首页 contours[:, :, 0] = contours[:, :, 0] * factor
TypeError: tuple indices must be integers or slices, not tuple
contours[:, :, 0] = contours[:, :, 0] * factor TypeError: tuple indices must be integers or slices, not tuple
时间: 2023-07-23 10:01:07 浏览: 101
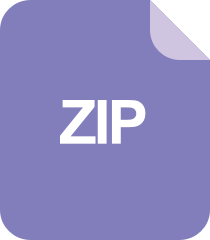
svg-path-contours:从svg获取离散点列表
svg-path-contours 将SVG路径近似为2D轮廓(折线)的离散列表。 这对于碰撞检测,相交,三角剖分和WebGL渲染等很有用。它不会尝试清理或优化离散点。 用法: var parse = require ( 'parse-svg-path' ) var contours = require ( 'svg-path-contours' ) var path = 'm10,10C45.812,24.024,45.673,24,45.529,24H31.625 c0.482-3.325,6.464-2.758,8.913-3.155z' var result = contours ( parse ( path ) ) /* [ [ [10,10], ...etc ] [ [x, y], ... ] ] */ 三角剖分示例: var parse = requi
这个错误是由于在对`contours`进行索引时,使用了元组`( :, :, 0)`作为索引。然而,元组索引在NumPy中是不被允许的,因为它期望整数或切片作为索引。
如果你想要将`contours`中的数据的第一个维度乘以`factor`,你可以使用以下方式来实现:
```python
contours[:, :, 0] *= factor
```
这样会将`contours`中的第一维度的所有元素乘以`factor`。请确保`factor`是一个数值类型的变量,并且与`contours[:, :, 0]`的形状相匹配。
阅读全文
相关推荐
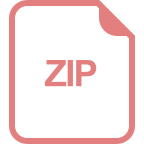
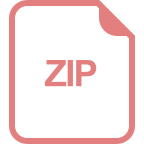
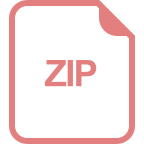
CSDN会员
开通CSDN年卡参与万元壕礼抽奖
最新推荐
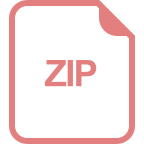
Windows平台下的Fastboot工具使用指南
资源摘要信息:"Windows Fastboot.zip是一个包含了Windows环境下使用的Fastboot工具的压缩文件。Fastboot是一种在Android设备上使用的诊断和工程工具,它允许用户通过USB连接在设备的bootloader模式下与设备通信,从而可以对设备进行刷机、解锁bootloader、安装恢复模式等多种操作。该工具是Android开发者和高级用户在进行Android设备维护或开发时不可或缺的工具之一。"
知识点详细说明:
1. Fastboot工具定义:
Fastboot是一种与Android设备进行交互的命令行工具,通常在设备的bootloader模式下使用,这个模式允许用户直接通过USB向设备传输镜像文件以及其他重要的设备分区信息。它支持多种操作,如刷写分区、读取设备信息、擦除分区等。
2. 使用环境:
Fastboot工具原本是Google为Android Open Source Project(AOSP)提供的一个组成部分,因此它通常在Linux或Mac环境下更为原生。但由于Windows系统的普及性,许多开发者和用户需要在Windows环境下操作,因此存在专门为Windows系统定制的Fastboot版本。
3. Fastboot工具的获取与安装:
用户可以通过下载Android SDK平台工具(Platform-Tools)的方式获取Fastboot工具,这是Google官方提供的一个包含了Fastboot、ADB(Android Debug Bridge)等多种工具的集合包。安装时只需要解压到任意目录下,然后将该目录添加到系统环境变量Path中,便可以在任何位置使用Fastboot命令。
