在.net4.5框架下使用c#下载大文件的webapi接口示例,
时间: 2024-10-22 12:16:27 浏览: 62
在.NET Framework 4.5下,使用C#创建一个Web API接口来下载大文件通常涉及到分块传输和HTTP Range头的处理。以下是一个简单的示例,展示了如何设计一个API来提供这样的功能:
```csharp
using System;
using System.IO;
using System.Net;
using System.Net.Http;
using System.Web.Http;
// 假设我们有一个名为LargeFileService的类,其中包含实际的大文件数据
public class LargeFileService
{
private byte[] _fileData; // 替换成你的大文件数据
public HttpResponseMessage DownloadFile(int chunkSize = 4096)
{
if (_fileData == null)
{
return Request.CreateResponse(HttpStatusCode.NotFound, "File not found.");
}
HttpResponseMessage response = new HttpResponseMessage(HttpStatusCode.OK);
response.Content = new ByteArrayContent(_fileData);
// 设置允许范围请求
response.Headers.Add("Accept-Ranges", "bytes");
response.Content.Headers.ContentType = new MediaTypeHeaderValue("application/octet-stream");
// 处理Range请求
if (Request.Headers.Range != null)
{
long startByte = Convert.ToInt64(Request.Headers.Range.Item1.Start);
long endByte = Convert.ToInt64(Request.Headers.Range.Item1.End);
// 确保请求范围内
if (startByte > endByte || startByte >= _fileData.Length)
{
return Request.CreateResponse(HttpStatusCode.PartialContent,
"Invalid range request.",
new HttpResponseMessageHeaders());
}
using (MemoryStream memoryStream = new MemoryStream())
{
int readLength;
using (var rangeStream = new RangeStream(_fileData, startByte, endByte - startByte + 1))
{
while ((readLength = rangeStream.Read(memoryStream.GetBuffer(), 0, chunkSize)) > 0)
{
memoryStream.SetLength(startByte, memoryStream.Length);
response.Content = new StreamContent(memoryStream);
break;
}
}
}
}
else
{
// 如果没有范围请求,则直接返回整个文件
response.Content.Headers.ContentRange = new ContentRangeHeaderValue(0, _fileData.Length - 1, _fileData.Length);
}
return response;
}
}
// 创建API控制器并映射路由
public class FileDownloadController : ApiController
{
private readonly LargeFileService _largeFileService;
public FileDownloadController(LargeFileService largeFileService)
{
_largeFileService = largeFileService;
}
[HttpGet]
[Route("api/download")]
public HttpResponseMessage Download()
{
return _largeFileService.DownloadFile();
}
}
```
在这个示例中,`Download`方法检查是否支持范围请求,如果是,则根据请求范围返回指定部分的文件内容;如果没有范围请求,则返回整个文件。
阅读全文
相关推荐
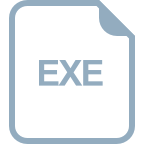
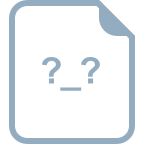







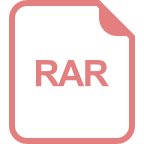
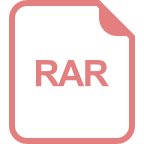
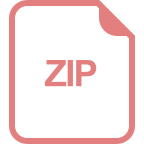
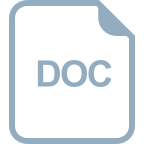
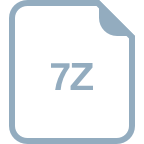
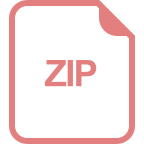
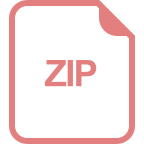
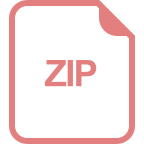
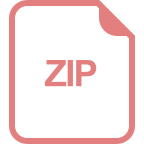