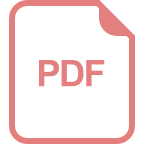
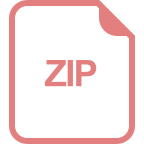
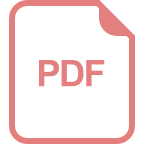
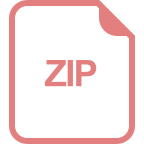
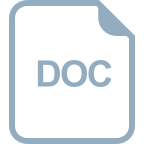
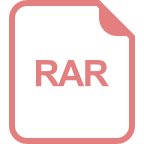
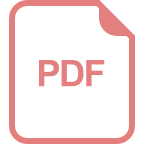
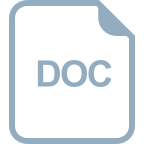
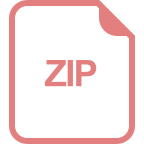
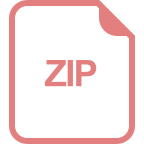