4. Fastboot的使用:
要使用Fastboot工具,用户首先需要确保设备已经进入bootloader模式。进入该模式的方法因设备而异,通常是通过组合特定的按键或者使用特定的命令来实现。之后,用户通过运行命令提示符或PowerShell来输入Fastboot命令与设备进行交互。常见的命令包括:
- fastboot devices:列出连接的设备。
- fastboot flash [partition] [filename]:将文件刷写到指定分区。
- fastboot getvar [variable]:获取指定变量的值。
- fastboot reboot:重启设备。
- fastboot unlock:解锁bootloader,使得设备能够刷写非官方ROM。
5. Fastboot工具的应用场景:
- 设备的系统更新或刷机。
- 刷入自定义恢复(如TWRP)。
- 在开发阶段对设备进行调试。
- 解锁设备的bootloader,以获取更多的自定义权限。
- 修复设备,例如清除用户数据分区或刷写新的boot分区。
- 加入特定的内核或修改系统分区。
6. 注意事项:
在使用Fastboot工具时需要格外小心,错误的操作可能会导致设备变砖或丢失重要数据。务必保证操作前已备份重要数据,并确保下载和刷入的固件是针对相应设备的正确版本。此外,不同的设备可能需要特定的驱动程序支持,因此在使用Fastboot之前还需要安装相应的USB驱动。
7. 压缩包文件说明:
资源中提到的"windows-fastboot.zip"是一个压缩文件,解压后应当包含一个或多个可执行文件、库文件等,这些文件合起来组成了Fastboot工具的Windows版本。解压后,用户应当参考解压后的文档或说明文件来进行安装和配置,以便能够正确地使用该工具。
总结而言,Fastboot作为一个功能强大的工具,它为Android设备的开发者和高级用户提供了一个界面简洁但功能全面的操作平台,以实现对设备深层次的控制。然而,它的使用要求用户具备一定的技术知识和经验,否则不当操作可能导致设备损坏。因此,使用Fastboot时应当谨慎并确保自己了解每个命令的具体含义和后果。

管理建模和仿真的文件
管理Boualem Benatallah引用此版本:布阿利姆·贝纳塔拉。管理建模和仿真。约瑟夫-傅立叶大学-格勒诺布尔第一大学,1996年。法语。NNT:电话:00345357HAL ID:电话:00345357https://theses.hal.science/tel-003453572008年12月9日提交HAL是一个多学科的开放存取档案馆,用于存放和传播科学研究论文,无论它们是否被公开。论文可以来自法国或国外的教学和研究机构,也可以来自公共或私人研究中心。L’archive ouverte pluridisciplinaire
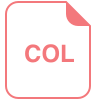
DLMS规约深度剖析:从基础到电力通信标准的全面掌握
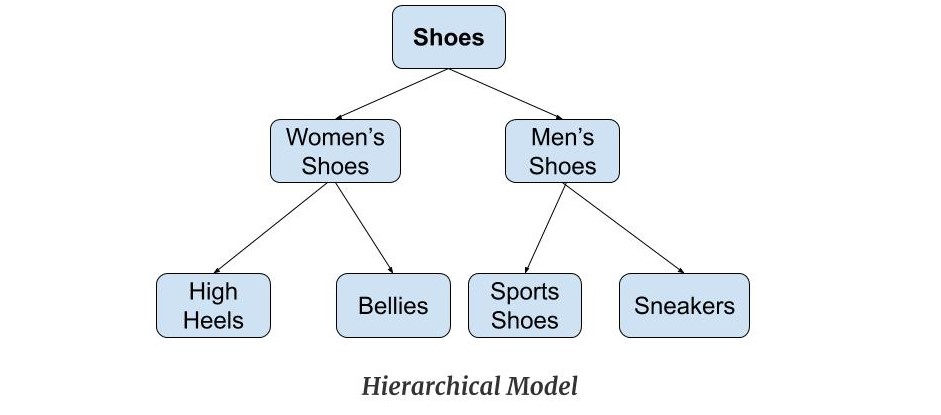
# 摘要
DLMS/COSEM是一种广泛应用于智能电网和电力计量领域的通信协议。本文首先介绍了DLMS规约的基础概念、起源以及核心技术原理,包括协议架构、数据模型、通信过程、数据封装与传输机制。随后,文章探讨了DLMS规约在电力通信中的实际应用,如智能电表和电网自动化系统的数据通信,并分析了DLMS规约的测试与验证方法。文

修改代码,使其正确运行
要使提供的代码能够正确运行,需要解决以下几个关键点:
1. **输入处理**:确保从控制台读取的文法规则和待解析字符串格式正确。
2. **FIRST集和FOLLOW集计算**:确保FIRST集和FOLLOW集的计算逻辑正确无误。
3. **预测分析表构建**:确保预测分析表的构建逻辑正确,并且能够处理所有可能的情况。
4. **LL(1)分析器**:确保LL(1)分析器能够正确解析输入字符串并输出解析过程。
以下是经过修改后的完整代码:
```java
package com.example.demo10;
import java.util.*;
public class Main
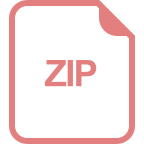
Python机器学习基础入门与项目实践
资源摘要信息:"机器学习概述与Python在机器学习中的应用"
