react使用react redux的过程代码
时间: 2024-06-05 13:03:47 浏览: 19
React Redux 是 React 的一个状态管理库,它可以帮助开发者更好地组织和管理 React 应用的状态。使用 React Redux 的过程如下:
1. 安装 React Redux
```
npm install react-redux
```
2. 创建 store
在应用的入口文件中,我们需要创建一个 Redux store。这个 store 将会保存应用的所有状态。
```javascript
import { createStore } from 'redux';
import rootReducer from './reducers';
const store = createStore(rootReducer);
```
3. 创建 reducers
reducers 指的是一个纯函数,它接收旧的 state 和一个 action,然后返回新的 state。这个过程被称为“reducing”。
```javascript
const initialState = {
count: 0
};
function rootReducer(state = initialState, action) {
switch (action.type) {
case 'INCREMENT':
return {
count: state.count + 1
};
case 'DECREMENT':
return {
count: state.count - 1
};
default:
return state;
}
}
export default rootReducer;
```
4. 将 store 注入到应用中
```javascript
import React from 'react';
import ReactDOM from 'react-dom';
import { Provider } from 'react-redux';
import store from './store';
import App from './App';
ReactDOM.render(
<Provider store={store}>
<App />
</Provider>,
document.getElementById('root')
);
```
5. 使用 connect 连接 React 和 Redux
```javascript
import { connect } from 'react-redux';
function Counter(props) {
return (
<div>
<h1>{props.count}</h1>
<button onClick={props.increment}>+</button>
<button onClick={props.decrement}>-</button>
</div>
);
}
function mapStateToProps(state) {
return {
count: state.count
};
}
function mapDispatchToProps(dispatch) {
return {
increment: () => dispatch({ type: 'INCREMENT' }),
decrement: () => dispatch({ type: 'DECREMENT' })
};
}
export default connect(mapStateToProps, mapDispatchToProps)(Counter);
```
6. 在组件中使用 Redux
现在我们可以在组件中使用 Redux 的状态和操作了。
```javascript
import React from 'react';
import Counter from './Counter';
function App() {
return (
<div>
<Counter />
</div>
);
}
export default App;
```
相关推荐
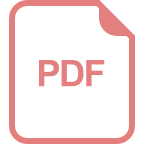
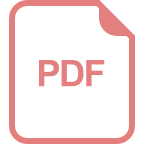
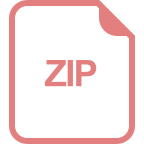














