用C语言 实现单链表初始化 在任意位置插入、 删除 清空 遍历写出完整代码
时间: 2024-09-19 14:17:40 浏览: 62
以下是使用C语言实现单链表的一些基本操作,包括初始化、在任意位置插入、删除、清空以及遍历:
```c
#include <stdio.h>
#include <stdlib.h>
// 定义链表节点结构体
typedef struct Node {
int data;
struct Node* next;
} Node;
// 初始化链表
void initList(Node** head) {
*head = NULL;
}
// 在指定位置插入节点
void insertNodeAtPosition(Node** head, int data, int position) {
Node* newNode = (Node*)malloc(sizeof(Node));
newNode->data = data;
newNode->next = NULL;
if (*head == NULL) {
*head = newNode;
return;
}
Node* current = *head;
for (int i = 0; i < position - 1 && current != NULL; ++i) {
current = current->next;
}
if (current == NULL) {
printf("Insertion position out of range.\n");
return;
}
newNode->next = current->next;
current->next = newNode;
}
// 删除节点
void deleteNode(Node** head, int targetData) {
if (*head == NULL) {
return;
}
if ((*head)->data == targetData) {
Node* temp = *head;
*head = (*head)->next;
free(temp);
return;
}
Node* current = *head;
while (current->next != NULL && current->next->data != targetData) {
current = current->next;
}
if (current->next == NULL) {
printf("Element not found in the list.\n");
return;
}
Node* toRemove = current->next;
current->next = current->next->next;
free(toRemove);
}
// 遍历并打印链表
void traverseList(Node* head) {
if (head == NULL) {
printf("List is empty.\n");
return;
}
Node* current = head;
while (current != NULL) {
printf("%d -> ", current->data);
current = current->next;
}
printf("NULL\n");
}
// 清空链表
void clearList(Node** head) {
Node* current = *head;
Node* temp;
while (current != NULL) {
temp = current;
current = current->next;
free(temp);
}
*head = NULL;
}
int main() {
Node* head = NULL;
// 初始化链表
initList(&head);
// 插入节点示例
insertNodeAtPosition(&head, 5, 0); // 在开始处插入5
insertNodeAtPosition(&head, 10, 2); // 在第二个位置插入10
// 遍历并打印链表
traverseList(head);
// 删除节点示例
deleteNode(&head, 10); // 删除值为10的节点
// 再次遍历并打印链表
traverseList(head);
// 清空链表
clearList(&head);
// 打印链表是否已清空
traverseList(head);
return 0;
}
```
以上代码包含了链表的基本操作。你可以根据需求选择运行特定的部分。注意,这个例子假设了链表中的数据类型是int,并且没有处理特殊边界情况,如在删除元素时找不到目标元素等。在实际应用中,应根据实际情况添加错误检查和异常处理。
阅读全文
相关推荐
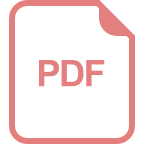
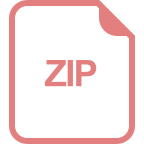
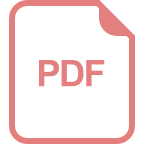
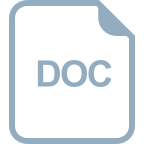
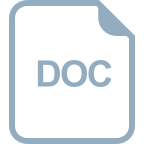
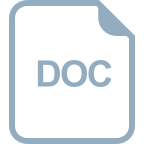
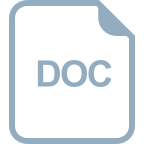
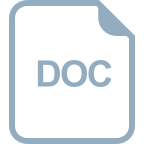
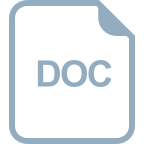
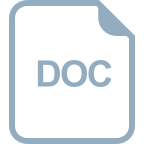
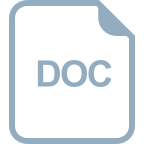
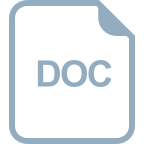
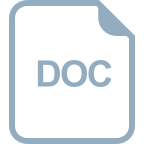
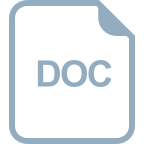


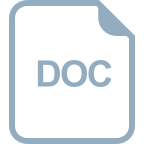

