python判断html标签是否存在
时间: 2024-09-22 17:10:27 浏览: 52
在Python中,可以使用BeautifulSoup库来解析HTML文档并检查特定标签是否存在。BeautifulSoup是一个流行的HTML和XML处理库,它能方便地遍历和查找元素。下面是一个简单的例子:
```python
from bs4 import BeautifulSoup
def check_html_tag(html_content, tag_name):
soup = BeautifulSoup(html_content, 'html.parser')
# 检查tag_name是否存在于页面中
if soup.find(tag_name):
return True
else:
return False
# 使用示例
html = '<html><body><h1>Hello, World!</h1></body></html>'
if check_html_tag(html, 'h1'):
print("存在'h1'标签")
else:
print("'h1'标签不存在")
相关问题
python解析html标签
在Python中,你可以使用多种库来解析HTML标签。其中,比较常用的是Beautiful Soup和lxml库。
首先,你需要安装这些库。你可以使用以下命令来安装它们:
```
pip install beautifulsoup4
pip install lxml
```
接下来,你可以使用Beautiful Soup来解析HTML标签。下面是一个简单的例子:
```python
from bs4 import BeautifulSoup
# 假设你有一个HTML文档,可以从文件中读取或者直接使用字符串
html_doc = """
<html>
<head>
<title>示例网页</title>
</head>
<body>
<h1>标题</h1>
<p>这是一个示例网页。</p>
<a href="http://www.example.com">链接</a>
</body>
</html>
"""
# 创建Beautiful Soup对象
soup = BeautifulSoup(html_doc, 'lxml')
# 获取标题标签的内容
title = soup.title.string
print("标题:", title)
# 获取第一个段落标签的内容
paragraph = soup.p.string
print("段落:", paragraph)
# 获取链接标签的URL
link = soup.a['href']
print("链接:", link)
```
上述代码使用Beautiful Soup解析HTML标签,并提取了标题、段落和链接的内容。你可以根据需要进行进一步的解析和处理。
另外,如果你对性能要求较高,可以考虑使用lxml库。它也是一个强大的HTML解析库,使用方法与Beautiful Soup类似。
希望能对你有所帮助!如果还有其他问题,请随时提问。
python读取html文件
要读取 HTML 文件,可以使用 Python 内置的 `open()` 函数打开文件,然后使用文件对象的 `read()` 方法读取文件内容。例如:
```python
with open('example.html', 'r') as f:
html_content = f.read()
```
这样就可以将 `example.html` 文件的内容读取到 `html_content` 变量中。接下来你可以使用第三方库(如 `beautifulsoup4` 或 `lxml`)解析 HTML 内容,或者使用 Python 自带的 `html.parser` 模块进行解析。这里以 `html.parser` 为例:
```python
from html.parser import HTMLParser
class MyHTMLParser(HTMLParser):
def handle_starttag(self, tag, attrs):
print("Encountered a start tag:", tag)
def handle_endtag(self, tag):
print("Encountered an end tag :", tag)
def handle_data(self, data):
print("Encountered some data :", data)
parser = MyHTMLParser()
parser.feed(html_content)
```
这个例子中,我们继承了 `HTMLParser` 类,并覆盖了其三个方法,用于处理 HTML 中的起始标签、结束标签和数据。`parser.feed(html_content)` 会将解析过程应用到 `html_content` 变量中的 HTML 内容上,并输出解析结果。
阅读全文
相关推荐
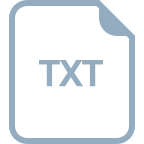
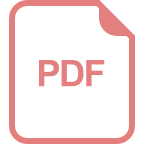
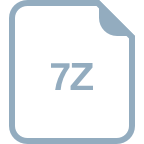













