编写Java+Application,求出e=1+1+\div+1!+1+\div+2!+1+\div+3!++\dotsc+1/n!+\dotsc的近似值,要求误差小于0.00001。
时间: 2023-10-10 10:08:32 浏览: 51
首先,我们可以使用以下代码在Java中计算e的近似值,直到误差小于0.00001为止:
```java
public class CalculateE {
public static void main(String[] args) {
double e = 1.0;
double term = 1.0;
int n = 1;
while (term >= 0.00001) {
term = term / n;
e += term;
n++;
}
System.out.println("e的近似值为: " + e);
}
}
```
这段代码使用了一个循环来计算每个项的值,并将其累加到e中,直到最后一个项小于0.00001为止。输出的结果将是e的近似值。
回答完问题后,这里是您要求的相关问题:
相关问题:
1. 如何在Java中计算阶乘?
. 如何在Java中计算指数值?
3. 如何在Java中处理浮点数的精度问题?
4. 如何使用递归来计算e的近似值?
5. 如何优化计算e的近似值的性能?
相关问题
帮我用java+vue实现一个简单的图书管理系统
A:
首先,你可以使用Java编写后端,使用Vue编写前端。下面是一个示例:
1. 后端代码:
```
package com.example.library;
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
@SpringBootApplication
public class LibraryApplication {
public static void main(String[] args) {
SpringApplication.run(LibraryApplication.class, args);
}
}
```
```
package com.example.library.controller;
import com.example.library.entity.Book;
import com.example.library.service.BookService;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.http.HttpStatus;
import org.springframework.http.ResponseEntity;
import org.springframework.web.bind.annotation.*;
import java.util.List;
@RestController
@RequestMapping("/api/v1/books")
public class BookController {
@Autowired
private BookService bookService;
@GetMapping
public ResponseEntity<List<Book>> getBooks() {
List<Book> books = bookService.getBooks();
return new ResponseEntity<>(books, HttpStatus.OK);
}
@PostMapping
public ResponseEntity<Book> addBook(@RequestBody Book book) {
Book addedBook = bookService.addBook(book);
return new ResponseEntity<>(addedBook, HttpStatus.CREATED);
}
@PutMapping("/{id}")
public ResponseEntity<Book> updateBook(@PathVariable Long id, @RequestBody Book book) {
Book updatedBook = bookService.updateBook(id, book);
return new ResponseEntity<>(updatedBook, HttpStatus.OK);
}
@DeleteMapping("/{id}")
public ResponseEntity<Void> deleteBook(@PathVariable Long id) {
bookService.deleteBook(id);
return new ResponseEntity<>(HttpStatus.NO_CONTENT);
}
}
```
```
package com.example.library.entity;
import lombok.AllArgsConstructor;
import lombok.Data;
import lombok.NoArgsConstructor;
import javax.persistence.*;
@Data
@AllArgsConstructor
@NoArgsConstructor
@Entity
@Table(name = "books")
public class Book {
@Id
@GeneratedValue(strategy = GenerationType.AUTO)
private Long id;
private String title;
private String author;
private String publisher;
}
```
```
package com.example.library.repository;
import com.example.library.entity.Book;
import org.springframework.data.jpa.repository.JpaRepository;
import java.util.Optional;
public interface BookRepository extends JpaRepository<Book, Long> {
Optional<Book> findById(Long id);
}
```
```
package com.example.library.service;
import com.example.library.entity.Book;
import com.example.library.exception.ResourceNotFoundException;
import com.example.library.repository.BookRepository;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Service;
import java.util.List;
@Service
public class BookService {
@Autowired
private BookRepository bookRepository;
public List<Book> getBooks() {
return bookRepository.findAll();
}
public Book addBook(Book book) {
return bookRepository.save(book);
}
public Book updateBook(Long id, Book newBook) {
Book oldBook = bookRepository.findById(id).orElseThrow(() -> new ResourceNotFoundException("Book not found"));
oldBook.setTitle(newBook.getTitle());
oldBook.setAuthor(newBook.getAuthor());
oldBook.setPublisher(newBook.getPublisher());
return bookRepository.save(oldBook);
}
public void deleteBook(Long id) {
bookRepository.deleteById(id);
}
}
```
2. 前端代码:
```
<template>
<div>
<table>
<thead>
<tr>
<th>ID</th>
<th>Title</th>
<th>Author</th>
<th>Publisher</th>
<th>Actions</th>
</tr>
</thead>
<tbody>
<tr v-for="book in books" :key="book.id">
<td>{{ book.id }}</td>
<td>{{ book.title }}</td>
<td>{{ book.author }}</td>
<td>{{ book.publisher }}</td>
<td>
<button @click="editBook(book)">Edit</button>
<button @click="deleteBook(book.id)">Delete</button>
</td>
</tr>
</tbody>
</table>
<div v-if="showForm">
<h2 v-if="!editMode">Add book</h2>
<h2 v-if="editMode">Edit book</h2>
<form @submit.prevent="submitForm">
<label>Title:</label>
<input type="text" v-model="book.title" required>
<label>Author:</label>
