用vhdl写FIFO 并调用FIFO IP核
时间: 2024-04-14 21:27:11 浏览: 19
下面是一个使用VHDL编写FIFO并调用FIFO IP核的示例:
首先,我们需要编写FIFO的VHDL代码。以下是一个简单的FIFO示例:
```vhdl
library ieee;
use ieee.std_logic_1164.all;
use ieee.numeric_std.all;
entity fifo is
generic (
DATA_WIDTH : integer := 8;
DEPTH : integer := 16
);
port (
clk : in std_logic;
rst : in std_logic;
wr_en : in std_logic;
rd_en : in std_logic;
din : in std_logic_vector(DATA_WIDTH-1 downto 0);
dout : out std_logic_vector(DATA_WIDTH-1 downto 0);
full : out std_logic;
empty : out std_logic
);
end entity fifo;
architecture behavioral of fifo is
type fifo_mem is array (0 to DEPTH-1) of std_logic_vector(DATA_WIDTH-1 downto 0);
signal mem : fifo_mem;
signal wr_ptr : integer range 0 to DEPTH-1 := 0;
signal rd_ptr : integer range 0 to DEPTH-1 := 0;
signal count : integer range 0 to DEPTH := 0;
begin
process(clk, rst)
begin
if rst = '1' then
mem <= (others => (others => '0'));
wr_ptr <= 0;
rd_ptr <= 0;
count <= 0;
elsif rising_edge(clk) then
if wr_en = '1' then
mem(wr_ptr) <= din;
wr_ptr <= wr_ptr + 1;
count <= count + 1;
end if;
if rd_en = '1' then
dout <= mem(rd_ptr);
rd_ptr <= rd_ptr + 1;
count <= count - 1;
end if;
end if;
end process;
full <= '1' when count = DEPTH else '0';
empty <= '1' when count = 0 else '0';
end architecture behavioral;
```
接下来,我们将FIFO IP核实例化到我们的设计中。以下是一个示例代码,展示了如何实例化FIFO IP核并连接到上面的FIFO模块:
```vhdl
library ieee;
use ieee.std_logic_1164.all;
use ieee.numeric_std.all;
entity top_design is
port (
clk : in std_logic;
rst : in std_logic;
wr_en : in std_logic;
rd_en : in std_logic;
din : in std_logic_vector(7 downto 0);
dout : out std_logic_vector(7 downto 0);
full : out std_logic;
empty : out std_logic
);
end entity top_design;
architecture behavioral of top_design is
component fifo is
generic (
DATA_WIDTH : integer := 8;
DEPTH : integer := 16
);
port (
clk : in std_logic;
rst : in std_logic;
wr_en : in std_logic;
rd_en : in std_logic;
din : in std_logic_vector(DATA_WIDTH-1 downto 0);
dout : out std_logic_vector(DATA_WIDTH-1 downto 0);
full : out std_logic;
empty : out std_logic
);
end component fifo;
signal fifo_din : std_logic_vector(7 downto 0);
signal fifo_dout : std_logic_vector(7 downto 0);
signal fifo_full : std_logic;
signal fifo_empty : std_logic;
begin
fifo_inst : fifo
generic map (
DATA_WIDTH => 8,
DEPTH => 16
)
port map (
clk => clk,
rst => rst,
wr_en => wr_en,
rd_en => rd_en,
din => fifo_din,
dout => fifo_dout,
full => fifo_full,
empty => fifo_empty
);
-- 连接FIFO IP核的输入和输出接口到其他信号或模块
-- 例如,将FIFO IP核的输入接口连接到顶层设计的输入信号
fifo_din <= din;
-- 将FIFO IP核的输出接口连接到顶层设计的输出信号
dout <= fifo_dout;
-- 将FIFO IP核的状态信号连接到顶层设计的输出信号
full <= fifo_full;
empty <= fifo_empty;
end architecture behavioral;
```
上述示例中,我们通过实例化`fifo`模块,并将FIFO IP核的输入和输出接口与顶层设计的信号连接起来。这样,我们就可以在顶层设计中使用FIFO IP核了。
请注意,具体的实例化过程可能因为使用的工具和FPGA器件而有所不同。确保参考相关文档和用户手册来了解如何正确实例化和调用FIFO IP核。
相关推荐
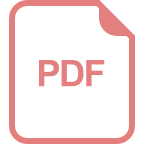









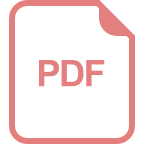