建立一个顺序链表,实现对顺序表的初始化,判空,求顺序表的长度,插入新元素,查找和删除功能
时间: 2024-11-09 21:28:36 浏览: 19
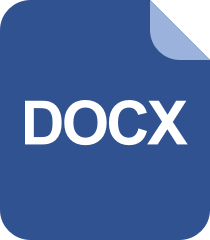
顺序表输入、查找、插入、删除

创建一个顺序链表通常会包含以下几个步骤:
1. **初始化** (构造函数):
初始化一个顺序链表时,可以设置一个头节点(通常是`nullptr`),表示链表为空。例如,在C++中:
```cpp
class ListNode {
public:
int data;
ListNode* next;
// 构造函数
ListNode(int value = 0, ListNode* nextNode = nullptr) : data(value), next(nextNode) {}
};
ListNode* createList() {
return new ListNode(); // 如果不需要初始值,传入nullptr即可
}
```
2. **判断链表是否为空**:
判断一个链表是否为空,就是检查头节点是否为`nullptr`。
```cpp
bool isEmpty(ListNode* head) {
return head == nullptr;
}
```
3. **求链表长度**:
需遍历整个链表计算节点数。从头节点开始,每经过一个节点计数加一。
```cpp
int length(ListNode* head) {
int count = 0;
while (head != nullptr) {
++count;
head = head->next;
}
return count;
}
```
4. **插入新元素**:
可以通过设置新节点的`next`指向现有节点并更新头节点指针来实现插入。
```cpp
void insertAtEnd(ListNode*& head, int value) {
if (isEmpty(head)) {
head = new ListNode(value);
} else {
ListNode* newNode = new ListNode(value);
ListNode* current = head;
while (current->next != nullptr) {
current = current->next;
}
current->next = newNode;
}
}
```
5. **查找元素**:
从头节点开始逐个比较,直到找到匹配项或遍历完整个链表。
```cpp
ListNode* search(ListNode* head, int value) {
while (head != nullptr && head->data != value) {
head = head->next;
}
return head;
}
```
6. **删除元素**:
删除指定值的节点,需要找到该节点的前一个节点,然后将其`next`指向当前节点的下一个节点。
```cpp
void removeElement(ListNode*& head, int value) {
if (isEmpty(head) || head->data == value) {
delete head;
head = nullptr; // 或者头节点就是我们要删除的那个节点
return;
}
ListNode* current = head;
while (current->next != nullptr && current->next->data != value) {
current = current->next;
}
if (current->next != nullptr) {
delete current->next;
current->next = nullptr;
}
}
```
阅读全文
相关推荐
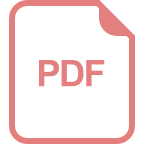
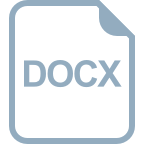
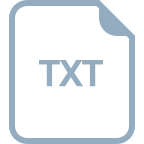
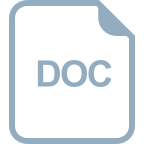




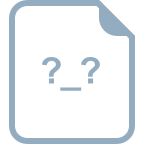
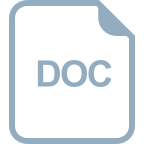
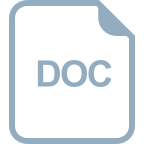
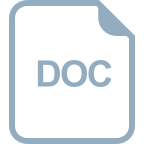
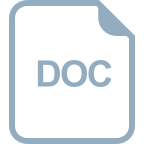
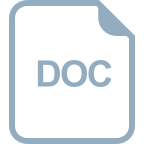
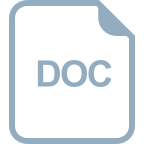
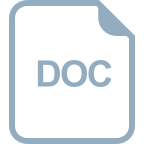

