使用面向对象的方法在canvas中绘制多条运动的龙
时间: 2024-11-22 15:34:52 浏览: 25
在HTML5的Canvas上使用面向对象的方法绘制多条动态的龙,通常涉及以下几个步骤:
1. **创建类**:首先,你可以定义一个`Dragon`类,包含龙的基本属性如位置(x, y)、方向、颜色等,以及绘制身体、头部和尾巴的方法。
```javascript
class Dragon {
constructor(x, y, direction, color) {
this.x = x;
this.y = y;
this.direction = direction;
this.color = color;
this.body = [];
}
// 添加更多方法,如绘制身体、头部和尾巴
draw() {
// 绘制代码
}
move() {
// 更新位置并调整方向
}
}
```
2. **绘制方法**:在`draw`方法中,通过循环绘制龙的身体、头部和尾巴部分,每次移动时更新它们的位置。
```javascript
draw() {
for (let i = 0; i < this.body.length; i++) {
ctx.beginPath();
ctx.moveTo(this.body[i].x, this.body[i].y);
ctx.lineTo(this.x + this.body[i].dx, this.y + this.body[i].dy);
ctx.strokeStyle = this.color;
ctx.stroke();
}
}
```
3. **动画处理**:在`move`方法中,计算每个点的新坐标,并在每次渲染时调用`draw`方法。
```javascript
move() {
// 更新每个点的方向和位置
// ...
requestAnimationFrame(() => this.draw());
}
```
4. **实例化和控制**:最后,在主函数中创建多个`Dragon`实例,并控制它们的行为,比如改变速度、方向或停止移动。
```javascript
function createDragons(num) {
const dragons = [];
for (let i = 0; i < num; i++) {
const dragon = new Dragon(// 初始化位置和方向);
dragons.push(dragon);
}
setInterval(() => {
dragons.forEach(dragon => dragon.move());
}, 1000 / /* 每秒帧数 */);
}
createDragons(3); // 创建3条龙
```
阅读全文
相关推荐








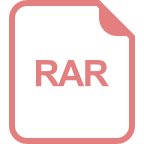










