python np.ndarray转换为np.float
时间: 2023-11-20 21:14:31 浏览: 93
要将一个 `np.ndarray` 转换为 `np.float` 类型,你可以使用 `astype()` 方法。下面是一个示例代码:
```python
import numpy as np
array = np.array([1, 2, 3, 4, 5]) # 示例数组
float_array = array.astype(np.float) # 将数组转换为 np.float 类型
print(float_array)
```
输出:
```
[1. 2. 3. 4. 5.]
```
在上面的代码中,我们使用了 `astype()` 方法,将原始数组 `array` 转换为 `np.float` 类型的数组 `float_array`。
相关问题
下面的代码是交叉熵损失函数,这个类中定义两种方法,value求其函数值,derivative求其导数,给出完整代码 class CrossEntropy(Loss): ''' Cross entropy loss function ''' def value(self, yhat: np.ndarray, y: np.ndarray) -> float: #### write your code below #### return def derivative(self, yhat: np.ndarray, y: np.ndarray) -> np.ndarray: #### write your code below #### return
下面是包含 `value()` 和 `derivative()` 方法的完整代码:
```python
import numpy as np
class CrossEntropy(Loss):
'''
Cross entropy loss function
'''
def value(self, yhat: np.ndarray, y: np.ndarray) -> float:
# 计算交叉熵损失函数的值
loss = -np.mean(y * np.log(yhat + 1e-9))
return loss
def derivative(self, yhat: np.ndarray, y: np.ndarray) -> np.ndarray:
# 计算交叉熵损失函数的导数
derivative = (yhat - y) / (yhat * (1 - yhat) + 1e-9)
return derivative
```
在上述代码中,我们定义了一个名为 `CrossEntropy` 的类,它继承了一个名为 `Loss` 的基类。这个类中包含了 `value()` 和 `derivative()` 两个方法。
在 `value()` 方法中,我们使用交叉熵的公式计算损失函数的值。我们使用 `-np.mean(y * np.log(yhat + 1e-9))` 来计算交叉熵,其中 `y` 是真实标签,`yhat` 是预测值。我们添加了一个小的常数 `1e-9` 来避免取对数时出现无穷大的情况。
在 `derivative()` 方法中,我们使用交叉熵损失函数的导数公式 `(yhat - y) / (yhat * (1 - yhat) + 1e-9)` 来计算导数。其中 `y` 是真实标签,`yhat` 是预测值。
你可以根据你的需求使用这个 `CrossEntropy` 类来计算交叉熵损失函数的值和导数。
python numpy.ndarray 转list
可以使用tolist()函数将numpy.ndarray转换为list。
是的,可以使用 `tolist()` 函数将 NumPy 数组转换为 Python 列表。例如,如果 `arr` 是一个 NumPy 数组,你可以使用以下代码将其转换为列表:
```python
import numpy as np
arr = np.array([1, 2, 3])
lst = arr.tolist()
```
现在,`lst` 是一个 Python 列表,它包含与 `arr` 相同的元素。当您创建一个NumPy数组时,您可以将其视为具有以下属性的列表:
1. 数组的维数或轴数,也称为rank。
2. 数组的形状,即每个轴的长度。
3. 数组的数据类型,例如整数、浮点数或布尔值。
此外,NumPy数组还具有许多有用的属性和方法,包括:
1. ndarray.ndim:数组的轴数或维数(rank)。
2. ndarray.shape:数组的形状,以整数元组的形式返回,例如(3,4)表示3行4列的矩阵。
3. ndarray.size:数组中元素的总数。
4. ndarray.dtype:描述数组中元素类型的对象,例如numpy.int32、numpy.int16或numpy.float64等。
5. ndarray.itemsize:数组中每个元素的字节大小。
6. ndarray.data:包含数组实际元素的缓冲区,通常不需要直接访问它。
下面是一个例子:
```
import numpy as np
# 创建一个形状为(2,3)的二维数组
a = np.array([[1,2,3], [4,5,6]])
print("ndim:", a.ndim) # 输出: 2
print("shape:", a.shape) # 输出: (2, 3)
print("size:", a.size) # 输出: 6
print("dtype:", a.dtype) # 输出: int64
print("itemsize:", a.itemsize) # 输出: 8
print("data:", a.data) # 输出: <memory at 0x7fb93883e9a0>
```
相关推荐
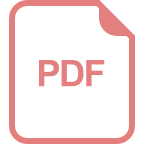
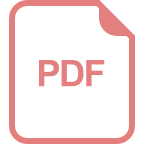
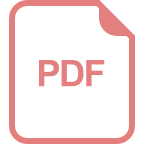












