shareingjdbc 读写分离 druid 不能批量更新
时间: 2023-09-06 19:02:40 浏览: 157
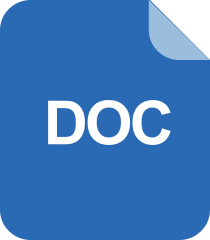
JDBC批量插入 更新 删除等操作

SharingJDBC 是一个用于读写分离的 Java 数据库中间件,可以通过将读操作和写操作分别路由到不同的数据库节点,以提高数据库读写能力和性能。
Druid 是一个开源的数据库连接池组件,它提供了连接池管理、SQL 解析、SQL 优化、SQL 状态监控和统计以及批量处理等功能。然而,Druid 在进行批量更新操作时存在一定的限制。
具体而言,Druid 的批量更新操作是通过 JDBC 的批处理机制来实现的,即将一批更新操作打包成一个批次进行执行。但是,由于数据库的不同实现,对批量更新的支持程度各不相同。有些数据库如 MySQL 对批量更新的支持非常好,而有些数据库如 Oracle 的支持则相对较弱。
因此,由于数据库的差异性,Druid 在进行批量更新操作时可能会遇到一些限制,特别是在某些数据库环境下。在这种情况下,即使使用了 SharingJDBC 进行读写分离,也不能解决 Druid 本身的批量更新限制。
为解决这个问题,我们可以考虑以下几种方案:
1. 如果数据库支持批量更新操作,可以尝试使用数据库的原生批量更新功能,而不是依赖于 Druid 的批量处理机制。
2. 对于不支持或支持较弱的数据库,可以通过自行编写代码,将多次更新操作组织在同一个事务中,以减少数据库的交互次数,并提高性能。
3. 如果对批量更新操作的性能要求不高,也可以将更新操作拆分成多个单个更新操作,逐一执行。
总之,虽然 Druid 在某些数据库环境下可能不能进行高效的批量更新操作,但我们可以通过其他手段来解决这个问题,以提高系统的性能和可靠性。
阅读全文
相关推荐
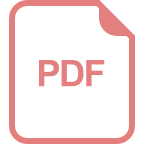
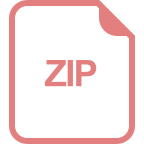
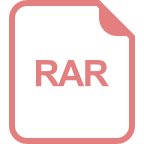
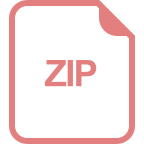
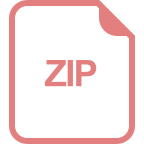
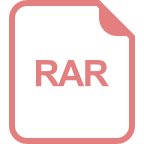
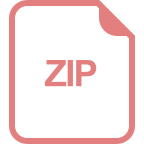
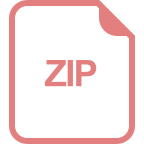
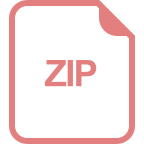
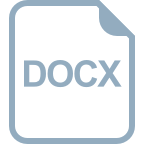
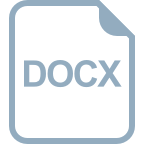
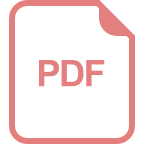
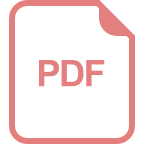
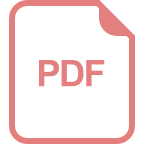
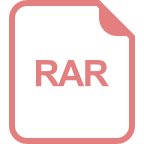
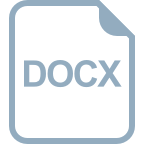