机器学习是人工智能的一个分支,它让计算机能够通过大量的数据学习来自动寻找规律,并据此进行预测或决策。机器学习的核心是建立一个能够从数据中学习的模型,该模型能够在未知数据上做出准确预测。这一过程通常涉及到数据的预处理、特征选择、模型训练、验证、测试和部署。
机器学习方法主要可以分为监督学习、无监督学习、半监督学习和强化学习。
监督学习涉及标记好的训练数据,其目的是让模型学会从输入到输出的映射。在这个过程中,模型学习根据输入数据推断出正确的输出值。常见的监督学习算法包括线性回归、逻辑回归、支持向量机(SVM)、决策树、随机森林和神经网络等。
无监督学习则是处理未标记的数据,其目的是探索数据中的结构。无监督学习算法试图找到数据中的隐藏模式或内在结构。常见的无监督学习算法包括聚类、主成分分析(PCA)、关联规则学习等。
半监督学习和强化学习则是介于监督学习和无监督学习之间的方法。半监督学习使用大量未标记的数据和少量标记数据进行学习,而强化学习则是通过与环境的交互来学习如何做出决策。
Python作为一门高级编程语言,在机器学习领域中扮演了非常重要的角色。Python之所以受到机器学习研究者和从业者的青睐,主要是因为其丰富的库和框架、简洁易读的语法以及强大的社区支持。
在Python的机器学习生态系统中,有几个非常重要的库:
1. NumPy:提供高性能的多维数组对象,以及处理数组的工具。
2. Pandas:一个强大的数据分析和操作工具库,提供DataFrame等数据结构,能够方便地进行数据清洗和预处理。
3. Matplotlib:一个用于创建静态、动态和交互式可视化的库,常用于生成图表和数据可视化。
4. Scikit-learn:一个简单且高效的工具,用于数据挖掘和数据分析,支持多种分类、回归、聚类算法等。
5. TensorFlow:由Google开发的开源机器学习库,适用于大规模的数值计算,尤其擅长于构建和训练深度学习模型。
6. Keras:一个高层神经网络API,能够使用TensorFlow、CNTK或Theano作为其后端进行计算。
机器学习的典型工作流程包括数据收集、数据预处理、特征工程、模型选择、训练、评估和部署。在这一流程中,Python可以贯穿始终,从数据采集到模型部署,Python都能提供强大的支持。
由于机器学习的复杂性,一个成功的机器学习项目往往需要跨学科的知识,包括统计学、数学、计算机科学、数据分析等领域。因此,掌握Python及其相关库的使用只是机器学习工作的一部分,还需要有扎实的理论基础和实践经验。
总结来说,机器学习是一个涉及数据挖掘、统计分析、算法优化等多个领域的综合性科学。Python由于其简洁的语法、丰富的库支持和强大的社区力量,成为了进行机器学习研究和应用开发的首选语言。随着技术的不断进步和算法的持续优化,机器学习的应用领域也在不断扩大,从早期的搜索引擎、推荐系统到现代的自动驾驶、医疗诊断等领域都有着广泛的应用。

"互动学习:行动中的多样性与论文攻读经历"
多样性她- 事实上SCI NCES你的时间表ECOLEDO C Tora SC和NCESPOUR l’Ingén学习互动,互动学习以行动为中心的强化学习学会互动,互动学习,以行动为中心的强化学习计算机科学博士论文于2021年9月28日在Villeneuve d'Asq公开支持马修·瑟林评审团主席法布里斯·勒菲弗尔阿维尼翁大学教授论文指导奥利维尔·皮耶昆谷歌研究教授:智囊团论文联合主任菲利普·普雷教授,大学。里尔/CRISTAL/因里亚报告员奥利维耶·西格德索邦大学报告员卢多维奇·德诺耶教授,Facebook /索邦大学审查员越南圣迈IMT Atlantic高级讲师邀请弗洛里安·斯特鲁布博士,Deepmind对于那些及时看到自己错误的人...3谢谢你首先,我要感谢我的两位博士生导师Olivier和Philippe。奥利维尔,"站在巨人的肩膀上"这句话对你来说完全有意义了。从科学上讲,你知道在这篇论文的(许多)错误中,你是我可以依
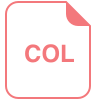
【Shell脚本进阶】:wc命令行数统计的高级用法及解决方案

# 摘要
本文首先介绍wc命令的基本使用和功能,旨在为读者提供一个理解文本行、单词、字节统计的基础。随后,深入探讨了wc命令的高级用法,包括对多个文件的统计与比较,与其他工具如grep和awk的结合使用,以及通过管道实现的高效数据处理。文章第三部分关注wc命令的性能优化和实用技巧,包括提升效率的执行方法和解决常见使用问题的策略。最后,文章探索了wc命令的替代工具与功

python编写一个程序,使得根据输入的起点和终点坐标值计算出坐标方位角
