在.net4.5框架下提供一个使用c#下载大文件的webapi接口
时间: 2024-10-22 07:26:31 浏览: 28
在.NET 4.5框架下,你可以创建一个Web API服务来处理大文件的下载。这里是一个简单的示例,展示如何设计一个支持分块下载的API接口:
```csharp
using System;
using System.IO;
using System.Threading.Tasks;
using System.Web.Http;
namespace YourNamespace.Controllers
{
public class DownloadController : ApiController
{
[HttpGet]
public async Task<HttpResponseMessage> DownloadLargeFile(string fileId)
{
if (string.IsNullOrEmpty(fileId))
return Request.CreateResponse(HttpStatusCode.BadRequest, "Invalid file ID");
// 假设你有一个存储大文件的数据库或文件系统
string filePath = GetFilePathFromDatabase(fileId);
if (!System.IO.File.Exists(filePath))
return Request.CreateResponse(HttpStatusCode.NotFound, "File not found");
var contentLength = new FileInfo(filePath).Length;
var response = Request.CreateResponse(HttpStatusCode.OK);
response.Content.Headers.ContentLength = contentLength;
// 使用FileStreamResult或StreamContent进行流式传输
using (var fileStream = File.OpenRead(filePath))
{
response.Content = new StreamContent(fileStream);
response.Content.Headers.ContentType = new MediaTypeHeaderValue("application/octet-stream");
response.Content.Headers.ContentDisposition = new ContentDispositionHeaderValue("attachment")
{
FileName = Path.GetFileName(filePath)
};
// 为了支持断点续传,可以设置Range头来获取部分数据
if (Request.Headers.Range != null)
{
await HandlePartialDownload(fileStream, contentLength, response);
}
else
{
await response.Content.ReadAsStreamAsync();
}
return response;
}
}
private async Task HandlePartialDownload(Stream fileStream, long totalSize, HttpResponseMessage response)
{
// Parse Range header and seek to the appropriate position
byte[] rangeStartBytes = Convert.ToInt64(response.Headers.RequestRange.Start, 10).ToByteArray();
long startByte = BitConverter.ToInt64(rangeStartBytes, 0);
// Check for valid range and adjust end byte accordingly
long endByte;
if (rangeStartBytes.Length == 8 && !response.Headers.RequestRange.HasRangeEnd)
{
endByte = totalSize - 1;
}
else
{
byte[] rangeEndBytes = Convert.ToInt64(response.Headers.RequestRange.End.ToString(), 10).ToByteArray();
endByte = Math.Min(BitConverter.ToInt64(rangeEndBytes, 0), totalSize - 1);
}
// Seek to the starting position and send only requested data
fileStream.Seek(startByte, SeekOrigin.Begin);
response.Content.Headers.ContentRange = new ContentRangeHeaderValue(startByte + 1, endByte, totalSize);
byte[] buffer = new byte[4096];
int bytesRead;
while ((bytesRead = await fileStream.ReadAsync(buffer, 0, buffer.Length)) > 0)
{
await response.Content.WriteAsync(buffer, 0, bytesRead);
}
}
}
}
```
这个`DownloadLargeFile`方法首先验证请求的文件ID,然后读取文件并设置响应头。如果请求包含Range头,它将处理断点续传。注意,这只是一个基本示例,实际生产环境中可能需要考虑错误处理、文件版本控制、并发访问限制等问题。
阅读全文
相关推荐
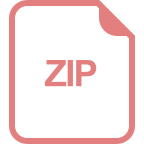
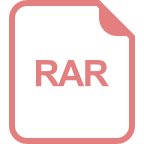
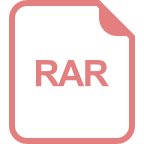









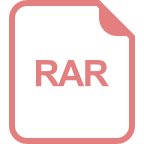
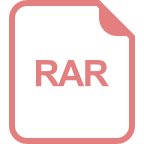
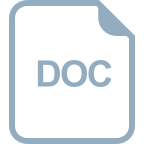
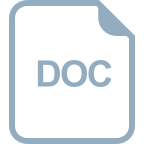
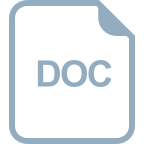
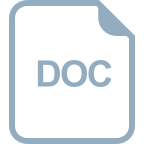