stm32f103c8t6 串口接收HAL
时间: 2024-12-25 18:20:56 浏览: 4
### STM32F103C8T6 HAL UART Receive Example Code
For the STM32F103C8T6 microcontroller using the Hardware Abstraction Layer (HAL) library to implement UART reception, a typical setup involves initializing the UART peripheral and configuring it for receiving data. Below is an example of how this can be achieved:
#### Initialization Function
The initialization function sets up the UART parameters such as baud rate, word length, stop bits, parity mode, hardware flow control, etc.
```c
#include "stm32f1xx_hal.h"
UART_HandleTypeDef huart1;
void MX_USART1_UART_Init(void)
{
huart1.Instance = USART1;
huart1.Init.BaudRate = 9600;
huart1.Init.WordLength = UART_WORDLENGTH_8B;
huart1.Init.StopBits = UART_STOPBITS_1;
huart1.Init.Parity = UART_PARITY_NONE;
huart1.Init.Mode = UART_MODE_TX_RX;
huart1.Init.HwFlowCtl = UART_HWCONTROL_NONE;
huart1.Init.OverSampling = UART_OVERSAMPLING_16;
if (HAL_UART_Init(&huart1) != HAL_OK)
{
Error_Handler();
}
}
```
This configuration initializes `USART1` with common settings suitable for basic communication tasks[^1].
#### Receiving Data Using Polling Method
To receive data via polling method, one would use the following approach which waits until there's incoming data on the line before proceeding further.
```c
uint8_t receivedData[20]; // Buffer to store received bytes
if(HAL_UART_Receive(&huart1, receivedData, sizeof(receivedData), HAL_MAX_DELAY)== HAL_OK){
// Process Received Data Here...
} else {
// Handle error here...
}
```
In addition to these snippets, comprehensive documentation about the HAL library including detailed descriptions of functions used above like `HAL_UART_Init()` or `HAL_UART_Receive()`, along with their respective parameter lists and return values should also be consulted from official sources provided by STMicroelectronics.
--related questions--
1. How does interrupt-based UART reception differ from polling-based methods?
2. What are some best practices when working with UART interfaces on STM32 devices?
3. Can you provide examples of handling errors during UART transmission/reception operations?
4. Is DMA supported for UART transfers in STM32F1 series? If yes, what advantages does it offer over standard modes?
5. Are there any specific considerations while choosing between different types of UART configurations available within the HAL API?
阅读全文
相关推荐
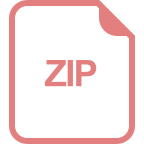
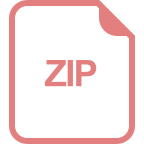
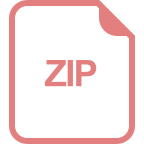






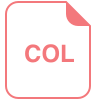