在Python中,我们可以使用Haversine公式来计算两个经纬度之间的方位角。首先,你需要安装`geopy`库,它提供了一个方便的方式来处理地理位置相关的操作。如果你还没有安装,可以使用`pip install geopy`命令。
下面是一个简单的示例程序,用于计算两个点之间的方位角:
```python
from math import radians, cos, sin, asin, sqrt
from geopy.distance import distance
def calculate_bearing(start_point, end_point):
# 将坐标转换
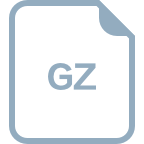
Achilles-2 原始压缩包内容解密
资源摘要信息:"achilles_2.orig.tar.gz"
该压缩包文件名为"achilles_2.orig.tar.gz",它遵循传统的命名规则,其中"orig"通常表示原始版本的软件包或文件。在这个上下文中,"achilles_2"很可能是指软件包的名称,而数字"2"则表示版本号。压缩文件扩展名".tar.gz"表明这是一个使用gzip进行压缩的tar归档文件。Tar是Unix和类Unix系统中用于将多个文件打包成一个文件的工具,而gzip是一种广泛用于压缩文件的工具,它使用了Lempel-Ziv编码(LZ77)算法以及32位CRC校验。
在IT领域,处理此类文件需要掌握几个关键知识点:
1. 版本控制与软件包命名:在软件开发和分发过程中,版本号用于标识软件的不同版本。这有助于用户和开发者追踪软件的更新、新功能、修复和安全补丁。常见的版本控制方式包括语义化版本控制(Semantic Versioning),它包括主版本号、次版本号和补丁号。
2. Tar归档工具:Tar是一个历史悠久的打包工具,它可以创建一个新的归档文件,或者将多个文件和目录添加到一个已存在的归档中。它可以与压缩工具(如gzip、bzip2等)一起使用来减小生成文件的大小,从而更方便地进行存储和传输。
3. Gzip压缩工具:Gzip是一种流行的文件压缩程序,它基于GNU项目,可以有效减小文件大小,常用于Unix和类Unix系统的文件压缩。Gzip通常用于压缩文本文件,但也能处理二进制文件和其他类型的文件。Gzip使用了LZ77算法以及用于减少冗余的哈夫曼编码,来达到较高的压缩比。
4. 文件格式与扩展名:文件扩展名通常用于标识文件类型,以便操作系统和用户可以识别文件的用途。在处理文件时,了解常见的文件扩展名(如.tar、.gz、.tar.gz等)是非常重要的,因为它们可以帮助用户识别如何使用和操作这些文件。
5. 文件传输和分发:压缩包是互联网上常见的文件传输形式之一。开发者和维护者使用压缩包来发布软件源代码或二进制文件,以便用户下载和安装。在处理下载的文件时,用户通常需要根据文件扩展名来解压和解包文件,以便能够访问或运行包内的文件。
总结来说,"achilles_2.orig.tar.gz"是一个包含了名为"achilles"的软件包的第二个版本的源代码压缩文件。要使用这个文件,用户需要使用支持.tar.gz格式的解压工具(如Linux系统中的tar命令或者图形用户界面工具如WinRAR),来解压和查看其中的内容。这样的处理过程涉及到软件版本管理、文件打包、压缩和解压等多个IT知识领域。

关系数据表示学习
关系数据卢多维奇·多斯桑托斯引用此版本:卢多维奇·多斯桑托斯。关系数据的表示学习机器学习[cs.LG]。皮埃尔和玛丽·居里大学-巴黎第六大学,2017年。英语。NNT:2017PA066480。电话:01803188HAL ID:电话:01803188https://theses.hal.science/tel-01803188提交日期:2018年HAL是一个多学科的开放存取档案馆,用于存放和传播科学研究论文,无论它们是否被公开。论文可以来自法国或国外的教学和研究机构,也可以来自公共或私人研究中心。L’archive ouverte pluridisciplinaireUNIVERSITY PIERRE和 MARIE CURIE计算机科学、电信和电子学博士学院(巴黎)巴黎6号计算机科学实验室D八角形T HESIS关系数据表示学习作者:Ludovic DOS SAntos主管:Patrick GALLINARI联合主管:本杰明·P·伊沃瓦斯基为满足计算机科学博士学位的要求而提交的论文评审团成员:先生蒂埃里·A·退休记者先生尤尼斯·B·恩