react 的redux使用方法
时间: 2023-12-25 21:54:34 浏览: 37
1. 安装redux和react-redux
```
npm install redux react-redux
```
2. 创建一个Redux store
```
import { createStore } from 'redux';
import rootReducer from './reducers';
const store = createStore(rootReducer);
```
3. 创建reducer
```
const initialState = {
count: 0
};
function rootReducer(state = initialState, action) {
switch (action.type) {
case 'INCREMENT':
return { count: state.count + 1 };
case 'DECREMENT':
return { count: state.count - 1 };
default:
return state;
}
}
```
4. 创建action
```
function increment() {
return { type: 'INCREMENT' };
}
function decrement() {
return { type: 'DECREMENT' };
}
```
5. 创建一个React组件并连接到Redux store
```
import { connect } from 'react-redux';
function Counter(props) {
return (
<div>
<h2>Count: {props.count}</h2>
<button onClick={props.increment}>+</button>
<button onClick={props.decrement}>-</button>
</div>
);
}
function mapStateToProps(state) {
return {
count: state.count
};
}
function mapDispatchToProps(dispatch) {
return {
increment: () => dispatch(increment()),
decrement: () => dispatch(decrement())
};
}
export default connect(mapStateToProps, mapDispatchToProps)(Counter);
```
6. 在应用中使用组件
```
import React from 'react';
import ReactDOM from 'react-dom';
import { Provider } from 'react-redux';
import Counter from './Counter';
import store from './store';
ReactDOM.render(
<Provider store={store}>
<Counter />
</Provider>,
document.getElementById('root')
);
```
相关推荐
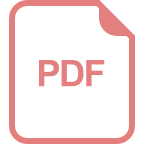
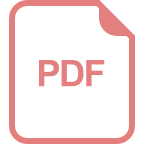














