建立一个顺序链表,实现对顺序表的初始化,判空,求顺序表的长度,插入元素,查找和删除功能
时间: 2024-11-09 08:27:55 浏览: 7
在C++中,我们可以使用结构体(struct)来表示顺序链表,它由一系列的节点组成,每个节点包含一个元素值和指向下一个节点的指针。下面是一个基本的顺序链表实现,包括初始化、判空、获取长度、插入、查找和删除等操作:
```cpp
#include <iostream>
// 定义节点结构
struct Node {
int data;
Node* next; // 指向下一个节点
};
class LinkedList {
private:
Node* head; // 链表头部
public:
// 初始化链表为空
LinkedList() : head(nullptr) {}
// 判断链表是否为空
bool isEmpty() const {
return head == nullptr;
}
// 获取链表长度
int length() const {
int count = 0;
Node* current = head;
while (current != nullptr) {
++count;
current = current->next;
}
return count;
}
// 插入元素到链表末尾
void insert(int value) {
Node* newNode = new Node();
newNode->data = value;
newNode->next = nullptr;
if (isEmpty()) {
head = newNode;
} else {
Node* current = head;
while (current->next != nullptr) {
current = current->next;
}
current->next = newNode;
}
}
// 查找元素
int find(int key) const {
Node* current = head;
int index = 1;
while (current != nullptr && current->data != key) {
current = current->next;
++index;
}
if (current == nullptr) {
return -1; // 元素未找到
}
return index;
}
// 删除元素(仅支持通过值删除)
void remove(int value) {
if (isEmpty()) {
return;
}
if (head->data == value) {
Node* temp = head;
head = head->next;
delete temp;
return;
}
Node* current = head;
Node* previous = nullptr;
while (current != nullptr && current->data != value) {
previous = current;
current = current->next;
}
if (current == nullptr) {
return; // 元素未找到
}
previous->next = current->next;
delete current;
}
// 打印链表
void display() const {
Node* temp = head;
while (temp != nullptr) {
std::cout << temp->data << " ";
temp = temp->next;
}
std::cout << "\n";
}
};
int main() {
LinkedList list;
list.insert(1); // 添加元素
list.insert(2);
list.insert(3);
list.display(); // 显示链表内容
// 测试查找和删除
std::cout << "Element 2 is found at position: " << list.find(2) << std::endl;
list.remove(2); // 删除元素
list.display();
return 0;
}
```
以上代码实现了顺序链表的基本功能,但是请注意,这个版本的链表只支持按值删除,如果需要按位置删除,可以考虑修改`remove`函数。
阅读全文
相关推荐
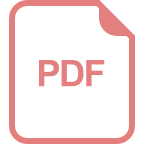
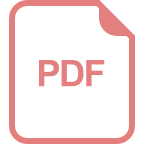
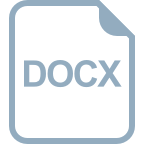

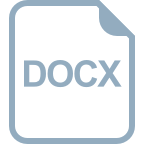
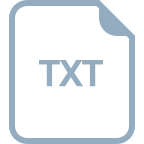
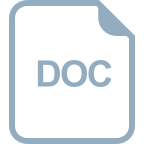
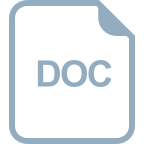
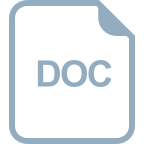
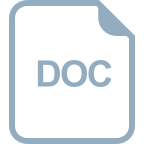
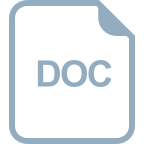
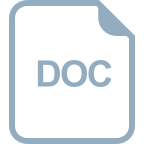
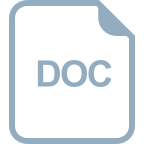



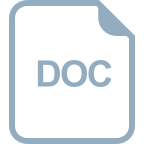
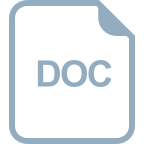