<input type="text" v-model="book.author" required>
<label>Publisher:</label>
<input type="text" v-model="book.publisher" required>
<button type="submit">{{ editMode ? 'Save changes' : 'Add' }}</button>
</form>
</div>
<button @click="showForm = true">Add book</button>
</div>
</template>
<script>
import axios from 'axios';
export default {
data() {
return {
books: [],
showForm: false,
editMode: false,
book: {
title: '',
author: '',
publisher: '',
},
};
},
created() {
this.getBooks();
},
methods: {
async getBooks() {
const { data } = await axios.get('/api/v1/books');
this.books = data;
},
async addBook(book) {
await axios.post('/api/v1/books', book);
this.getBooks();
},
async updateBook(id, book) {
await axios.put(`/api/v1/books/${id}`, book);
this.getBooks();
},
async deleteBook(id) {
await axios.delete(`/api/v1/books/${id}`);
this.getBooks();
},
editBook(book) {
this.editMode = true;
this.showForm = true;
this.book = { ...book };
},
async submitForm() {
if (this.editMode) {
await this.updateBook(this.book.id, this.book);
} else {
await this.addBook(this.book);
}
this.showForm = false;
this.editMode = false;
this.book = {
title: '',
author: '',
publisher: '',
};
},
},
};
</script>
```
请注意,这只是一个简单的示例,可以根据您的需要进行更改和修改。您还需要相应配置您的数据库和后端框架以进行完整的实现。
使用springboot+mybatis+thymeleaf编写代码实现分页查询
首先,需要在pom.xml中引入相应的依赖,这里以MySQL数据库为例:
```xml
<!-- SpringBoot Web模块 -->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<!-- SpringBoot Mybatis模块 -->
<dependency>
<groupId>org.mybatis.spring.boot</groupId>
<artifactId>mybatis-spring-boot-starter</artifactId>
<version>2.2.0</version>
</dependency>
<!-- MySQL驱动 -->
<dependency>
<groupId>mysql</groupId>
<artifactId>mysql-connector-java</artifactId>
<version>8.0.23</version>
</dependency>
```
其次,需要配置Mybatis和数据库连接,可以在application.yml中配置:
```yml
spring:
datasource:
driver-class-name: com.mysql.cj.jdbc.Driver
url: jdbc:mysql://localhost:3306/testdb?serverTimezone=UTC&useSSL=false&characterEncoding=utf8
username: root
password: root
mybatis:
config-location: classpath:mybatis-config.xml
mapper-locations: classpath:mapper/*.xml
```
其中,`datasource`为数据源的基本信息,`mybatis`则是Mybatis的配置信息,包括配置文件的位置和Mapper文件的位置。
接下来,需要编写Mapper接口和对应的Mapper XML文件。以User表为例:
```java
public interface UserMapper {
List<User> findUserByPage(@Param("start") Integer start, @Param("pageSize") Integer pageSize);
}
```
```xml
<mapper namespace="com.example.demo.mapper.UserMapper">
<select id="findUserByPage" resultType="com.example.demo.entity.User">
select * from user limit #{start},#{pageSize}
</select>
</mapper>
```
其中,`findUserByPage`方法为分页查询方法,`start`为起始位置,`pageSize`为每页数量。
最后,编写Controller层和前端页面。以UserController为例:
```java
@Controller
public class UserController {
@Autowired
private UserMapper userMapper;
@GetMapping("/user")
public String findUserByPage(@RequestParam(value = "pageNum", defaultValue = "1") Integer pageNum,
@RequestParam(value = "pageSize", defaultValue = "5") Integer pageSize,
Model model) {
Integer start = (pageNum - 1) * pageSize;
List<User> userList = userMapper.findUserByPage(start, pageSize);
PageInfo pageInfo = new PageInfo(userList);
model.addAttribute("pageInfo", pageInfo);
return "user";
}
}
```
其中,`findUserByPage`方法接收两个参数:`pageNum`和`pageSize`,表示当前页和每页数量。通过计算获得起始位置,调用Mapper接口进行分页查询,并通过`PageInfo`类将查询结果传递给前端页面。
在前端页面中通过`th:each`循环遍历查询结果,并通过`th:href`生成分页链接。以user.html为例:
```html
<!DOCTYPE html>
<html lang="en" xmlns:th="http://www.thymeleaf.org">
<head>
<meta charset="UTF-8">
<title>User List</title>
</head>
<body>
<table border="1">
<thead>
<tr>
<th>ID</th>
<th>Name</th>
<th>Age</th>
<th>Gender</th>
</tr>
</thead>
<tbody>
<tr th:each="user : ${pageInfo.list}">
<td th:text="${user.id}"></td>
<td th:text="${user.name}"></td>
<td th:text="${user.age}"></td>
<td th:text="${user.gender}"></td>
</tr>
</tbody>
</table>
<div>
<a th:href="@{/user?pageNum=1}">首页</a>
<a th:href="@{/user?pageNum=${pageInfo.prePage}}">上一页</a>
<a th:href="@{/user?pageNum=${pageInfo.nextPage}}">下一页</a>
<a th:href="@{/user?pageNum=${pageInfo.pages}}">尾页</a>
</div>
</body>
</html>
```
其中,`pageInfo.list`为查询结果列表,通过`th:each`循环遍历生成表格数据。底部的分页链接则通过`th:href`生成相应的链接。
到这里,一个简单的分页查询就完成了。需要注意的是,以上代码仅为示例,具体实现方式可能会有所不同,需要按照实际需求进行调整。
相关推荐
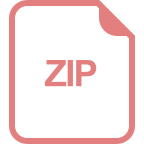
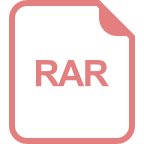
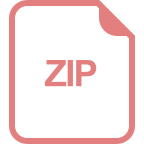













